Advanced React js Interview Questions and Answers
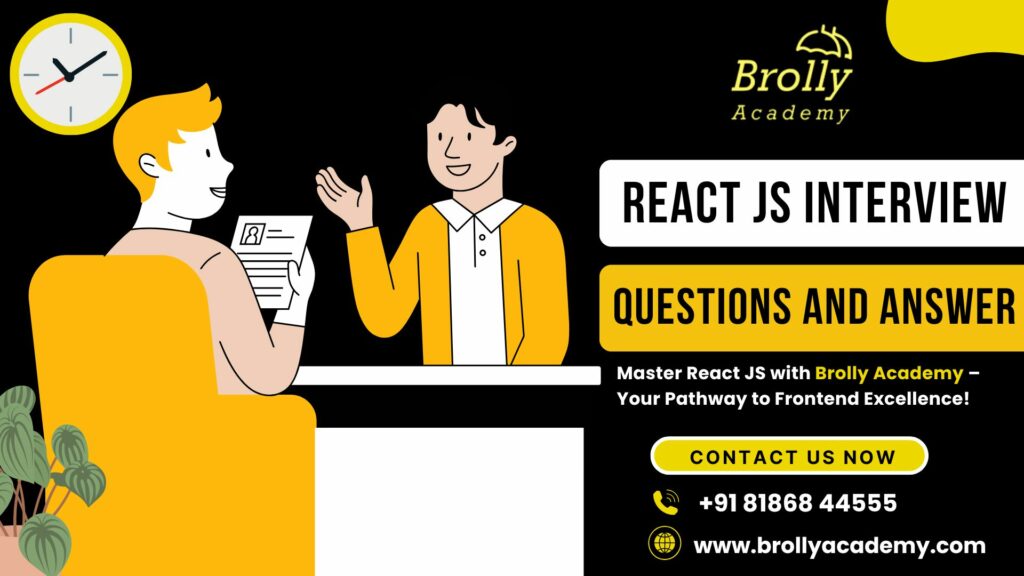
why React is important in web development.
- React is an open-source JavaScript library designed for building fast, scalable, and dynamic web applications.
- It was created by Jordan Walke, a software engineer at Facebook.
- Developers familiar with JavaScript can easily use React to develop interactive user interfaces.
- This collection of React interview questions covers fundamental to advanced topics, including Virtual DOM, Components, State, Props, JSX, Hooks, and Routing.
- Whether you’re a fresher or an experienced professional with 2–10 years of expertise, these questions will help you prepare for your next technical interview with confidence.
- React JS is a powerful JavaScript library used for building fast and scalable user interfaces. Developed by Facebook, it enables developers to build reusable components, manage state efficiently, and create dynamic single-page applications (SPAs).
- React’s virtual DOM ensures optimal performance, making it a preferred choice for front-end development
Learn with Brolly Academy and master React JS with hands on Projects training, expert interview guidance, and real-world projects to accelerate your career!
If you want a PDF of these React JS Interview Questions and Answers, click below to download.
React js Interview Questions and Answers for Freshers
1. What is React?
Answer: React is a JavaScript library developed by Facebook for building user interfaces.
It allows developers to build single-page applications by creating reusable UI components.
React uses a virtual DOM to optimize rendering performance by updating only the necessary parts of the UI.
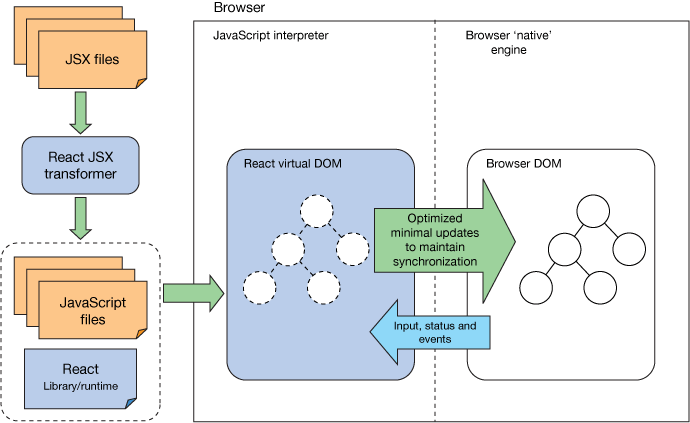
2. What is the virtual DOM?
Answer : The virtual DOM is a lightweight copy of the actual DOM (Document Object Model) in the memory. Changes occurs, React first updates the virtual DOM, and then it compares it with the real DOM to update only the changed elements, which results in improved performance.
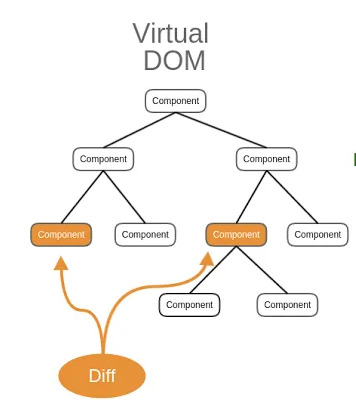
3. What are components in React?
Answer : Components are the building blocks of React applications. They are JavaScript functions or classes that accept inputs (props) and return a React element describing how the UI should appear. Components can be either functional components (stateless) or class components (stateful).
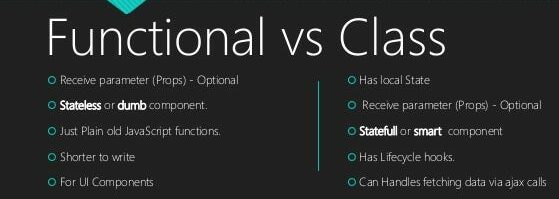
4. What is JSX?
Answer : JSX (JavaScript XML) is a syntax extension for JavaScript that looks similar to HTML. It is used in React to describe what the UI should look like. JSX allows you to write HTML-like code inside JavaScript, which React then converts to JavaScript functions that render elements on the screen.
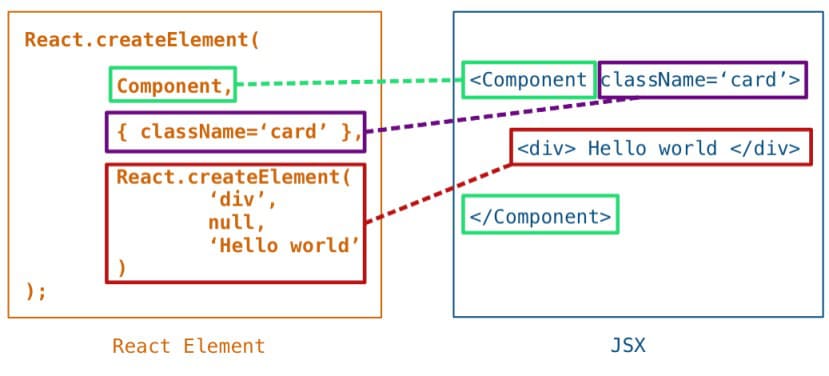
5. What are props in React?
Answer : Props (short for “properties”) are read-only inputs passed from a parent component to a child component. They allow data to be shared between components, and they cannot be modified within the child component. Props are used to render dynamic content in a component.
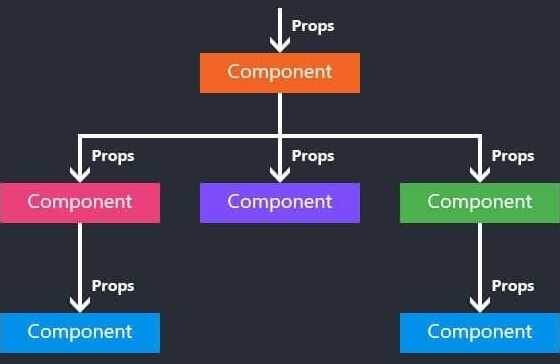
6. What is state in React?
Answer : State is an object used to represent the dynamic data of a component. Unlike props, state is mutable and can be changed within the component. The state triggers a re-render of the component whenever it changes. It is often used for handling user input, form data, or any other dynamic behavior.
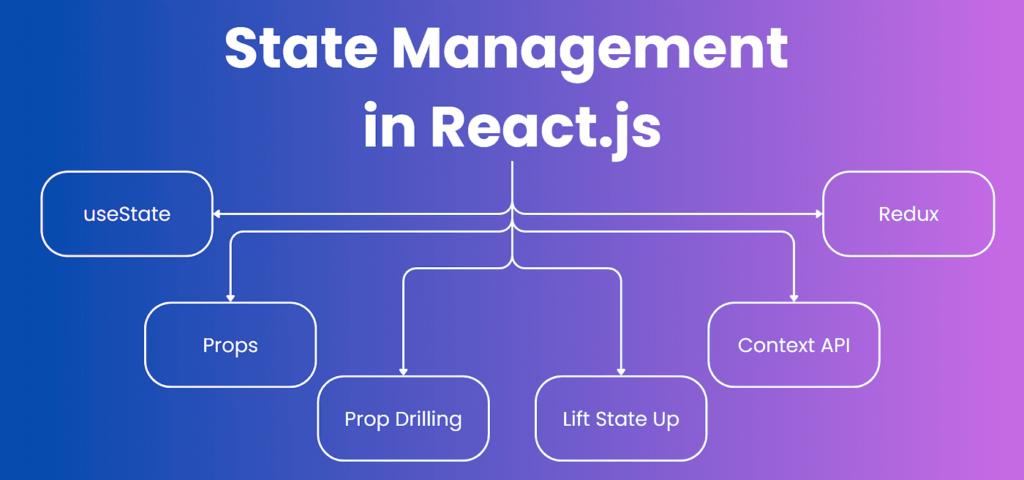
7. What is the difference between props and state?
Answer:
- Props: Passed from parent to child components and are read-only.
- State: Managed within the component and can be changed by the component itself. It represents the dynamic data that changes over time.
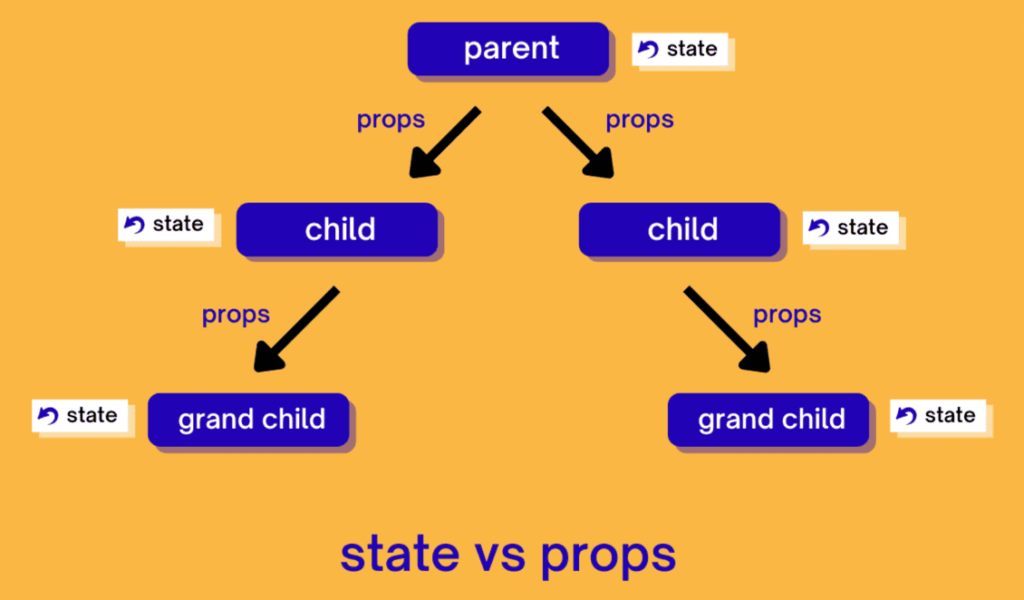
8. What are hooks in React?
Answer:
Hooks are functions that allow functional components to use state and other React features without writing a class. Some commonly used hooks include:
- useState: For adding state to a functional component.
- useEffect: For performing side effects (like fetching data or setting up subscriptions).
- useContext: For accessing the context API.
- useRef: For accessing and manipulating DOM elements.
