Django Interview Questions
100 Interview Questions for Freshers 1 - 5 years Experienced Candidates
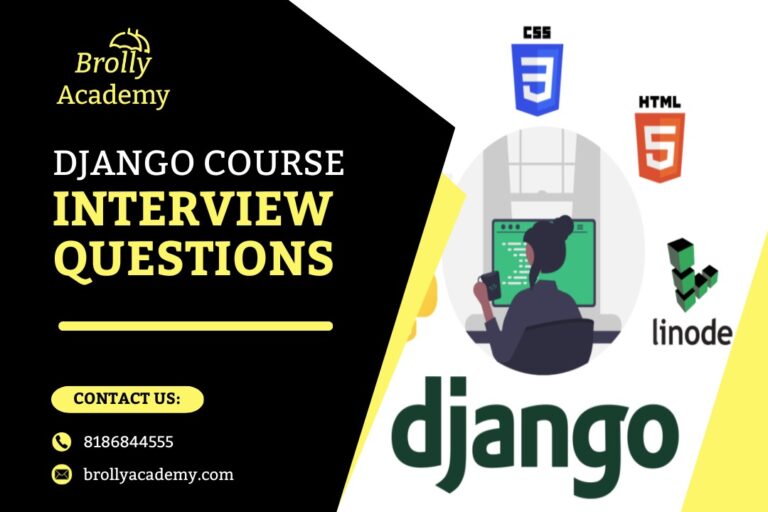
Django: The Python Powered Web Framework That Changed Development
The truth is, Django has revolutionized web development by making Python a formidable language for building robust, scalable web applications. Let’s explore this powerful framework’s journey and key features:
Origins and Evolution
Birth of Innovation (2003) – Django emerged in fall 2003 when web programmers Adrian Holovaty and Simon Willson at the Lawrence Journal-World newspaper began leveraging Python to create applications.
Public Release (2005) – Two years after its creation, Django was released to the public in July 2005 under a BSD license, making it free and open-source for developers worldwide.
Institutional Support (2008) – The Django Software Foundation (DSF), an independent US-based 501(c)(3) non-profit organization, took over Django’s development in June 2008, ensuring its continued growth and maintenance.
What Makes Django Special
High-Level Framework – Django provides abstractions for common web development patterns, allowing developers to build applications faster without reinventing the wheel.
MVT Architecture – Django follows the Model-View-Template architectural pattern, offering a clean separation of concerns that makes code more maintainable and scalable.
Python Foundation – Built on Python, Django inherits the language’s readability, versatility, and robust ecosystem, making it accessible to developers of various skill levels.
Open-Source Philosophy – As a free and open-source framework, Django benefits from community contributions while remaining accessible to everyone from individual developers to large enterprises.
Django continues to evolve with the changing web landscape, proving that frameworks can combine power with accessibility. By making smart architectural choices today, we pave the way for more efficient, secure, and sustainable web development.
Industry Giants Leveraging Django's Power
The truth is, Django has earned its place as the framework of choice for numerous influential organizations across diverse sectors. Here’s who trusts Django with their digital infrastructure:
Instagram – This photo-sharing platform with over a billion users relies on Django to handle its massive scale and complex functionality.
Mozilla – The organization behind Firefox browser employs Django for various web applications and services, validating its security and reliability.
PBS (Public Broadcasting Service) – America’s premier public media organization uses Django to deliver content and manage their digital presence.
The Washington Times – This major news publication leverages Django’s content management capabilities to serve news to millions of readers.
Disqus – The popular commenting platform processes millions of comments daily using Django’s robust architecture.
Bitbucket – This code hosting service by Atlassian depends on Django to manage repositories and developer workflows.
NextDoor – The neighborhood-focused social network uses Django to create community connections while maintaining privacy and security.
Why Industry Leaders Choose Django: Key Advantages
Comprehensive Ecosystem – Django’s rich ecosystem includes thousands of third-party packages that integrate seamlessly, accelerating development while maintaining quality.
Battle-Tested Maturity – With over 15 years of development, Django has evolved through real-world implementation, resulting in a stable, secure, and feature-rich framework trusted by developers worldwide.
Built-in Administrative Interface – Django’s powerful admin dashboard provides instant CRUD (Create, Read, Update, Delete) operations on data models, dramatically reducing development time for back-office functionality.
Extensible Plugin Architecture – The plugin system allows developers to enhance applications with additional features while maintaining full customization capabilities, striking the perfect balance between standardization and flexibility.
Extensive Library Support – Thanks to Django’s large development community, libraries exist for virtually every web development need, from authentication to image processing to API creation.
Sophisticated ORM System – Django’s Object-Relational Mapping allows developers to work with databases using Python objects rather than SQL, increasing productivity while reducing the risk of SQL injection attacks.
By incorporating these advantages into your development workflow, you’re not just building applications—you’re building sustainable digital solutions that can scale and evolve alongside your business requirements.
Top Latest Django interview questions for freshers
1. What is Django?
Django is a web development framework that was developed in a fast-paced newsroom. It is a free and open-source framework that was named after Django Reinhardt who was a jazz guitarist from the 1930s.
Django is maintained by a non-profit organization called the Django Software Foundation. The main goal of Django is to enable Web Development quickly and with ease.
2. Explain Django Architecture?
Django follows the MVT (Model View Template) pattern which is based on the Model View Controller architecture. It’s slightly different from the MVC pattern as it maintains its conventions, so the controller is handled by the framework itself.
The template is a presentation layer. It is an HTML file mixed with Django Template Language (DTL). The developer provides the model, the view, and the template then maps it to a URL, and finally, Django serves it to the user.
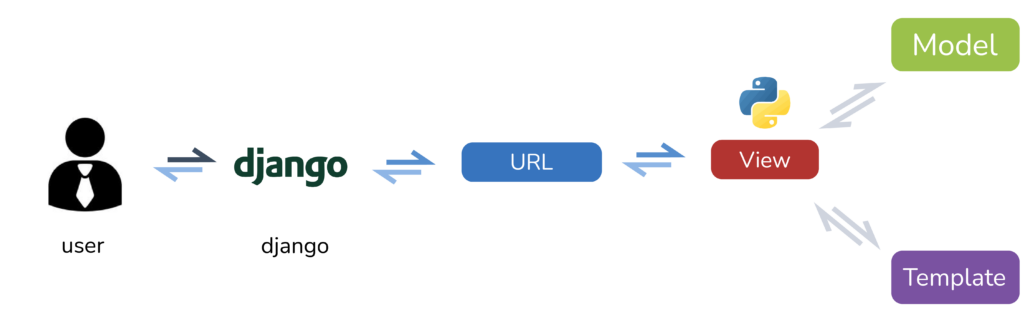
3. Explain the django project directory structure?
- manage.py – A command-line utility that allows you to interact with your Django project
- __init__.py – An empty file that tells Python that the current directory should be considered as a Python package
- settings.py – Comprises the configurations of the current project like DB connections.
- urls.py – All the URLs of the project are present here
- wsgi.py – This is an entry point for your application which is used by the web servers to serve the project you have created.
4. What are models in Django?
A model in Django refers to a class that maps to a database table or database collection. Each attribute of the Django model class represents a database field. They are defined in app/models.py
Example:
from django.db import models
class SampleModel(models.Model):
field1 = models.CharField(max_length = 50)
field2 = models.IntegerField()
class Meta:
db_table = “sample_model”
Every model inherits from django.db.models.Model
Our example has 2 attributes (1 char and 1 integer field), those will be in the table fields.
The metaclass helps you set things like available permissions, singular and plural versions of the name, associated database table name, whether the model is abstract or not, etc.
5. Is Django backend or front end?
Django is suitable for both the backend and frontend. It’s a collection of Python libraries that allow you to develop useful web apps ideal for backend and frontend purposes.
Learn Django From Our Expert Trainer
6. What is the latest version of Django? And explain its features.
The latest version of Django is Django 3.1. The new features of it are:
- Supports asynchronous views and middleware
- Provides JSON field support for all database backends
- Admin layout
- Path lib
- Code Reusability
CDN Integration
- SECURE_REFERRER_POLICY
7. What is the difference between Python and Django?
Both Python and Django are intertwined but not the same. Python is a programming language used for various application developments: machine learning, artificial intelligence, desktop apps, etc.
Django is a Python web framework used for full-stack app development and server development.
Using core Python, you can build an app from scratch or craft the app with Django using prewritten bits of code
8. What architecture does Django use?
Django follows a Model-View-Template (MVT) architecture. It contains three different parts:
- Model: Logical data structure behind the entire app and signified by a database.
- View: It’s a user interface. What you see when you visit a website is called a user interface. Represented by HTML/CSS/Javascript files.
- Template: Deals with the presentation of data.
9. Explain Django's code reusability.
Compared to other frameworks, Django offers more code reusability. As Django is a combination of apps, copying those apps from one directory to another with some tweaks to the settings.py file won’t need much time to write new applications from scratch.
That is why Django is the rapid development framework, and this kind of code reusability is not allowed in any other framework.
10. What are the advantages of using Django?
- Django’s stack is loosely coupled with tight cohesion
- The Django apps make use of very less code
- Allows quick development of websites
- Follows the DRY or the Don’t Repeat Yourself Principle which means, one concept or a piece of data should live in just one place
- Consistent at low as well as high levels
- Behaviors are not implicitly assumed, they are rather explicitly specified
- SQL statements are not executed too many times and are optimized internally
- Can easily drop into raw SQL whenever required
- Flexibility while using URLs
Want to download these Django Interview Questions and Answers in the form of a readable and printable PDF for your interview preparation?
Click the download button below for the PDF version
Learn Django From Our Expert Trainer
Advanced Django experienced technical developers Questions
11. How do you connect your Django project to the database?
Django comes with a default database which is SQLite. To connect your project to this database, use the following commands:
- python manage.py migrate (migrate command looks at the INSTALLED_APPS settings and creates database tables accordingly)
- python manage.py makemigrations (tells Django you have created/ changed your models)
- python manage.py sqlmigrate <name of the app followed by the generated id> (sqlmigrate takes the migration names and returns their SQL)
12. What are ‘Models’?
Models are a single and definitive source for information about your data. It consists of all the essential fields and behaviors of the data you have stored. Often, each model will map to a single specific database table.
In Django, models serve as the abstraction layer that is used for structuring and manipulating your data. Django models are a subclass of the django.db.models.Model class and the attributes in the models represent database fields.
13. What are ‘views’?
Django views serve the purpose of encapsulation. They encapsulate the logic liable for processing a user’s request and for returning
the response to the user. Views in Django either return an HttpResponse or raise an exception such as Http404. HttpResponse contains the objects that consist of the content that is to be rendered to the user.
Views can also be used to perform tasks such as reading records from the database, delegating to the templates, generating a PDF file, etc.
14. What are ‘templates’?
Django’s template layer renders the information to be presented to the user in a designer-friendly format. Using templates, you can generate HTML dynamically.
The HTML consists of both static as well as dynamic parts of the content. You can have any number of templates depending on the requirement of your project. It is also fine to have none of them.
15. What are views in Django?
A view function, or “view” for short, is simply a Python function that takes a web request and returns a web response. This response can be HTML contents of a web page, or a redirect, or a 404 error, or an XML document, or an image, etc.
Example:
from django.http import HttpResponse
def sample_function(request):
return HttpResponse(“Welcome to Django”)
There are two types of views:
- Function-Based Views: In this, we import our view as a function.
- Class-based Views: It’s an object-oriented approach.
11. How do you connect your Django project to the database?
Django comes with a default database which is SQLite. To connect your project to this database, use the following commands:
- python manage.py migrate (migrate command looks at the INSTALLED_APPS settings and creates database tables accordingly)
- python manage.py makemigrations (tells Django you have created/ changed your models)
- python manage.py sqlmigrate <name of the app followed by the generated id> (sqlmigrate takes the migration names and returns their SQL)
What are models in Django?
The truth is, Django models are the cornerstone of any Django application’s data structure. Models serve as the single, definitive source of information about your data, containing all the essential fields and behaviors of the data you’re storing.
Django Models: Core Concepts
Database Mapping – Each Django model class directly maps to a single database table or collection, creating a clean, Pythonic interface to your data.