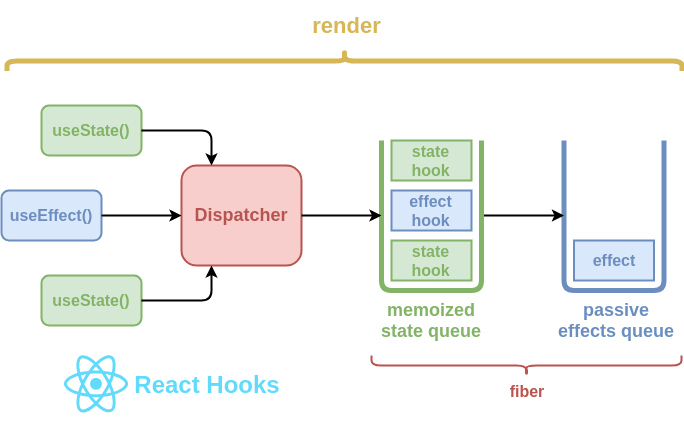
9. What is the useEffect hook?
Answer:
useEffect is a hook that allows you to perform side effects in functional components. It is commonly used for tasks like fetching data, setting up subscriptions, or manipulating the DOM. It takes a function as an argument, which is executed after every render or when specific dependencies change.
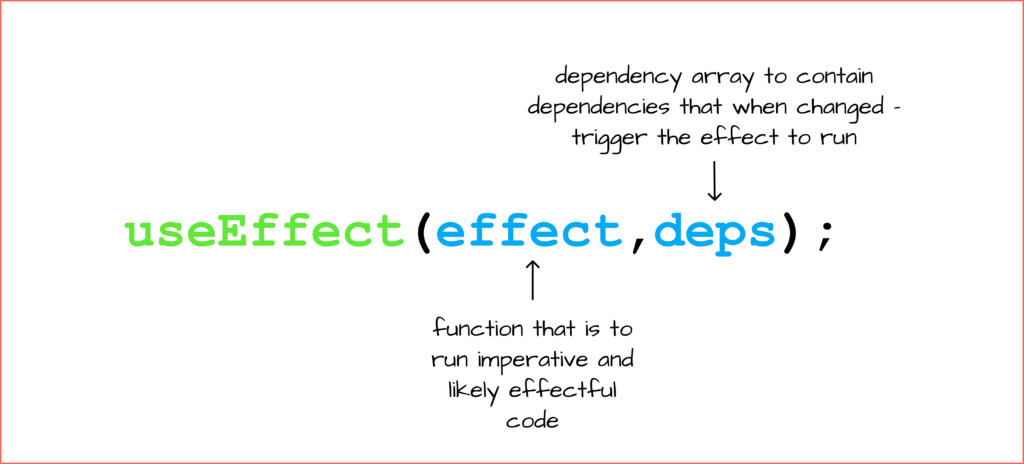
10. What is a component lifecycle in React?
Answer:
Component lifecycle refers to the different stages a component goes through during its existence, including:
- Mounting: When the component is being created and inserted into the DOM.
- Updating: When the component’s state or props change.
Unmounting: When the component is being removed from the DOM. Lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount are used to manage side effects in class components. With functional components, these can be handled using the useEffect hook.
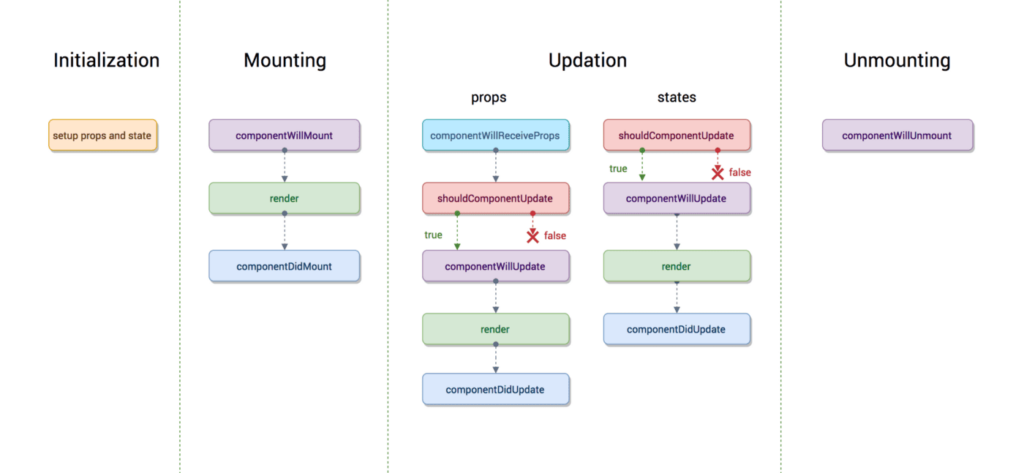
11. What is Redux?
Answer:
Redux is a state management library used with React to manage the application state in a centralized store. It provides a predictable way to manage the state of an entire application, making it easier to share state across components. Redux follows a unidirectional data flow and consists of actions, reducers, and a store.
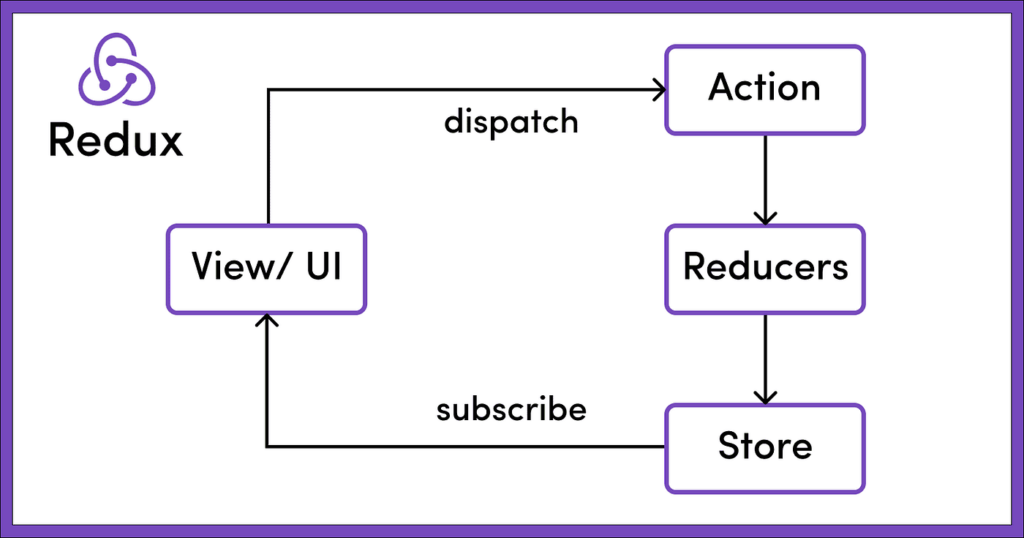
12. What is the difference between React and Angular?
Answer:
- React is a JavaScript library focused on building user interfaces with components. It uses a virtual DOM and can be combined with other libraries for state management (like Redux).
- Angular is a full-fledged MVC framework that provides a complete solution for building web applications. It includes built-in tools for routing, form handling, HTTP requests, and more.
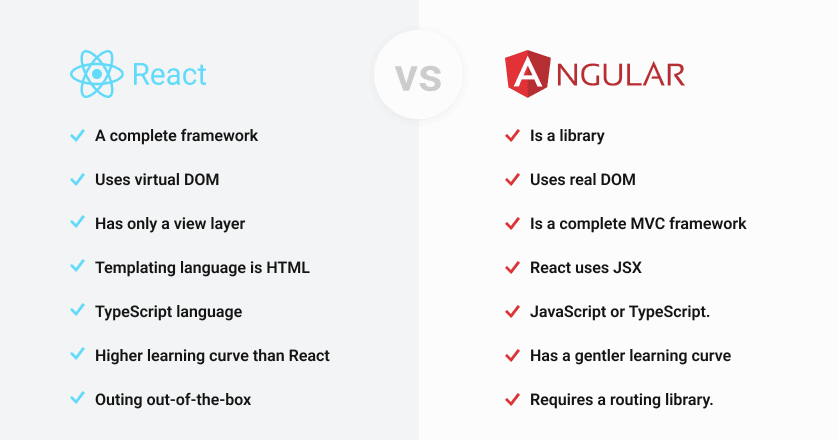
13. What are controlled and uncontrolled components?
Answer:
- Controlled components are those where form elements (like input fields) are controlled by React’s state. The state value is the source of truth, and the form element updates based on state.
- Uncontrolled components are those where the form elements maintain their own state. React doesn’t control the state of the form elements, and you can access the values using refs.
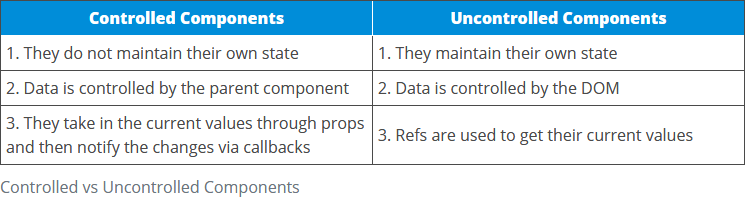
14. What is the purpose of keys in React lists?
Answer:
Keys help React identify which items have changed, been added, or removed in a list. When rendering lists of elements, each element must have a unique key to ensure efficient updates and avoid unnecessary re-renders.
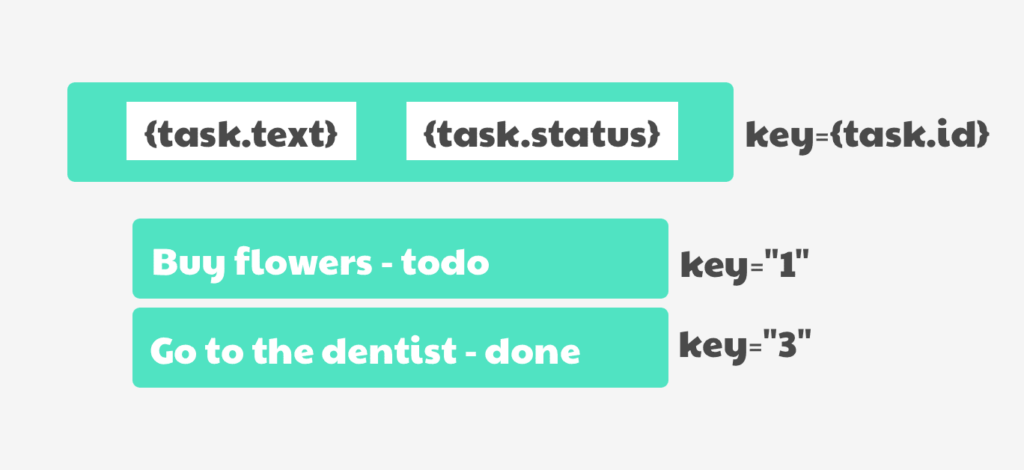
15. What is the context API in React?
Answer:
The Context API is a way to share values (like theme, language, or authentication status) between components without having to pass props through every level of the component tree. It is often used for global state management.
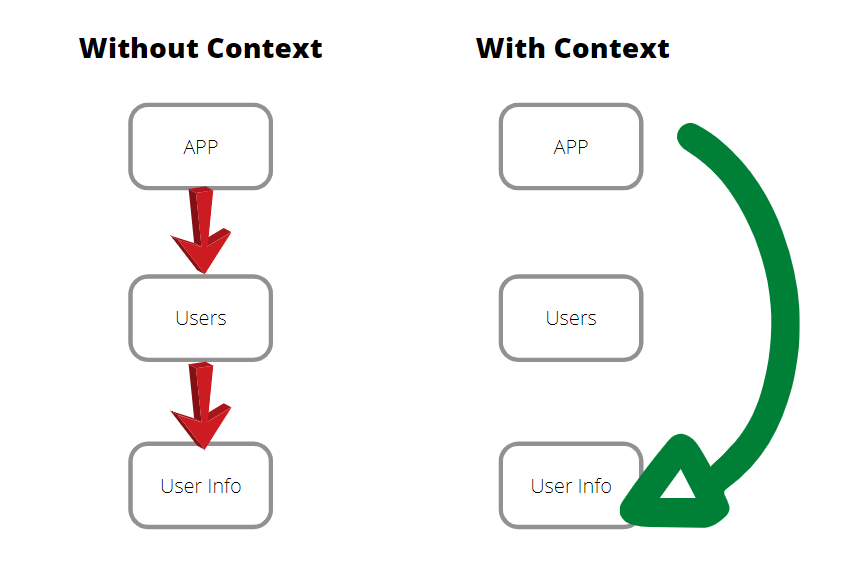
16. What is the difference between a functional component and a class component?
Answer:
- Functional Components: These are simpler components defined as functions. They do not have lifecycle methods or this binding, but can use hooks (like useState, useEffect) for state and lifecycle management.