Attribute-Field Relationship – Every attribute within your model class translates to a corresponding field in the database table, handling data typing and validation automatically.
ORM Integration – Models are the foundation of Django’s Object-Relational Mapping system, eliminating the need to write raw SQL for most database operations.
Inheritance Structure – All Django models inherit from the powerful django.db.models.Model base class, which provides essential methods for data manipulation.
Anatomy of a Django Model
Here’s how a typical Django model is structured:
from django.db import models
class SampleModel(models.Model):
field1 = models.CharField(max_length=50)
field2 = models.IntegerField()
class Meta:
db_table = “sample_model”
In this example:
Class Definition – SampleModel inherits from models.Model, connecting it to Django’s ORM system.
Field Declarations – Two fields are defined:
field1: A character field limited to 50 characters
field2: An integer field for numeric data
Meta Configuration – The nested Meta class provides additional configuration options.
The Power of Meta Options
The Meta class serves as a configuration container for model-level options:
Table Naming – db_table = “sample_model” explicitly sets the database table name, overriding Django’s default naming convention.
Advanced Controls – Beyond table naming, Meta options provide control over:
Permission definitions
Verbose naming (singular and plural)
Abstract model designation
Ordering defaults
Unique constraints
Index configurations
By leveraging Django models effectively, developers can create maintainable, secure database schemas that evolve alongside application requirements, ensuring data integrity while maximizing development productivity.
13. What are views in Django?
Django Views: The Controllers of Web Responses
The truth is, views form the critical processing layer in Django’s architecture, acting as the bridge between data models and templates. A view function is fundamentally a Python callable that accepts a web request and returns an appropriate web response.
Core Functions of Django Views
Request Processing – Views receive HTTP requests from users, processing parameters, form data, and headers to determine appropriate actions.
Business Logic Execution – Within views, application logic determines how to manipulate data, handle user inputs, and prepare information for presentation.
Response Generation – Views generate appropriate HTTP responses that can include:
- Rendered HTML content
- JSON or XML for API endpoints
- File downloads or image data
- Redirect instructions
- Error responses (404, 403, etc.)
Middleware Integration – Views work with Django’s middleware ecosystem for cross-cutting concerns like authentication, caching, and security.
Implementing Basic Views
Here’s a fundamental view implementation:
from django.http import HttpResponse
def sample_function(request):
return HttpResponse("Welcome to Django")
This simple view:
- Receives the request object containing client information
- Returns an HTTP response with plain text content
- Automatically handles proper HTTP headers and status codes
View Implementation Approaches
Django supports two primary paradigms for creating views:
Function-Based Views (FBVs)
- Implemented as standard Python functions
- Direct and explicit control flow
- Excellent for simple, focused use cases
- Lightweight and easy to understand
- Greater flexibility for custom logic
Class-Based Views (CBVs)
- Implemented as Python classes that inherit from View
- Promote code reuse through inheritance and mixins
- Built-in handling for common HTTP methods (GET, POST, etc.)
- Consistent patterns for common web operations
- Simplified implementation of complex behaviors
By making strategic choices about view implementation approaches, developers can create maintainable, extensible web applications that balance simplicity with powerful features. The view layer is where Django’s “don’t repeat yourself” philosophy truly shines, enabling rapid development without sacrificing code quality
What are templates in Django or Django template language?
Django Templates: The Art of Dynamic Web Rendering
Understanding Django Templates
The truth is, Django templates are the cornerstone of transforming static HTML into dynamic, data-driven web experiences. They represent the ‘T’ in Django’s Model-View-Template (MVT) architecture, bridging the gap between raw data and user-facing interfaces.
Core Components of Django Templates
1. Variables: Dynamic Data Insertion
- Enclosed in double curly braces
{{ }}
- Dynamically replaced with actual values during rendering
- Allow seamless integration of backend data into frontend presentation
Example:
<h1>Welcome, {{ username }}!</h1>
2. Tags: Templating Logic Engines
- Enclosed in {% %}
- Provide powerful control structures and functionality
- Enable complex logic without writing Python code directly in templates
Key Tag Types:
- Conditional rendering (
{% if %}
) - Looping (
{% for %}
) - Template inheritance (
{% extends %}
) - Including other templates (
{% include %}
)
Example:
{% if user.is_authenticated %}
<p>Welcome back!</p>
{% else %}
<p>Please log in.</p>
{% endif %}
3. Filters: Data Transformation
- Applied using the pipe symbol
|
- Modify variable display without changing original data
- Provide quick formatting and transformation capabilities
Common Filters:
lower
: Convert text to lowercaseupper
: Convert text to uppercaselength
: Get string or list lengthdate
: Format date objects
Example:
{{ username|capfirst }}
{{ publication_date|date:"F j, Y" }}
4. Comments: Inline Documentation
- Enclosed in
{# #}
- Single-line comments that don’t render in final output
- Useful for developer notes and template documentation
Example:
{# This is a template comment #}
Rendering Process Explained
Context Preparation
- Views generate a context dictionary
- Contains data to be inserted into template
- Bridges backend logic with frontend presentation
Template Rendering
- Variables replaced with actual values
- Tags processed and executed
- Filters applied to modify data presentation
- Static HTML remains unchanged
Best Practices
Separation of Concerns
- Keep complex logic in views
- Use templates primarily for presentation
- Maintain clean, readable template code
Template Inheritance
- Use base templates for consistent layout
- Create modular, reusable template structures
- Reduce code duplication
Performance Considerations
- Minimize complex logic in templates
- Use template fragment caching
- Optimize database queries in views
Django templates transform web development by providing a powerful, flexible system for dynamic content rendering. By understanding these core constructs, developers can create sophisticated, data-driven web applications with remarkable efficiency.
15. What are views in Django?
A view function, or “view” for short, is simply a Python function that takes a web request and returns a web response. This response can be HTML contents of a web page, or a redirect, or a 404 error, or an XML document, or an image, etc.
Example:
from django.http import HttpResponse
def sample_function(request):
return HttpResponse(“Welcome to Django”)
There are two types of views:
- Function-Based Views: In this, we import our view as a function.
- Class-based Views: It’s an object-oriented approach.