- Class Components: These are more complex components defined as ES6 classes. They have lifecycle methods (e.g., componentDidMount, render) and use the this keyword to access component properties and state.
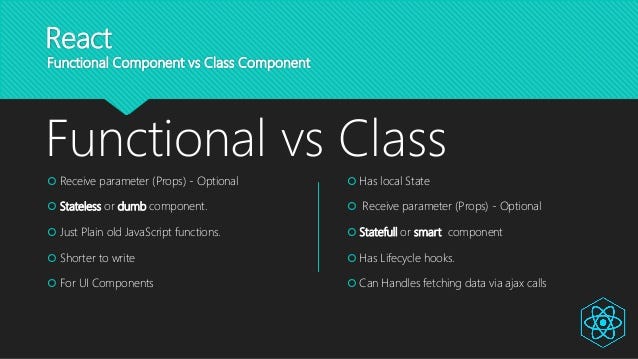
17. What is the use of the render method in React?
Answer:
The render method is a required method in class components. It returns the JSX or React elements that define the component’s UI. It gets called whenever there’s a change in state or props, causing the component to re-render.
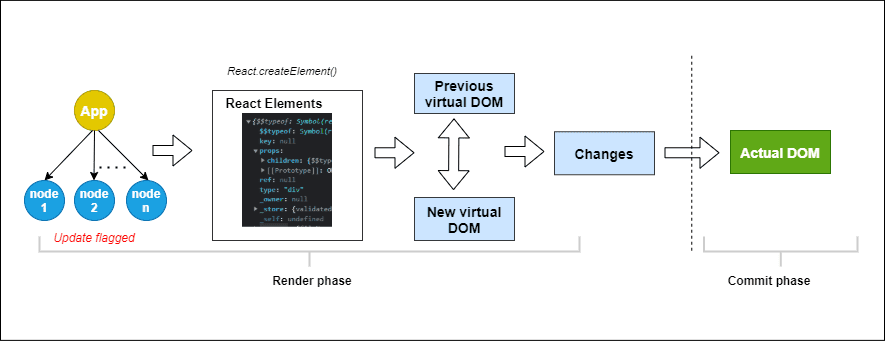
18. What are React Fragments?
Answer:
React Fragments are used to group multiple elements without adding an extra DOM node. They allow you to return multiple elements from a component without wrapping them in a div or another HTML element.
Example:
return (
<>
<h1>Title</h1>
<p>Some content</p>
</>
);
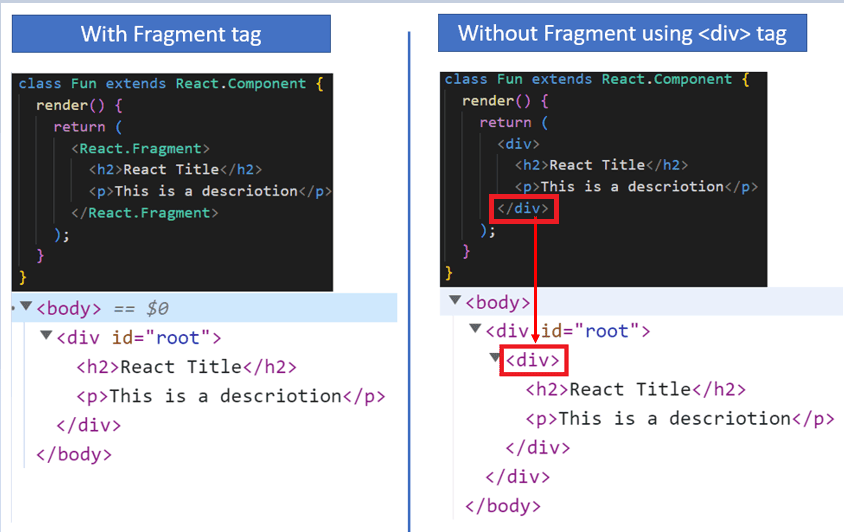
19. What is the use of useRef in React?
Answer:
useRef is a hook used to persist values between renders without causing re-renders. It is often used to reference DOM elements directly or store mutable values that do not need to trigger a re-render.
Example:
const inputRef = useRef(null);
const handleFocus = () => {
inputRef.current.focus();
};
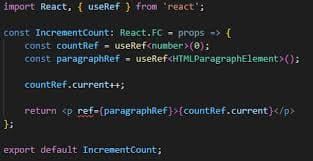
20. What is the purpose of shouldComponentUpdate?
Answer:
shouldComponentUpdate is a lifecycle method in class components that is called before re-rendering the component. It is used to optimize performance by preventing unnecessary re-renders. You can return false to skip re-rendering when the props or state haven’t changed.
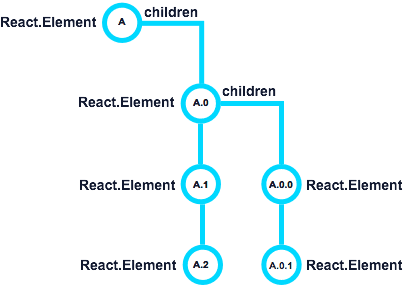
21. What is React.memo?
Answer:
React.memo is a higher-order component that is used to optimize performance in functional components by memoizing the result of a component’s render. If the props do not change, React will skip re-rendering that component, improving performance.
Example:
const MyComponent = React.memo((props) => {
return <div>{props.text}</div>;
});
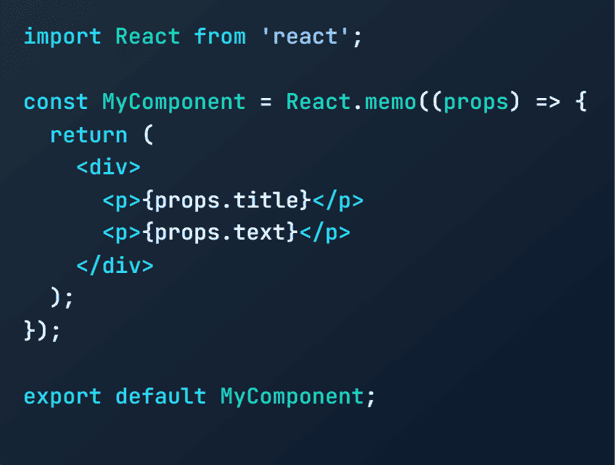
22. What are higher-order components (HOCs) in React?
Answer:
A higher-order component is a function that takes a component and returns a new component with additional props or logic. HOCs allow you to reuse component logic across different components.
Example:
function withExtraInfo(Component) {
return function EnhancedComponent(props) {
return <Component {…props} extra=”Some extra info” />;
};
}
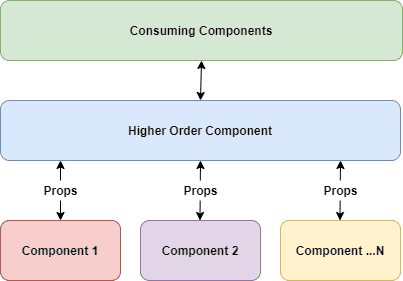
23. What is the difference between componentWillMount and componentDidMount?
Answer:
- componentWillMount: Called before the component is mounted and rendered. It is deprecated in newer versions of React.
- componentDidMount: Called immediately after the component is mounted (rendered). It is a good place for fetching data or setting up subscriptions.
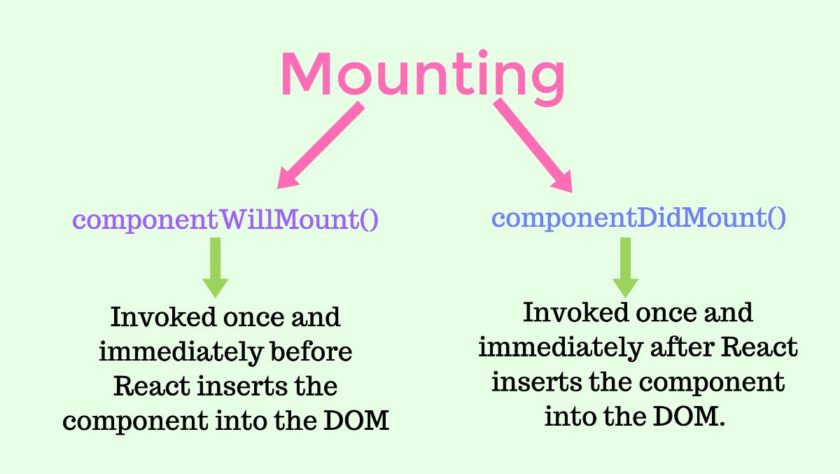
24. What is the useCallback hook used for?
Answer:
The useCallback hook is used to memoize callback functions so that they do not get re-created on every render. It helps prevent unnecessary re-renders of child components that depend on the callback function.
Example:
const memoizedCallback = useCallback(() => {
console.log(‘Callback function’);
}, [dependencies]);
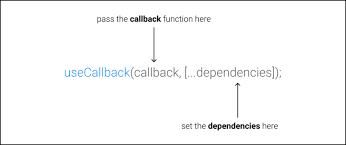
25. What is the difference between useEffect and componentDidMount?
Answer:
- componentDidMount: This is a lifecycle method in class components that is called once the component has been mounted (rendered).
- useEffect: This is a hook in functional components that allows you to perform side effects, such as data fetching or DOM manipulation. It is called after every render by default, but you can control when it runs by passing dependencies.
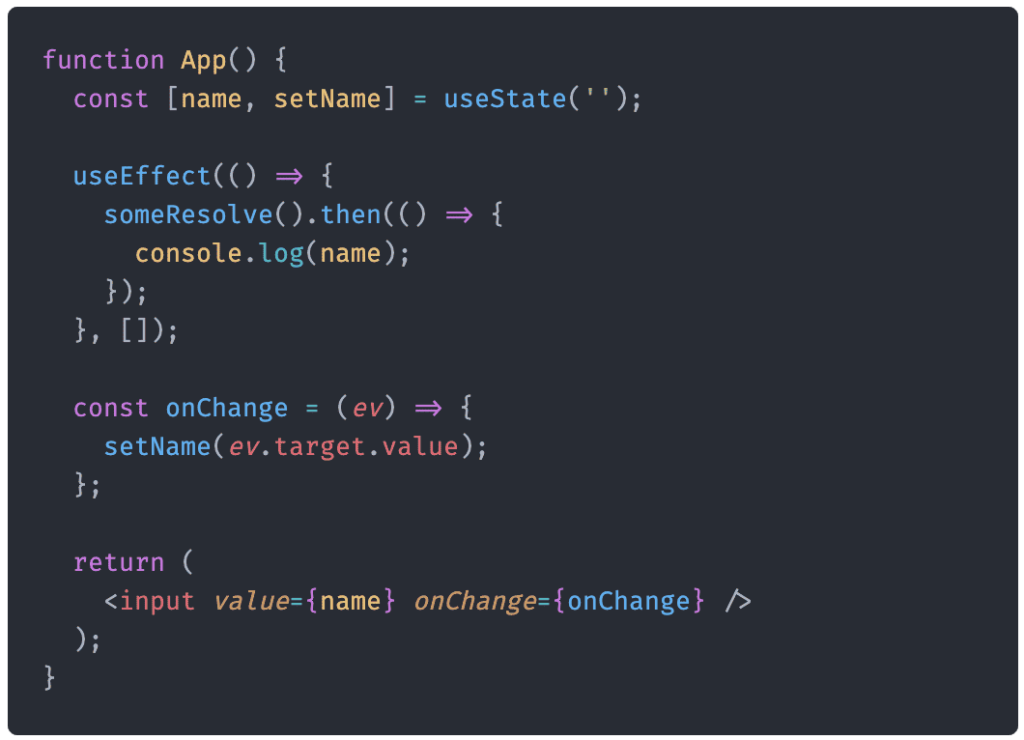
26. What is a React Key prop, and why is it important?
Answer:
The key prop is used to uniquely identify elements in an array or list when rendering dynamic elements in React. Keys help React efficiently update the UI by identifying which elements have changed, been added, or removed. They are necessary for maintaining state when rendering lists of components.