Learn Django From Our Expert Trainer
16. What is Django ORM?
Django’s Object-Relational Mapping (ORM): A Pythonic Database Interface
The truth is, Django’s ORM revolutionizes database interactions by providing a powerful abstraction layer that transforms database operations into intuitive Python code.
Key Advantages of Django ORM
Pythonic Database Interaction
- Eliminates the need for writing raw SQL queries
- Allows database operations using Python methods and objects
- Provides a consistent, database-agnostic approach to data manipulation
Comprehensive Database Operations
- Retrieve data with complex filtering
- Save and update records seamlessly
- Delete entries with simple method calls
- Perform advanced queries without SQL syntax
Database Abstraction Benefits
- Supports multiple database backends
- Simplifies database migrations
- Enhances code readability and maintainability
- Reduces potential SQL injection vulnerabilities
Example of ORM Interaction:
# Retrieving records
users = User.objects.filter(active=True)
# Creating a new record
new_user = User.objects.create(
username='django_developer',
email='ravivarmakite@gmail.com'
)
# Updating records
User.objects.filter(id=1).update(is_active=False)
Static Files: Managing Web Assets in Django
Understanding Static Files
Definition
- Additional web assets required by websites
- Includes images, JavaScript, CSS files
- Essential for creating interactive, visually appealing web experiences
Django’s Static Files Management
- Utilizes
django.contrib.staticfiles
app - Provides centralized management of static assets
- Simplifies collection and deployment of web resources
- Utilizes
Static Files Configuration
Settings Configuration
pythonSTATIC_URL = '/static/' STATICFILES_DIRS = [ BASE_DIR / 'static', ] STATIC_ROOT = BASE_DIR / 'staticfiles'
Organizational Structure
bashyour_project/ ├── static/ │ ├── css/ │ ├── js/ │ └── images/
Template Usage
django{% load static %} <link rel="stylesheet" href="{% static 'css/styles.css' %}"> <img src="{% static 'images/logo.png' %}" alt="Logo">
Best Practices
Centralized Asset Management
- Organize static files in dedicated directories
- Use Django’s static template tag for referencing
- Leverage
collectstatic
command for production deployment
Performance Optimization
- Minimize and compress static assets
- Utilize Django’s built-in static file handling
- Consider using content delivery networks (CDNs)
By embracing Django’s ORM and static files management, developers can create more efficient, maintainable, and scalable web applications. These tools abstract complex operations, allowing developers to focus on building innovative features rather than wrestling with low-level implementation details.
17. Define static files and explain their uses?
Websites generally need to serve additional files such as images. Javascript or CSS. In Django, these files are referred to as “static files”, Apart from that Django provides django.contrib.staticfiles to manage these static files.
18. What is Django Rest Framework(DRF)?
Django Rest Framework is an open-source framework based upon Django which lets you create RESTful APIs rapidly.
19. Describe the inheritance styles in Django?
Django offers three inheritance styles:
- Abstract base classes: You use this style when you want the parent class to retain the data you don’t want to type out for every child model.
- Multi-table inheritance: You use this style when you want to use a subclass on an existing model and want each model to have its database table.
- Proxy models: You use this style to modify Python-level behavior with the models without changing the Model’s field.
20. What are Django Models?
A model is a definitive source of information about data, defined in the “app/models.py”.
Models work as an abstraction layer that structures and manipulates data. Django models are a subclass of the “django.db.models”. Model class and the attributes in the models represent database fields.
Want to download these Django Interview Questions and Answers in the form of a readable and printable PDF for your interview preparation?
Click the download button below for the PDF version
Learn Django From Our Expert Trainer
21. Give a brief about the settings.py file.
As the name implies, it’s the main settings file of the Django file.
Everything inside the Django project, like databases, middlewares, backend engines, templating engines, installed applications, static file addresses, main URL configurations, allowed hosts and servers, and security keys stored in this file as a dictionary or list.
So when Django files start, it first executes the settings.py file and then loads the respective databases and engines to quickly serve the request.
22. Is Django a CMS?
No, Django is not a CMS (Content Management System). It’s just a web framework and programming tool that allows you to build websites.
23. What are static files in Django? And how can you set them?
In Django, static files are the files that serve the purpose of additional purposes such as images, CSS, or JavaScript files. Static files managed by “django.contrib.staticfiles”. There are three main things to do to set up static files in Django:
1) Set STATIC_ROOT in settings.py
2) Run manage.py collect static
3) Set up a Static Files entry on the PythonAnywhere web tab
24. What is the use of Middlewares in Django?
Middlewares in Django is a lightweight plugin that processes during request and response execution. It performs functions like security, CSRF protection, session, authentication, etc. Django supports various built-in middlewares.
25. What is the difference between CharField and TextField in Django?
- TextField is a large text field for large-sized text. In Django, TextField is used to store paragraphs and all other text data. The default form widget for this field is TextArea.
- CharField is a string field used for small- to large-sized strings. It is like a string field in C/C++. In Django, CharField is used to store small strings like first name, last name, etc.
Learn Django From Our Expert Trainer
Advanced Django Interview Questions
26. Describe Django Field Class types?
Every field in a model is an instance of the appropriate field class. In Django, field class types determine:
- The column type describes the database about what kind of data to store (e.g., INTEGER, VARCHAR, TEXT).
- The default HTML widget, while rendering a form field
(e.g. <input type=”text”>, <select>)
- The minimal validation requirements used in automatically generated forms and Django admin.
27. What is the usage of "Django-admin.py" and "manage.py"?
- Django-admin.py – It is a command-line utility for administrative tasks.
- manage.py – It is automatically created in each Django project and controls the Django project on the server or even to begin one. It has the following usage:
- Manages the project’s package on the sys. path.
- Sets the DJANGO_SETTINGS_MODULE environment variable
28. What is Jinja templating?
Jinja Templating is a very popular templating engine for Python, the latest version is Jinja2.
Some of its features are:
- Sandbox Execution – This is a sandbox (or a protected) framework for automating the testing process
- HTML Escaping – It provides automatic HTML Escaping as <, >, & characters have special values in templates and if using a regular text, these symbols can lead to XSS Attacks which Jinja deals with automatically.