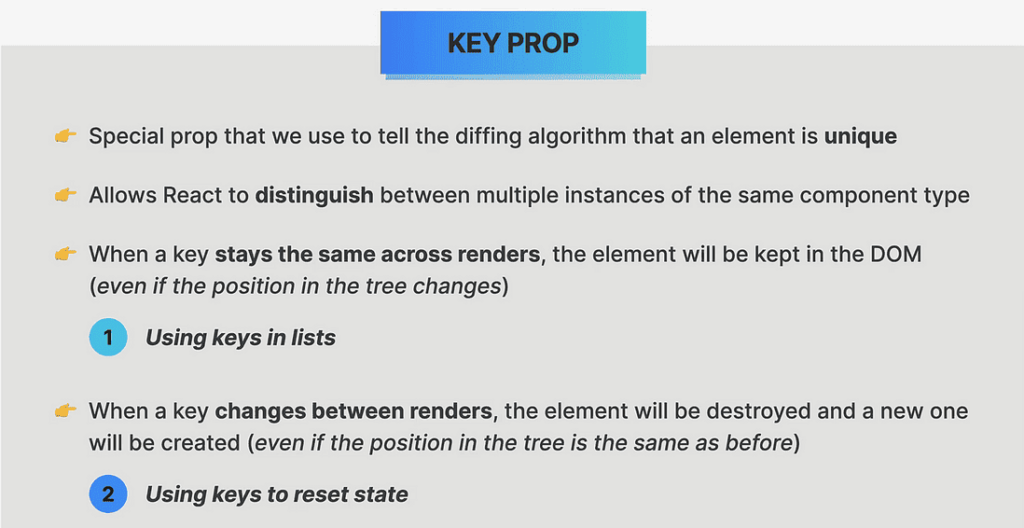
27. What is the Context API in which case you would use it?
Answer:
The Context API is used for state management and sharing data across the component tree without having to pass props manually at every level. It’s commonly used for global state (e.g., authentication, theme) that needs to be accessible throughout the app.
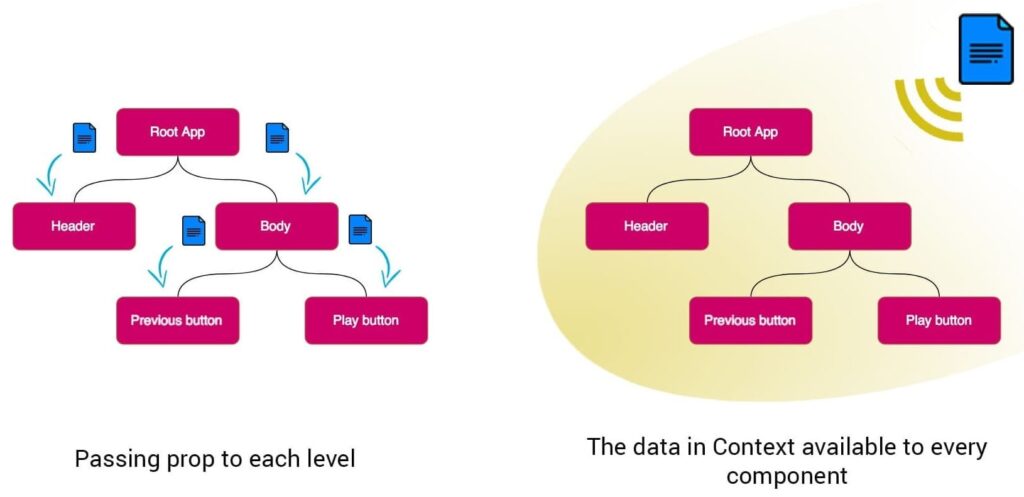
28. What are React hooks, and how do they work?
Answer:
React hooks are functions that allow you to use state and lifecycle features in functional components. They were introduced in React 16.8 to allow functional components to have state and side effects, which were previously only available in class components. Common hooks include useState, useEffect, and useContext.
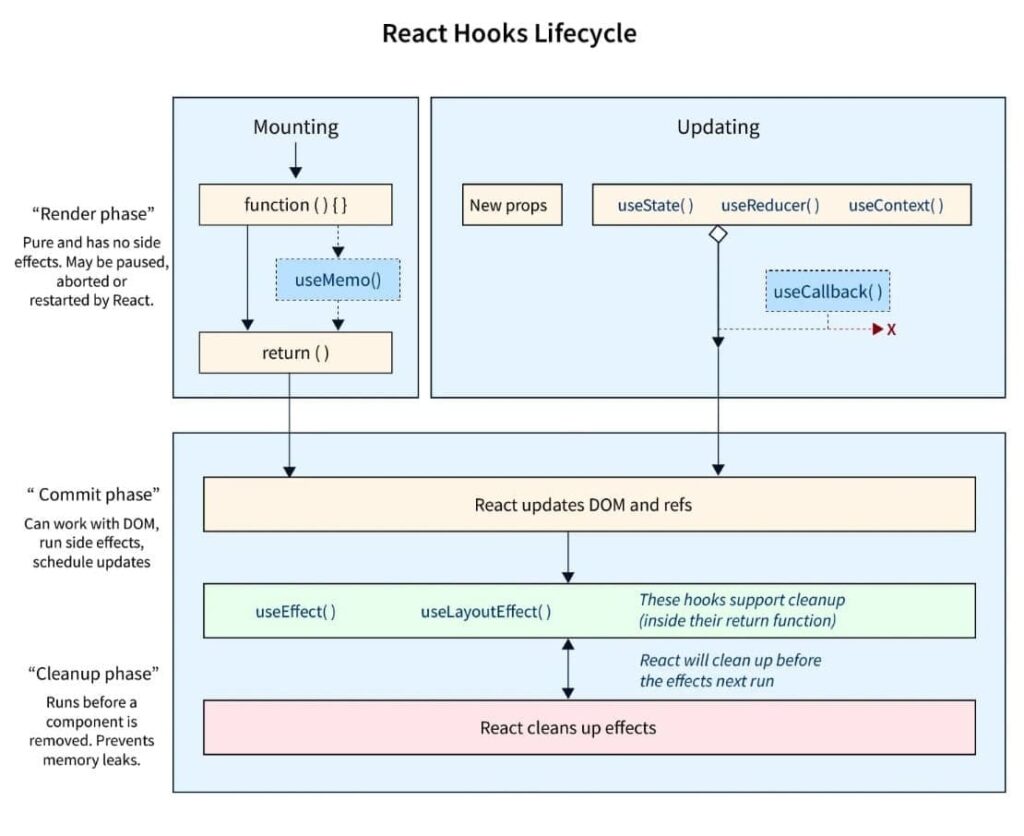
29. What is the difference between == and === in React (JavaScript)?
Answer:
- == is the equality operator that compares values after performing type conversion.
- === is the strict equality operator that compares both value and type without performing type conversion. It is generally recommended to use === in JavaScript for type safety.
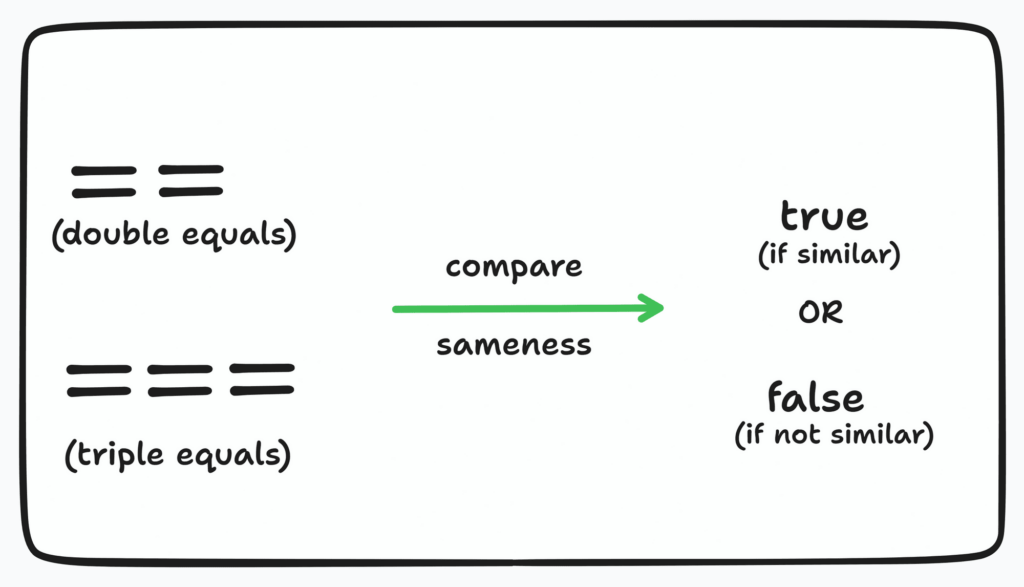
30. What are "dangerouslySetInnerHTML" and when should you use it?
Answer:
dangerouslySetInnerHTML is a React property that allows you to set HTML content directly within a component. It’s called “dangerous” because it can expose your app to cross-site scripting (XSS) attacks if the content isn’t properly sanitized.
Example:
<div dangerouslySetInnerHTML={{ __html: ‘<p>Some HTML content</p>’ }} />
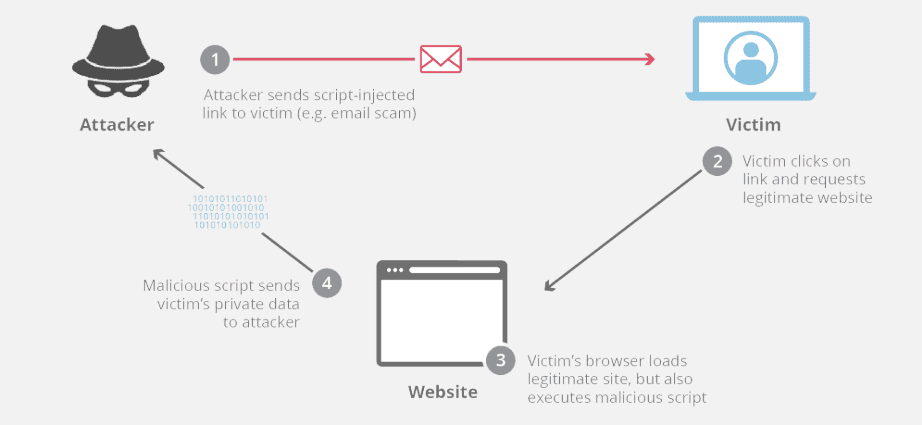
31. What is a pure component in React?
Answer:
A PureComponent in React is a component that only re-renders when its props or state have changed. It implements the shouldComponentUpdate lifecycle method with a shallow prop and state comparison, which can help optimize performance.
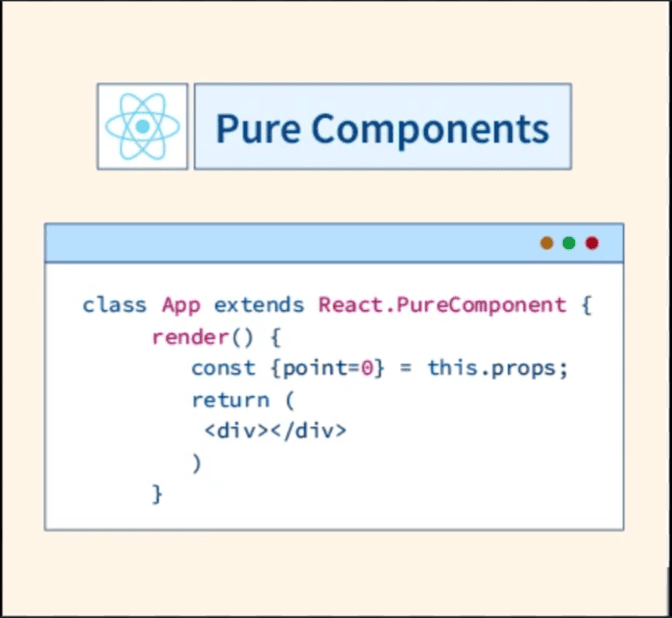
32. What is the useLayoutEffect hook, and how is it different from useEffect?
Answer:
useLayoutEffect is similar to useEffect, but it runs synchronously after all DOM mutations. It is used when you need to perform DOM measurements or synchronous updates before the browser paints. On the other hand, useEffect runs asynchronously after the paint.