- Template Inheritance
- Generates HTML templates much faster than default engine
- Easier to debug as compared to the default engine.
29. Explain user authentication in Django?
Django comes with a built-in user authentication system, which handles objects like users, groups, user-permissions, and a few cookie-based user sessions. Django User authentication not only authenticates the user but also authorizes him.
The system consists and operates on these objects:
- Users
- Permissions
- Groups
- Password Hashing System
- Forms Validation
- A pluggable backend system
30. How to configure static files?
Ensure that django.contrib.staticfiles is added to your INSTALLED_APPS
In your settings file. define STATIC_URL for ex.
STATIC_URL = ‘/static/’
In your Django templates, use the static template tag to create the URL for the given relative path using the configured STATICFILES_STORAGE.
{% load static %}
<img src=”{% static ‘my_sample/abcxy.jpg’ %}” alt=”ABC image”>
Store your static files in a folder called static in your app. For example my_sample/static/my_sample/abcxy.jpg
Want to download these Django Interview Questions and Answers in the form of a readable and printable PDF for your interview preparation?
Click the download button below for the PDF version
Learn Django From Our Expert Trainer
31. Explain Django Response lifecycle?
Whenever a request is made to a web page, Django creates an HttpRequest object that contains metadata about the request.
After that Django loads the particular view, passing the HttpRequest as the first argument to the view function. Each view will be returning an HttpResponse object.
On the big picture following steps occur when a request is received by Django:
- First, the Django settings.py file is loaded which also contains various middleware classes (MIDDLEWARES)
- The middlewares are also executed in the order in which they are mentioned in the MIDDLEWARE
- From here on the request is now moved to the URL Router, which simply gets the URL path from the request and tries to map with our given URL paths in the urls.py.
- As soon as it has mapped, it will call the equivalent view function, from where an equivalent response is generated
- The response also passes through the response middleware and sends it back to the client/browser.
32. What databases are supported by Django?
PostgreSQL and MySQL, SQLite, and Oracle. Apart from these, Django also supports databases such as ODBC, Microsoft SQL Server, IBM DB2, SAP SQL Anywhere, and Firebird using third-party packages. Note: Officially Django doesn’t support any no-SQL databases.
33. What's the use of a session framework?
The grouping of activities arranged to accomplish a task together is known as Pipelines. It allows users to manage the individual activities as a single group and provide a quick overview of the activities involved in a complex task with many steps.
ADF activities are grouped into three parts:
- Data Movement Activities – Used to ingest data into Azure or export data from Azure to external data stores.
- Data Transformation Activities – Related to data processing and extracting information from data.
- Control Activities – Specify a condition or affect the progress of the pipeline.
34.What’s the use of Middleware in Django?
Middleware is something that executes between the request and response. In simple words, you can say it acts as a bridge between the request and the response.
Similarly In Django when a request is made it moves through middleware to views and data is passed through middleware as a response.
35. What is the context in Django?
Context is a dictionary mapping template variable name given to Python objects in Django. This is the general name, but you can give any other name of your choice if you want.
Learn Django From Our Expert Trainer
36. What is django.shortcuts.render function?
When a view function returns a webpage as HttpResponse instead of a simple string, we use render(). The render function is a shortcut function that lets the developer easily pass the data dictionary with the template.
This function then combines the template with a data dictionary via a templating engine. Finally, this render() returns as HttpResponse with the rendered text, which is the data returned by models.
Thus, Django render() bypasses most of the developer’s work and lets him use different template engines.
The basic syntax:
render(request, template_name, context=None, content_type=None, status=None, using=None)
The request is the parameter that generates the response. The template name is the HTML template used, whereas the context is a dict of the data passed on the page from the python.
You can also specify the content type, the status of the data you passed, and the render you are returning.
37. What’s the significance of the settings.py file?
As the name suggests this file stores the configurations or settings of our Django project, like database configuration, backend engines, middlewares, installed applications, main URL configurations, static file addresses, templating engines, main URL configurations, security keys, allowed hosts, and much more.
38. How to view all items in the Model?
ModelName.objects.all()
39. How to filter items in the Model?
ModelName.objects.filter(field_name=”term”)
40. What’s the difference between a project and an app in Django?
The app is a module that deals with the dedicated requirements in a project. On the other hand, the project covers an entire app. In Django terms, a project can contain different apps, while an app features in various projects.
Want to download these Django Interview Questions and Answers in the form of a readable and printable PDF for your interview preparation?
Click the download button below for the PDF version
Learn Django From Our Expert Trainer
41. Explain Django URL in brief?
Django allows you to design URL functions however you want. For this, you need to create a Python module informally called URLconf (URL configuration).
This module is purely a Python code and acts as a mapping between URL path expressions and Python functions. Also, this mapping can be as long or short as needed and can also reference other mappings.
The length of this mapping can be as long or short as required and can also reference other mappings. Django also provides a way to translate URLs according to the active language.
42. Explain Django session
Django uses the session to keep track of the state between the site and a particular browser. Django supports anonymous sessions.
The session framework stores and retrieves data on a per-site-visitor basis. It stores the information on the server side and supports sending and receiving cookies. Cookies store the data of the session ID but not the actual data itself.
43. What are Django cookies?
A cookie is a piece of information stored in the client’s browser. To set and fetch cookies, Django provides built-in methods. We use the set_cookie() method for setting a cookie and the get() method for getting the cookie.
You can also use the request.COOKIES[‘key’] array to get cookie values.
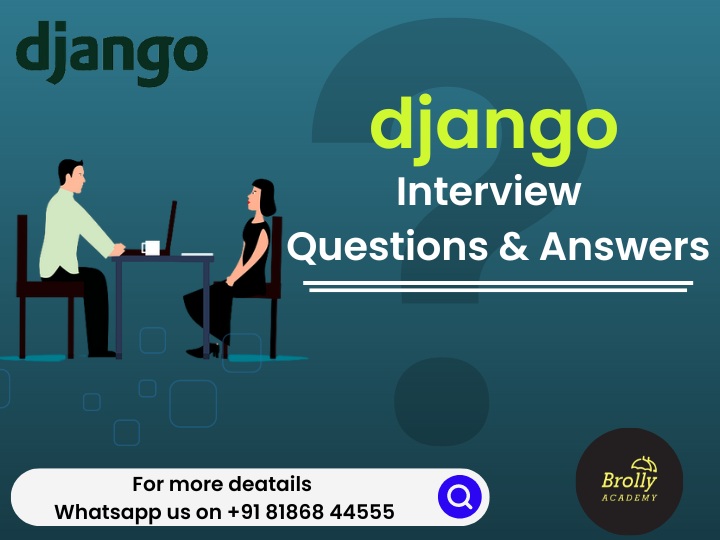
44. Explain the file structure of a typical Django project.
A typical Django project consists of these four files:
- manage.py
- settings.py
- __init__.py
- urls.py
- wsgi.py
The final four files are inside a directory, which is at the same level as manage.py.