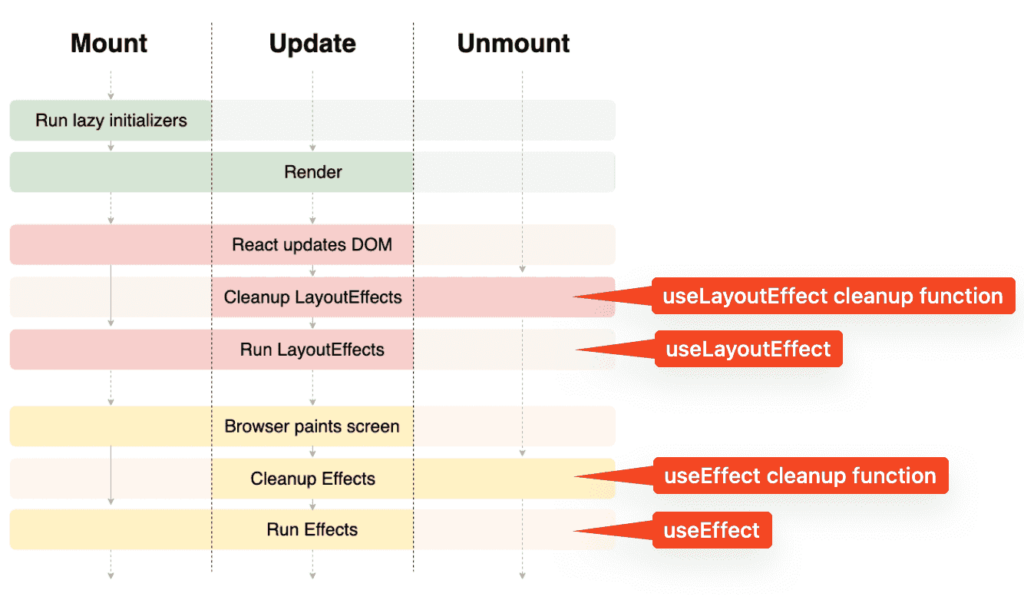
33. What is an event handler in React, and how do you handle events?
Answer:
An event handler in React is a function triggered when a specific event occurs (e.g., a button click). React uses a synthetic event system, which normalizes events across different browsers. To handle events, you pass an event handler function to the JSX element’s event property (e.g., onClick, onChange).
Example:
const handleClick = () => {
console.log(‘Button clicked’);
};
<button onClick={handleClick}>Click me</button>
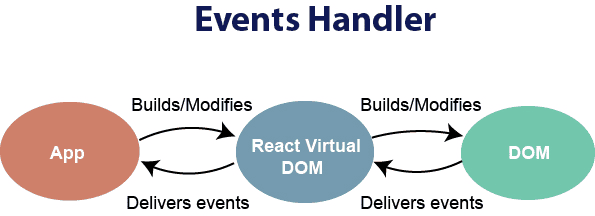
34. What is the dangerouslySetInnerHTML property?
Answer:
dangerouslySetInnerHTML is a property in React that allows you to inject raw HTML content into the DOM. It is “dangerous” because it can lead to cross-site scripting (XSS) vulnerabilities if the content is not sanitized properly.
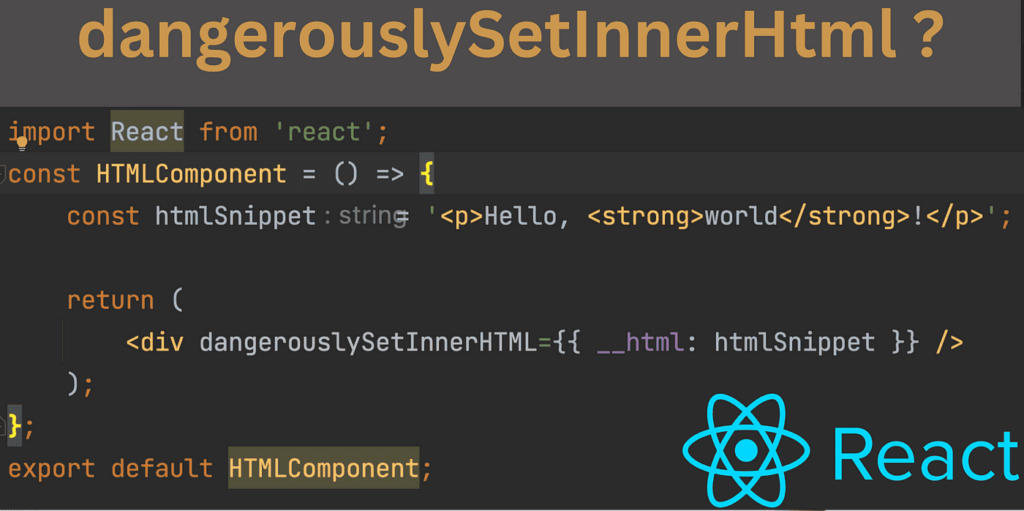
35. What are controlled and uncontrolled components in React?
Answer:
- Controlled Components: These are components where the form element’s value is controlled by React’s state. React is the source of truth for the form’s data.
- Uncontrolled Components: These are components where the form element maintains its own state. The data can be accessed using refs.
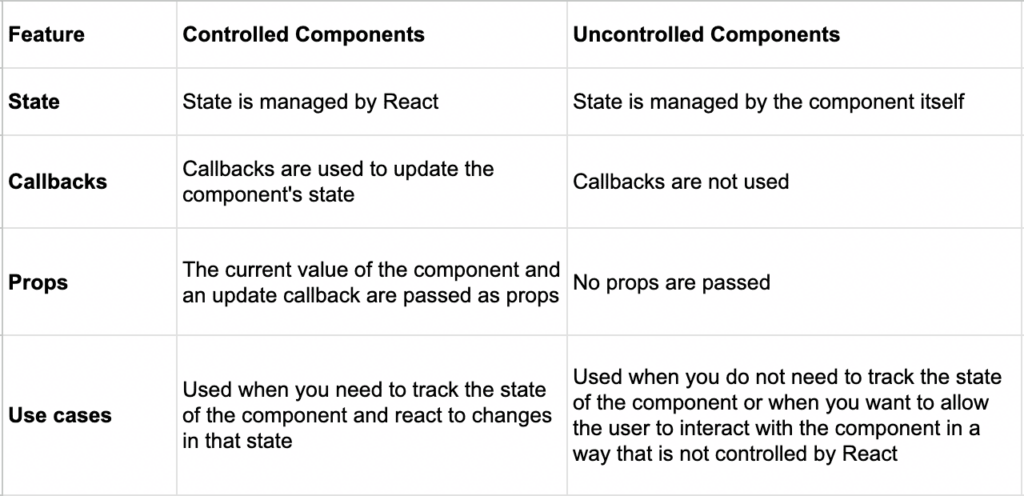
36. What are React's synthetic events?
Answer:
Synthetic events are a cross-browser wrapper around the browser’s native event system. React uses a synthetic event system to normalize events so that they behave consistently across different browsers. They provide methods like preventDefault(), stopPropagation(), and persist().
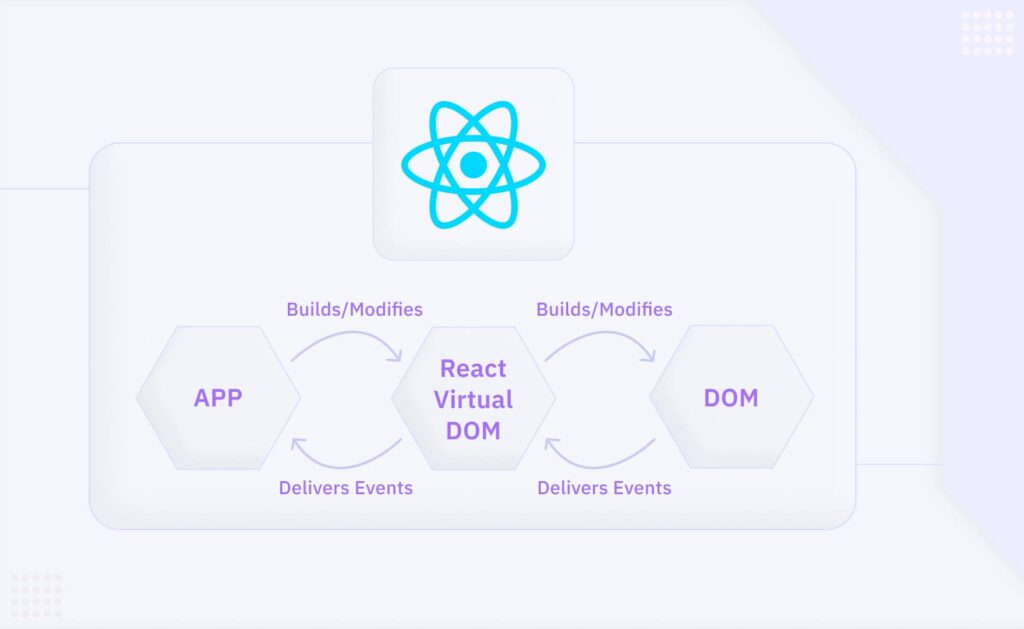
37. What is the difference between useEffect and useLayoutEffect?
Answer:
- useEffect: It runs after the component renders and updates the DOM, making it suitable for handling side effects such as data fetching.
- useLayoutEffect: It runs synchronously after DOM mutations but before the browser repaints the screen. It is useful for DOM measurements and making DOM changes that need to be reflected before the user sees them.
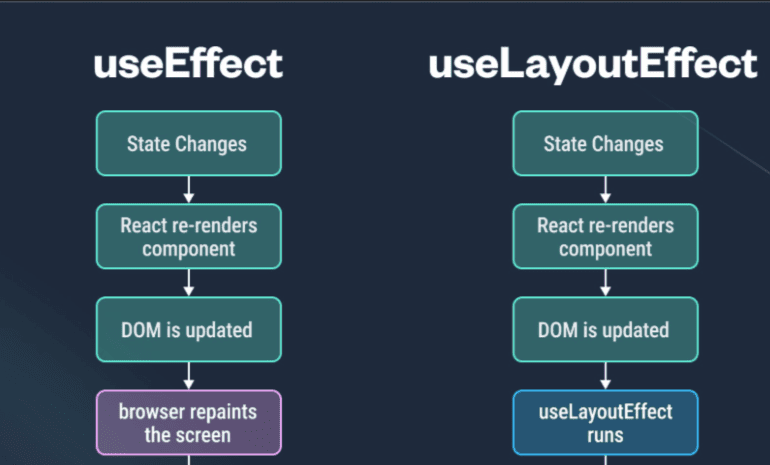
38. What are prop types in React?
Answer:
Prop types are a way to validate the props passed to a component. React provides the prop-types library to specify the expected types of props and warn developers if the types do not match. This helps in debugging and maintaining the code.