- manage.py is the command-line utility of your Django project and controls the Django project on the server.
- settings.py file includes information on all the apps installed in the project.
- The urls.py file acts as a map for the whole web project.
- The __init__.py file is an empty file that makes the python interpreter understand that the directory consisting of settings.py is a module/ package.
- The wsgi.py file is for the server format WSGI
45. Why is Django called a loosely coupled framework?
Django is known as a loosely coupled framework beca+use of its MVT architecture.
Django’s architecture is a variant of MVC architecture, and MVT is beneficial because it completely discards server code from the client’s machine. Models and Views are present on the client machine, and templates only return to the client.
All the architecture components are different from each other. Both frontend and backend developers can work simultaneously on the projects as they won’t affect each other when changed.
Learn Django From Our Expert Trainer
46. What is the Django REST framework (DRF)?
Django REST framework is a flexible and powerful toolkit for building Web APIs rapidly.
The following are the significant reasons that are making REST framework perfect choice:
- Web browsable API
- Authentication policies
- Serialization
- Extensive documentation and excellent community support.
- Perfect for web apps since they have low bandwidth.
- Global companies like Red Hat, Mozilla, Heroku, Eventbrite, etc., trust this framework.
47. Difference between Django OneToOneField and ForeignKey Field?
Both of them are of the most common types of fields used in Django. The only difference between these two is that ForeignKey field consists of an on_delete option along with a model’s class because it’s used for many-to-one relationships while on the other hand, the OneToOneField, only carries out a one-to-one relationship and requires only the model’s class.
48. Explain the Django REST framework.
With the help of Django’s REST framework, you can create RESTful APIs quickly and efficiently.
This framework gets funding from various big shot companies and is popular due to its multiple commendable features such as serialization, authentication policies, etc.
RESTful APIs are well-suited for creating web applications since they use low bandwidth and can communicate over the internet via GET, POST and PUT methods.
49. How to view and filter items from the database?
In order to view all the items from your database, you can make use of the ‘all()’ function in your interactive shell as follows:
- XYZ.objects.all() where XYZ is some class that you have created in your models
To filter out some element from your database, you either use the get() method or the filter method as follows:
- XYZ.objects.filter(pk=1)
- XYZ.objects.get(id=1)
50. Explain how a request is processed in Django?
In case some user requests a page from some Django powered site, the system follows an algorithm that determines which Python code needs to be executed. Here are the steps that sum up the algorithm:
Want to download these Django Interview Questions and Answers in the form of a readable and printable PDF for your interview preparation?
Click the download button below for the PDF version
Learn Django From Our Expert Trainer
51. Is Django too monolithic? Explain this statement.
The Django framework is monolithic, which is valid to some extent. As Django’s architecture is MVT-based, it requires some rules that developers need to follow to execute the appropriate files at the right time.
With Django, you get significant customizations with implementations. Through this, you cannot change file names, variable names, and predefined lists.
Django’s file structure is a logical workflow. Thus the monolithic behavior of Django helps developers to understand the project efficiently.
52. Explain user authentication in Django
Django comes with a built-in user authentication system to handle objects such as users, groups, permissions, etc. It not only performs authentication but authorization as well.
Following are the system objects:
- users
- Groups
- Password Hashing System
- Permissions
- A pluggable backend system
- Forms Validation
Apart from this, there are various third-party web apps that we can use instead of the default system to provide more user authentication with more features.
53. What is the "django.shortcuts.render" function?
When a View function returns a web page as HttpResponse instead of a simple string, we use the render function.
Render is a shortcut for passing a data dictionary with a template. This function uses a templating engine to combine templates with a data dictionary.
Finally, the render() returns the HttpResponse with the rendered text, the models’ data.
Syntax:
render(request, template_name, context=None, content_type=None, status=None, using=None)
The request generates a response.
The template name and other parameters pass the dictionary.
For more control, specify the content type, the data status you passed, and the render you are returning.
54. What is the use of forms in Django?
Forms serve the purpose of receiving user inputs and using that data for logical operations on databases. Django supports form classes to create HTML forms. It defines a form and how it works and appears.
Django’s forms handle the following parts:
- Prepares and restructures data to make it ready for rendering
- Creates HTML forms for the data
- Processes submitted forms and data from the client.
55. Can you explain how to add View functions to the urls.py file?
There are two ways to add the view function to the main URLs config:
- Adding a function View
In this method, import the particular View’s function and add the specific URL to the URL patterns list.
- Adding a Class-based view
This one is a more class-based approach. For this, import the class from the views.py and then add the URL to the URL patterns. An inbuilt method is needed to call the class as a view.
Write the name of the function on the previous method as shown below:
class_name.as_view()
This will pass your view class as a view function.
Both function-based and class-based have their advantages and disadvantages. Depending on the situation, you can use them to get the right results.
Learn Django From Our Expert Trainer
56. Explain Django Security.
Protecting user’s data is an essential part of any website design. Django implements various sufficient protections against several common threats. The following are Django’s security features:
- Cross-site scripting (XSS) protection
- SQL injection protection
- Cross-site request forgery (CSRF) protection
- Enforcing SSL/HTTPS
- Session security
- Clickjacking protection
- Host header validation
57. What is Ajax in Django?
AJAX (Asynchronous JavaScript And XML) allows web pages to update asynchronously to and from the server by exchanging data in Django. That means without reloading a complete webpage you can update parts of the web page.
It involves a combination of a browser built-in XMLHttpRequest object, HTML DOM, and JavaScript.
58. How to handle Ajax requests in Django?
To handle Ajax requests in the Django web framework, perform the following:
- Initialize Project
- Create models
- Create views
- Write URLs
- Carry out requests with Jquery Ajax.
- Register models to admin
59. What are Django generic views?
Writing views is a heavy task. Django offers an easy way to set Views called Generic Views. They are classes but not functions and stored in “django.views.generic”.
Generic views act as a shortcut for common usage patterns. They take some common idioms and patterns in view development and abstract them to write common views of data without repeating yourself quickly.
60. What is the correct way to make a variable available to all your templates?
In case all your templates need the same objects, use “RequestContext.” This method takes HttpRequest as the first parameter and populates the context with a few variables simultaneously as per the engine’s context_processors configuration option.
Want to download these Django Interview Questions and Answers in the form of a readable and printable PDF for your interview preparation?
Click the download button below for the PDF version
Learn Django From Our Expert Trainer
61. How does templating work in Django?
Templates are the reason behind Django’s ability to create dynamic web pages. These templates are HTML code returned as an HTTP response.
Furthermore, Django has a templating engine capable of handling templating. You can use some of the template syntaxes while declaring the variables, control logic, and comments.
Once you provide all the template syntax within the HTML structure, the web page is requested and called by the view function. Later, the Django template engine will get the HTML structure with variables and the data to replace these variables.
The templating engine will replace these while executing the control logic and generating filters. It will then render the required HTML and send it to the browser.
62. What are view functions? Can you directly import a function within the URL?
A view is a middle layer between a model and a template, and it will take the data from the model and pass it to a template.
Every application in Django has a view.py file that stores view functions, and these functions take the argument and return the browser-renderable format.
You can easily import view functions in the URL file. To do so, you need to import the view function in the urls.py file and add the desired path required by the browser to call that function.
63. What is the django.shortcuts.render function?
When the webpage is returned as the HttpResponse instead of the simple string by a view function, use the render() function.
This function will allow the developers to pass the data dictionary using a template. Then this function will use the templating engine for combining the template with the data dictionary.
After that, this function will return the HttpResponse with the rendered text that is being returned by the models. In this way, this function will save a lot of time for developers and allow them to use different templating engines.
The basic render Syntax:
render(request, template_name, context=None, content_type=None, status=None, using=None) |
64. What does the urls-config file contain?
This config file stores all the URL lists and mapping to their respective view functions. These URLs can be mapped to view functions, class-based views, and url-config of another application.
The default URL list name is urlpatterns, and it contains all the path() or re_path() URL patterns. The project URL comes with the root urls.py file, and with every application, we can also make an isolated urls.py file for that application.
Example
#urls.py
65. What popular websites use Django?
Django is a compelling framework and is used by various renowned organizations. Some of the highly trafficked websites using Django are:
- Disqus
- Mozilla
- Bitbucket
- YouTube
- Spotify
- NASA
- Eventbrite
Learn Django From Our Expert Trainer
66. How can you set up the database in Django?
You can edit mysite/setting.py, a module representing Django settings. By default, Django uses SQLite, which is easy to use and does not require any type of installation.
If your database choice is different, you have to do the following keys in the DATABASE ‘default’ item for matching your database connection settings:
- Engines: You can make the changes to the database using ‘django.db.backends.sqlite3’, ‘django.db.backends.mysql’, ‘django.db.backends.postgresql_psycopg2’, ‘django.db.backends.oracle’, and so on.
- Name: If you use SQLite as your database, the database file will be on your computer. The name should be a full absolute path, including the file name of that file.
But, if you do not choose SQLite, you need to add the settings such as Password, Host, User, etc.
67. How can you set up static files in Django?
For setting up the static files in Django, you need to consider the below steps:
- Firstly, set the STATIC_ROOT in the settings.py file
- Run the manage.py collectstatic file
- Finally, set up a Static Files entry on the PythonAnywhere web tab
68. What is the session framework in Django?
Django comes with the session framework helping to store and retrieve the arbitrary data on a per-site-visitor basis. It saves all data on the server-side and abstracts the receiving and sending of cookies. You can implement the session via middleware.
69. What are the specific Django field class types?
Field class types specify the following:
- The database column type.
- The default HTML widget for availing while rendering a form field.
- The minimal validation requirements used in Django admin that helps in automatically generated forms.
70. What is a model in Django?
A Model is specified as a Python class derived from the Model class. This model class is imported from the django.db.models library. The main concept of Django Models is to create objects for storing data from a user in a user-defined format.
The model class is a pre-defined class with lots of benefits. You can define the field with specific attributes as you can do in SQL, but the same can also be achieved in Python.
This class is parsed by Django ORM or backend engine, and there is no need to do anything related to a database, such as creating tables and defining fields afterward mapping the fields with the attribute of the class.
Want to download these Django Interview Questions and Answers in the form of a readable and printable PDF for your interview preparation?
Click the download button below for the PDF version
Learn Django From Our Expert Trainer
71. How can you limit admin access so that the objects can only be edited by those users who have created them?
Django’s ModelAdmin class provides customization hooks using which, you can control the visibility and editability of objects in the admin. To do this, you can use the get_queryset() and has_change_permission().
72. What to do when you don’t see all objects appearing on the admin site?
Inconsistent row counts are a result of missing Foreign Key values or if the Foreign Key field is set to null=False. If the ForeignKey points to a record that does not exist and if that foreign is present in the list_display method, the record will not be shown the admin changelist.
73. Is it mandatory to use the model/ database layer?
No. The model/ database layer is actually decoupled from the rest of the framework.
74. Is Django named after that Quentin Tarantino movie?
No, Django is named after Django Reinhardt, a jazz guitarist from the 1930s to the early 1950s who is considered one of the best guitarists of all time.
75. Why do web developers prefer Django?
Web developers use Django because it:
- Allows code modules to be divided into logical groups, making them flexible to change
- Provides an auto-generated web admin module to ease website administration
- Provides a pre-packaged API for common user tasks
- Enables developers to define a given function’s URL
- Allows users to separate business logic from the HTML
- Is written in Python, one of the most popular programming languages available today
- Gives you a system to define the HTML template for your web page, avoiding code duplication
Learn Django From Our Expert Trainer
76. What is CRUD?
It has nothing to do with dirt or grime. It’s a handy acronym for Create, Read, Update, and Delete. It’s a mnemonic framework used to remind developers on how to construct usable models when building application programming interfaces (APIs).