Example:
MyComponent.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number
};
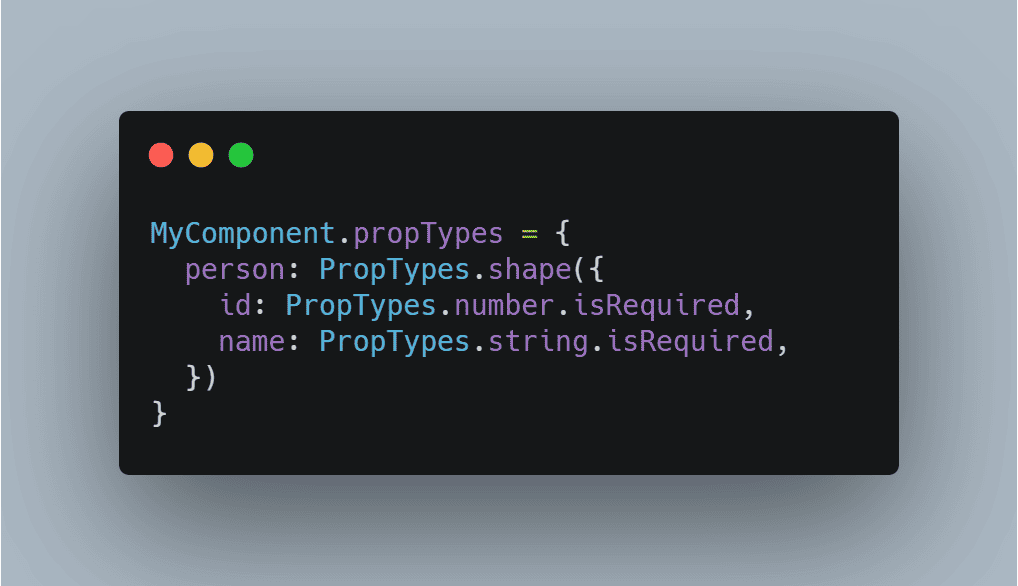
39. What is the significance of the key prop in React lists?
Answer:
The key prop is essential for React to identify which items have changed, been added, or removed. It helps React optimize rendering by only updating the changed elements rather than re-rendering the entire list.
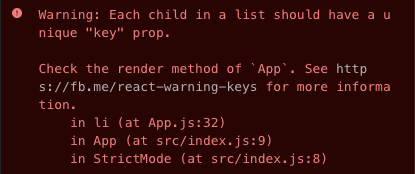
40. How do you conditionally render elements in React?
Answer:
You can conditionally render elements in React using JavaScript expressions. Common approaches include using ternary operators or logical && operator.
Example:
const isLoggedIn = true;
return isLoggedIn ? <h1>Welcome</h1> : <h1>Please log in</h1>;
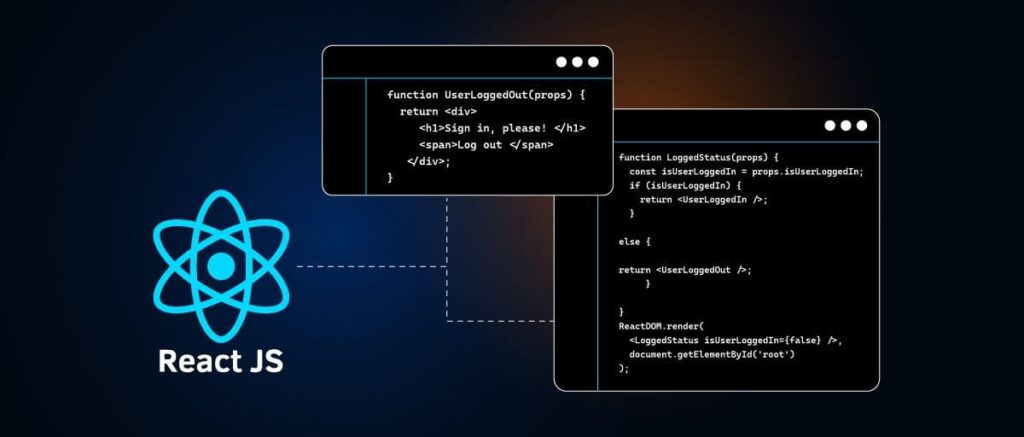
41. What is the purpose of React.StrictMode?
Answer:
React.StrictMode is a wrapper component that helps identify potential problems in an application. It does not render any visible UI but activates additional checks and warnings for its descendants, helping developers detect issues such as deprecated APIs or unsafe lifecycle methods.
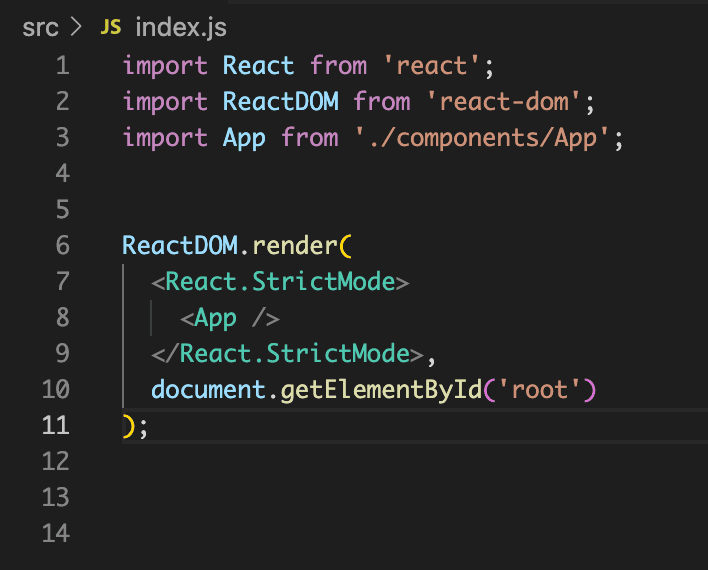
42. What are error boundaries in React?
Answer:
Error boundaries are components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI. They prevent an entire React application from crashing when an error occurs in a specific part of the UI.
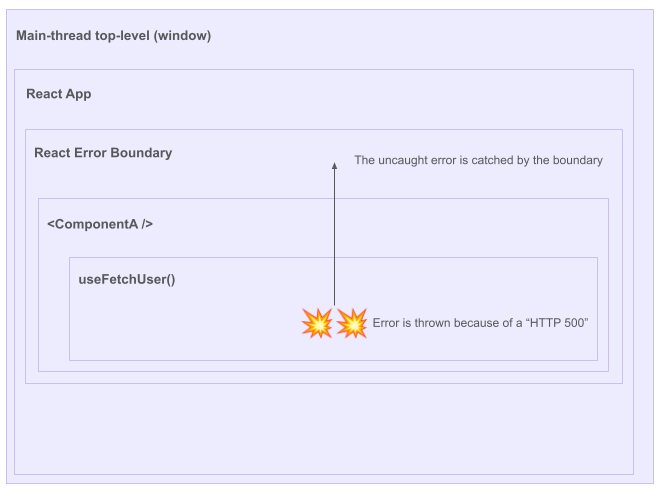
43. What is the useReducer hook used for in React?
Answer:
useReducer is a hook used to manage complex state logic in React components. It is an alternative to useState when state transitions depend on the previous state or when managing multiple state variables.
Example:
const [state, dispatch] = useReducer(reducer, initialState);
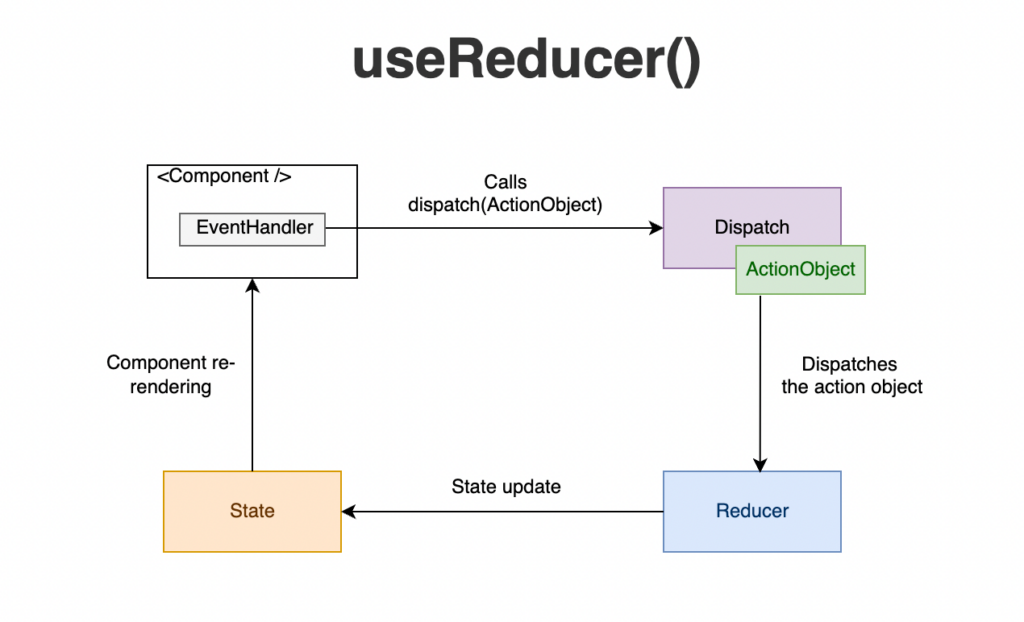
44. What are portals in React?
Answer:
Portals provide a way to render children into a DOM node that exists outside the hierarchy of the parent component. This is useful for rendering modal dialogs, tooltips, and other UI elements that need to be rendered outside the component tree.
Example:
ReactDOM.createPortal(child, container);
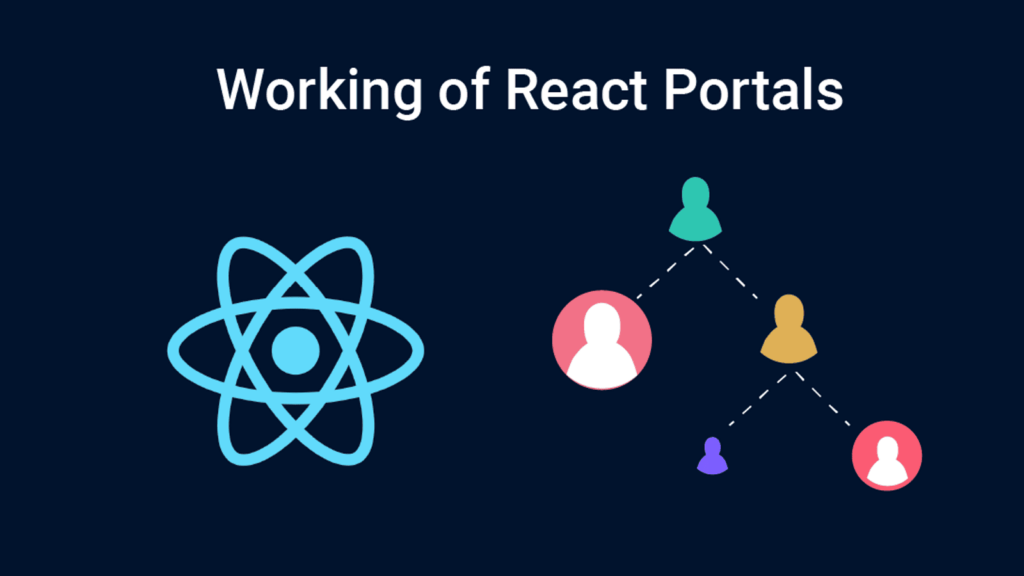
45. What is the useContext hook in React?
Answer:
useContext is a hook that allows you to consume values from a context provider in functional components. It provides a way to share state or data across components without having to pass props manually at every level.
Example:
const value = useContext(MyContext);
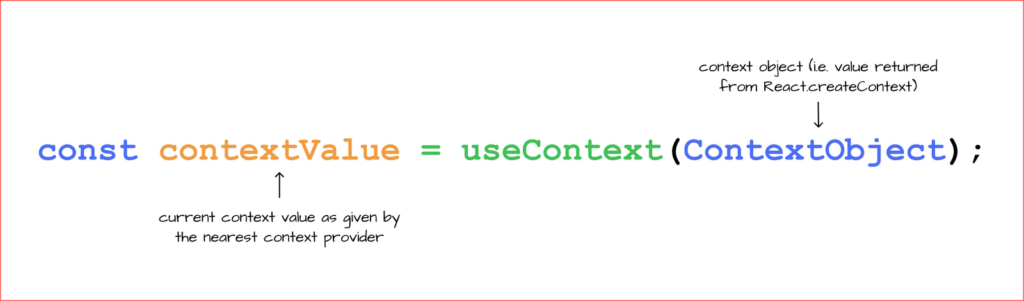
46. What is the difference between a component’s render method and the return statement in functional components?
Answer:
- render method: Used in class components to return JSX.
- return statement: Used in functional components to return JSX. Functional components don’t have a render method; they return JSX directly from the function.
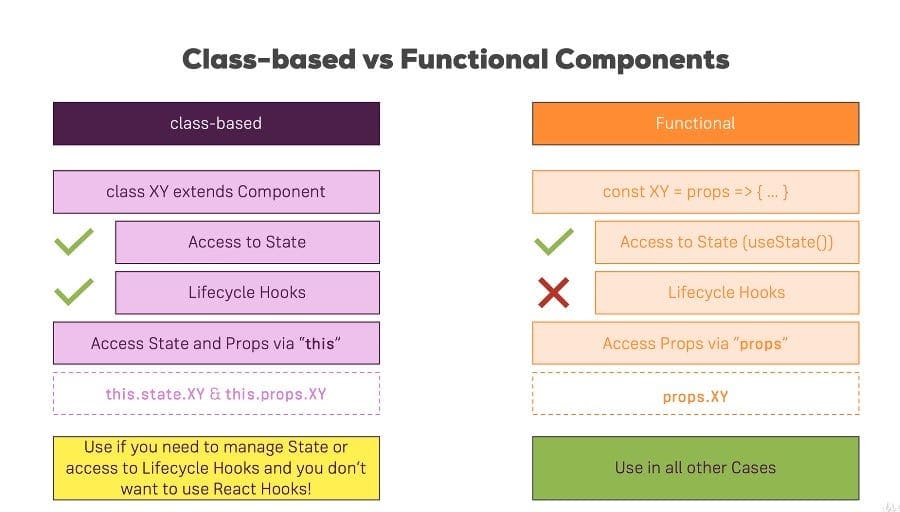
47. What are higher-order functions (HOF) in React?
Answer:
Higher-order functions are functions that take one or more functions as arguments or return a function. In React, higher-order components (HOCs) are an example of HOFs, where a function wraps another component to enhance its functionality.
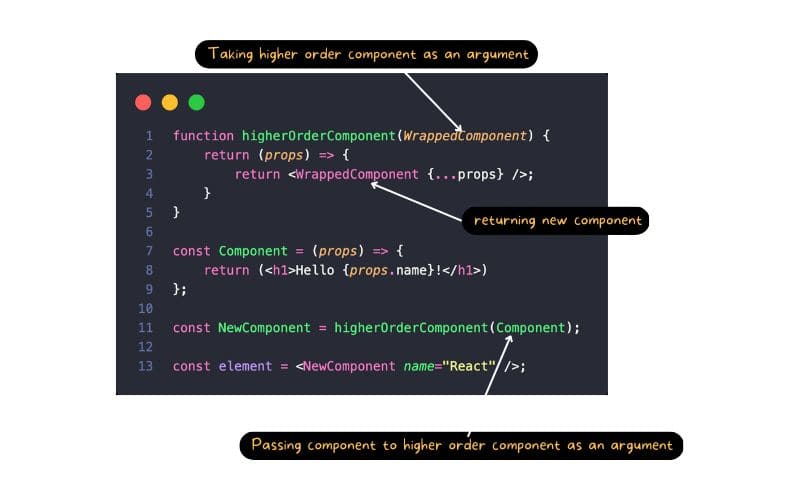
48. What are keys used for in React list rendering?
Answer:
Keys are used to identify which items in the list have changed, been added, or removed. They help React optimize rendering and avoid re-rendering unchanged elements in a list.
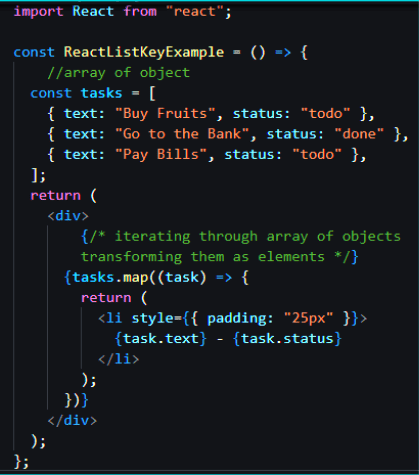
49. What is the useImperativeHandle hook in React?
Answer:
useImperativeHandle is a hook used with forwardRef to customize the instance value that is exposed to the parent component when using refs. It allows you to expose only specific methods or properties to the parent.
Example:
useImperativeHandle(ref, () => ({
focus: () => inputRef.current.focus(),
}));
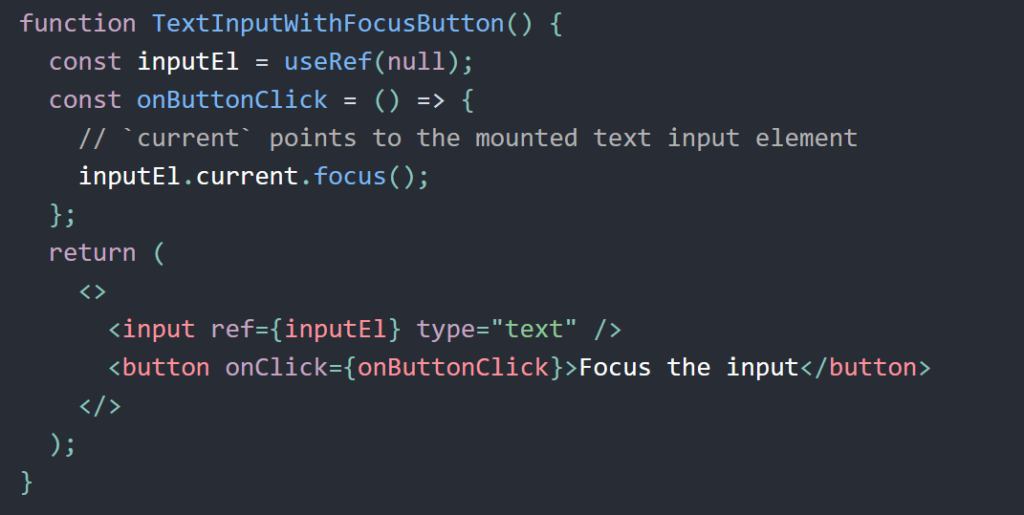
50. What is server-side rendering (SSR) in React?
Answer:
Server-side rendering (SSR) is the process of rendering a React application on the server and sending the fully rendered HTML to the client. This can improve performance and SEO because the page is immediately ready for the user, and search engines can crawl the content.
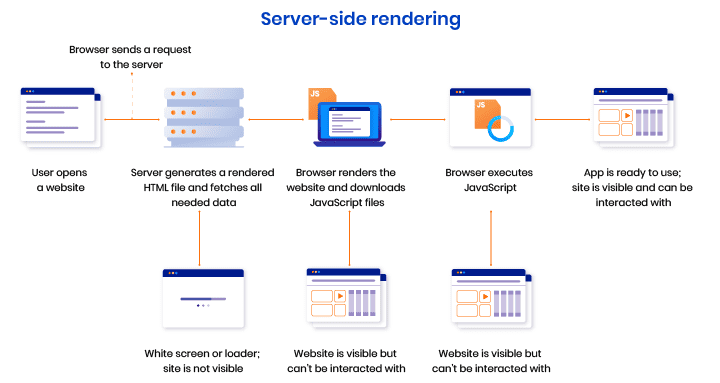
React JS Interview Questions and Answers – Get Expert Interview Guidance from Brolly Academy
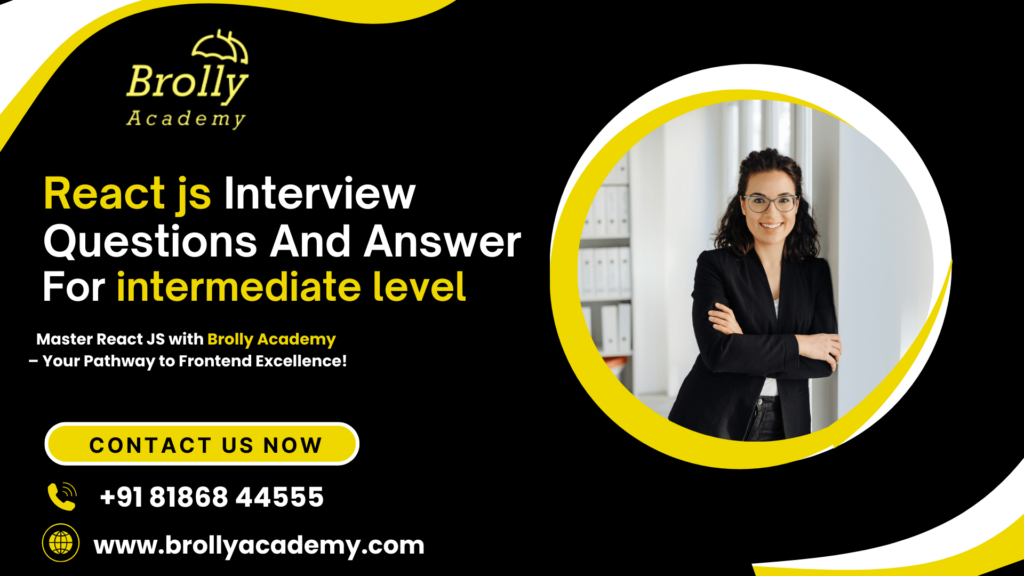
React js Interview Questions And Answer For intermediate level
1. What is React's reconciliation algorithm?
Answer:
React uses a reconciliation algorithm (also known as the “diffing” algorithm) to compare the virtual DOM with the real DOM and update only the changed parts. It uses a heuristic approach to efficiently determine which components need to be updated. React compares elements with the same type, and if the elements have different keys or props, it will replace them. It minimizes the number of DOM updates to improve performance.
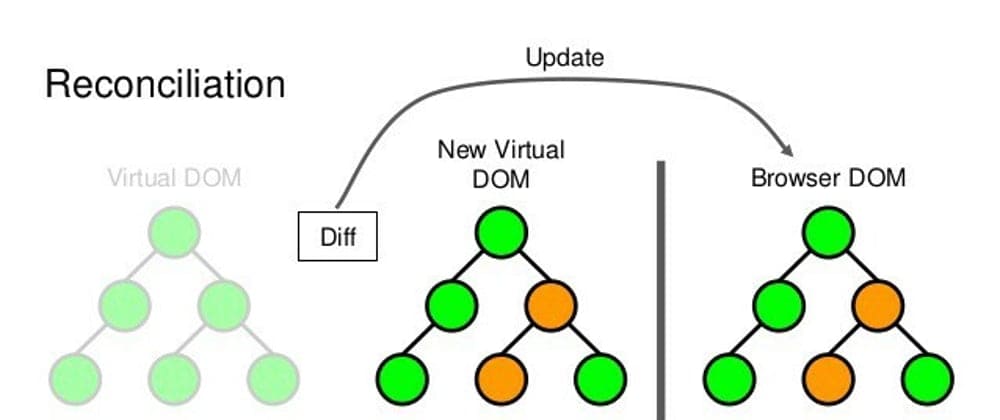
2. What is the significance of keys in React lists, and what happens if keys are not used?
Answer:
Keys help React identify which items have changed, been added, or removed, making the process of re-rendering more efficient. If keys are not used, React will re-render the entire list, even if only one item has changed. This can lead to performance issues and improper UI updates.
3. What is the purpose of React.memo and how does it work?
Answer:
React.memo is a higher-order component that memoizes a functional component. It prevents unnecessary re-renders by comparing the current props with the previous ones. If the props have not changed, React skips the render. This is useful for optimizing performance in functional components that receive the same props frequently.
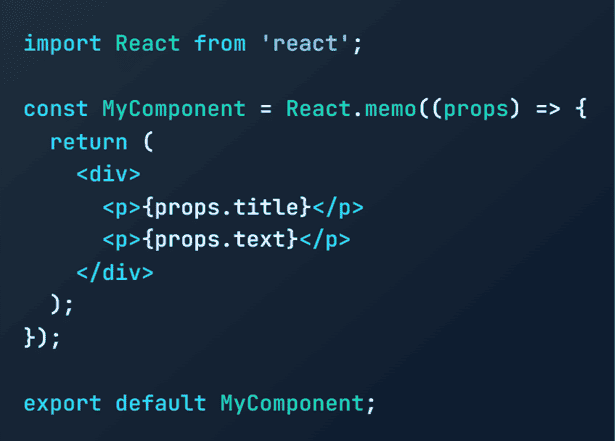
4. What are hooks and why were they introduced in React?
Answer:
Hooks were introduced in React 16.8 to allow functional components to use state and lifecycle features without needing to convert them to class components. They provide a simpler and cleaner way to manage state, side effects, and context in functional components. Some popular hooks include useState, useEffect, useContext, and useReducer.