77. Does Django have any drawbacks?
Django’s disadvantages include:
- Its monolithic size makes it unsuitable for smaller projects
- Everything hinges on Django’s ORM (Object-Relational Mapping)
- Everything must be explicitly defined due to a lack of convention
78. What does Django architecture look like?
Django architecture consists of:
- Models. Describes the database schema and data structure
- Views. Controls what a user sees. The view retrieves data from appropriate models, executes any calculations made, and passes it on to the template
- Templates. Controls how the user sees the pages. It describes how the data received from the views need to be altered or formatted to display on the page
- Controller. Made up of the Django framework and URL parsing
79. How do you check which version of Django that you have installed on your system?
You can check the version by opening the command prompt and entering the command:
Python-m Django–version
80. Does Django support multiple-column primary keys?
No, Django supports only single-column primary keys.
Want to download these Django Interview Questions and Answers in the form of a readable and printable PDF for your interview preparation?
Click the download button below for the PDF version
Learn Django From Our Expert Trainer
81. What is a QuerySet in the context of Django?
QuerySet is a collection of SQL queries. The command print(b.query) shows you the SQL query created from the Django filter call.
82. What do you use django.test.Client class for?
The Client class acts like a dummy web browser, enabling users to test views and interact with Django-powered applications programmatically. This is especially useful when performing integration testing.
83. What is a Meta Class in Django?
A Meta class is simply an inner class that provides metadata about the outer class in Django. It defines such things as available permissions, associated database table name, singular and plural versions of the name, etc.
84. When should we generate and apply migrations in a Django project and why?
We should do that whenever we create or make changes to the models of one of the apps in our project. This is because a migration is used to make changes to the database schema, and it is generated based on our models.
85. What is serialization in Django?
Serialization is the process of converting Django models into other formats such as XML, JSON, etc.
Learn Django From Our Expert Trainer
86. What are generic views?
When building a web application there are certain kinds of views that we build again and again, such as a view that displays all records in the database (e.g., displaying all books in the books table), etc.
These kinds of views perform the same functions and lead to repeated code. To solve this issue, Django uses class-based generic views.
When using generic views, all we have to do is inherit the desired class from Django.views.generic module and provide some information like model, context_object_name, etc.
87.Is Django stable?
Yes, Django is quite stable. Many companies like Disqus, Instagram, Pinterest, and Mozilla have been using Django for many years.
Want to download these Django Interview Questions and Answers in the form of a readable and printable PDF for your interview preparation?
Click the download button below for the PDF version
Django Framework: 15 Essential FAQs for Modern Web Development
Learn Django From Our Expert Trainer
1. What Exactly is Django?
Django is a high-level Python web framework that enables rapid development of secure and maintainable web applications. It follows the model-view-template (MVT) architectural pattern, providing developers with a robust toolkit for building complex web solutions efficiently.
2. Why Choose Django Over Other Web Frameworks?
Key advantages include:
Rapid development capabilities
Robust security features
Scalable architecture
Comprehensive documentation
Built-in administrative interface
Strong community support
DRY (Don’t Repeat Yourself) principle implementation
3. How Does Django’s MVT Architecture Work?
Model Layer
Represents database schema
Defines data structures
Manages database interactions through ORM
View Layer
Handles business logic
Processes user requests
Interacts between models and templates
Template Layer
Manages presentation logic
Renders dynamic content
Separates design from Python code
4. What is Django’s ORM and Why is it Powerful?
Django’s Object-Relational Mapping (ORM) allows developers to:
Interact with databases using Python code
Avoid writing raw SQL queries
Support multiple database backends
Implement complex queries with minimal code
Ensure database abstraction and portability
5. How Does Django Ensure Web Application Security?
Security features include:
Cross-site scripting (XSS) protection
Cross-site request forgery (CSRF) middleware
SQL injection prevention
Clickjacking protection
Secure password hashing
Authentication system
Comprehensive user permission management
6. What are Django Migrations?
Migrations are Django’s way of:
Propagating model changes to database schema
Versioning database structure
Allowing incremental, reproducible database modifications
Providing rollback capabilities
Maintaining database consistency across different environments
7. Can Django Support Asynchronous Programming?
Supports ASGI (Asynchronous Server Gateway Interface)
Enables async views and middleware
Improves performance for I/O-bound operations
Compatible with modern Python async frameworks
8. How Does Django Handle Authentication?
Django provides a robust authentication system that:
Manages user registration
Handles login/logout processes
Implements password reset functionality
Supports custom user models
Integrates with social authentication providers
9. What are Django Signals?
Signals allow decoupled applications to get notified when certain actions occur:
Sender-receiver communication pattern
Trigger actions based on model events
Reduce direct coupling between components
Enable modular event-driven architectures
10. How to Optimize Django Application Performance?
Performance optimization strategies:
Use select_related() and prefetch_related()
Implement database indexing
Utilize caching mechanisms
Minimize database queries
Use database query optimization techniques
11. What are Django REST Framework Capabilities?
Django REST Framework offers:
Powerful API development tools
Serialization capabilities
Authentication policies
Comprehensive documentation generation
Browsable API interfaces
Extensive customization options
12. How Does Django Support Internationalization?
Django provides:
Translation mechanisms
Locale-specific formatting
Language switching capabilities
Timezone handling
Multilingual content management
13. What Deployment Options Exist for Django Applications?
Deployment strategies include:
Heroku
AWS Elastic Beanstalk
DigitalOcean
Docker containerization
Gunicorn with Nginx
Platform-as-a-Service solutions
14. How Active is the Django Community?
The Django community offers:
Regular framework updates
Extensive documentation
Multiple conferences and meetups
Active GitHub repository
Comprehensive package ecosystem
Responsive support channels
15. What’s the Future of Django?
Django continues evolving with:
Enhanced async support
Improved performance
Modern web development integration
Machine learning compatibility
Cloud-native architecture support