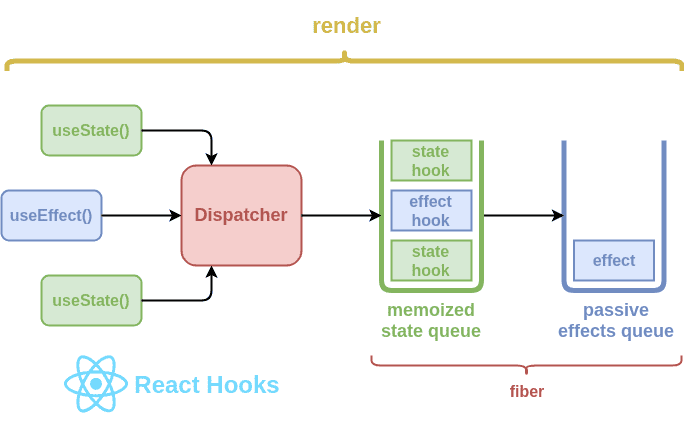
5. What is the difference between useState and useReducer?
Answer:
- useState is used for managing simple state in a component. It is ideal for scenarios where the state depends on user input or requires minimal updates.
- useReducer is typically used for more complex state management, such as when the state has multiple values or the state changes based on previous state values. It is often used as an alternative to useState in large-scale applications with more intricate state logic.
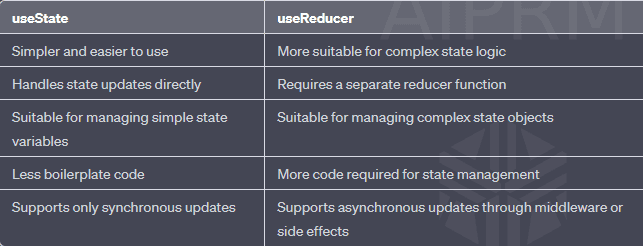
6. What is the Context API in React, and when would you use it?
Answer:
The Context API is a way to share global state across the component tree without having to pass props manually at each level. It is useful for scenarios where you need to access common values (e.g., authentication state, theme, language preferences) in multiple components. It consists of a provider (to provide the data) and a consumer (to access the data).
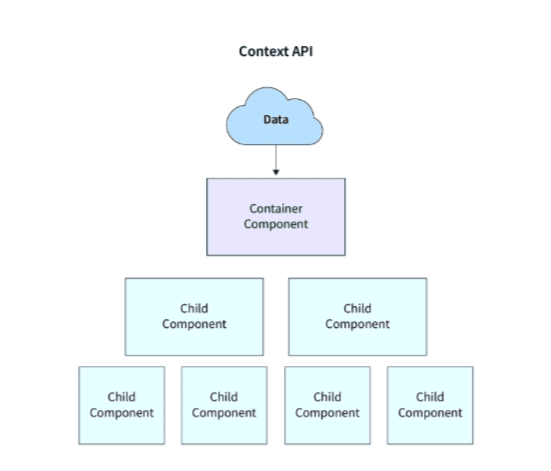
7. Explain how the useEffect hook works and when you should use it.
Answer:
useEffect is used to perform side effects in functional components, such as data fetching, DOM manipulation, or setting up subscriptions. It runs after the component has rendered, and it can be controlled with dependency arrays to specify when the effect should be triggered. If no dependencies are provided, the effect runs after every render.
Example:
useEffect(() => {
// This runs after the component mounts or updates
fetchData();
}, [dependencies]);
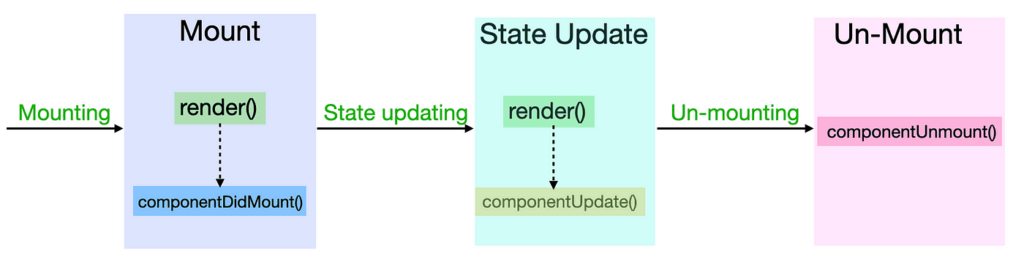
8. What are "controlled components" and "uncontrolled components" in React?
Answer:
- Controlled Components: These components have their form data (e.g., input values) controlled by React’s state. The component’s state is the single source of truth.
Uncontrolled Components: These components store their form data within the DOM and do not rely on React’s state. The data can be accessed using refs.
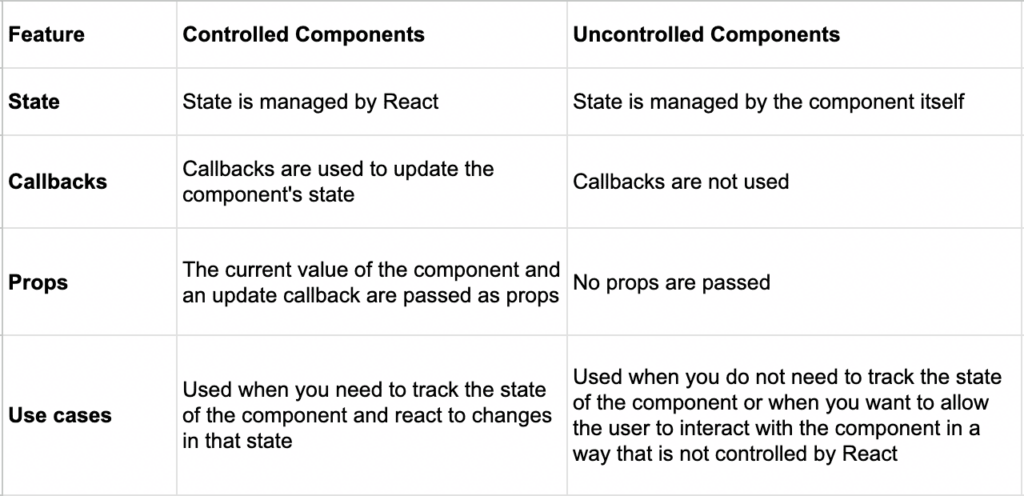
9. What is a higher-order component (HOC) in React?
Answer:
A higher-order component (HOC) is a function that takes a component and returns a new component with additional props or functionality. HOCs are used to reuse component logic, such as adding authentication checks or handling common behaviors across multiple components.
Example:
function withAuth(Component) {
return function AuthComponent(props) {
if (!isAuthenticated) {
return <Redirect to=”/login” />;
}
return <Component {…props} />;
};
}
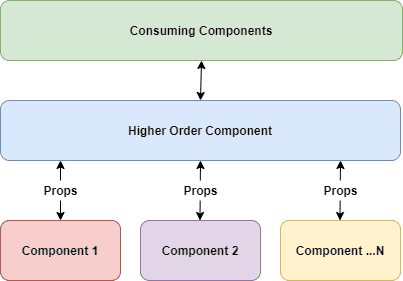
10. What are error boundaries in React?
Answer:
Error boundaries are React components that catch JavaScript errors in their child component tree, log those errors, and display a fallback UI. They prevent the entire application from crashing due to errors in a specific part of the UI. Error boundaries are typically implemented using the componentDidCatch lifecycle method in class components or the static getDerivedStateFromError method.
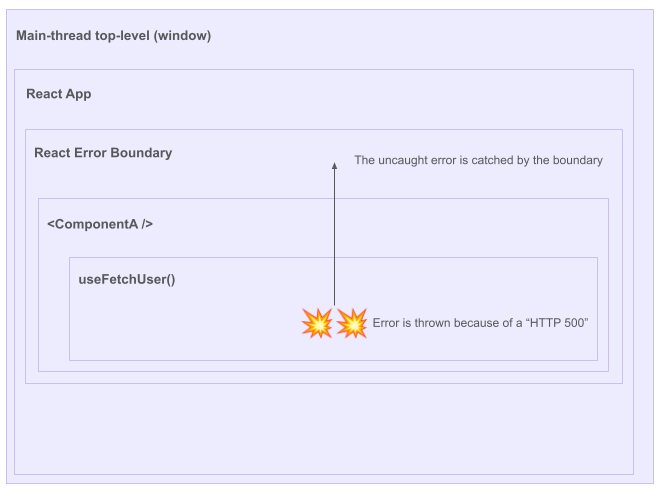
11. What is the significance of the useCallback hook in React?
Answer:
useCallback is a hook used to memoize functions so that they don’t get re-created on every render. It is useful when passing functions as props to child components or when functions depend on specific values and should only be recreated if those values change.
Example:
const memoizedCallback = useCallback(() => {
console.log(‘Callback’);
}, [dependency]);
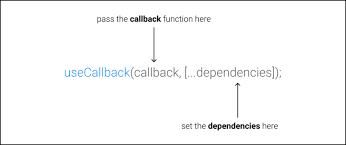
12. How do you optimize performance in a React application?
Answer:
Some common ways to optimize performance in React include:
- Using React.memo to prevent unnecessary re-renders of functional components.
- Using shouldComponentUpdate in class components to control re-renders.
- Code-splitting with React.lazy and Suspense to load components lazily.
- Avoiding anonymous functions and inline object literals within the JSX.
Leveraging the useCallback and useMemo hooks to memoize functions and values.
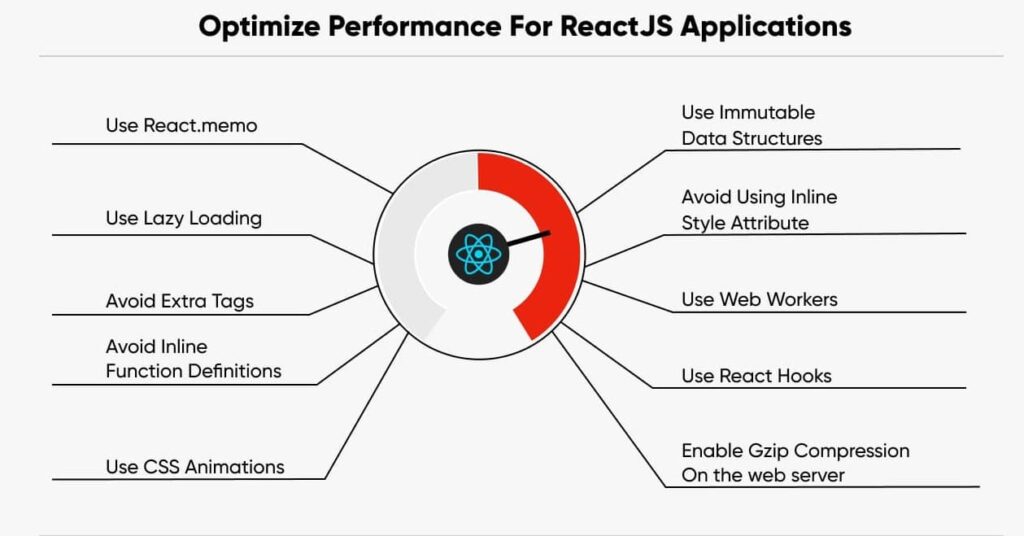
13. What is the difference between useEffect and useLayoutEffect?
Answer:
- useEffect runs after the component renders and the DOM is updated, making it suitable for tasks like fetching data or updating the UI.
- useLayoutEffect runs synchronously after all DOM mutations but before the browser paints the updates. It is useful for reading and modifying the DOM before the user sees the changes (e.g., measuring elements).
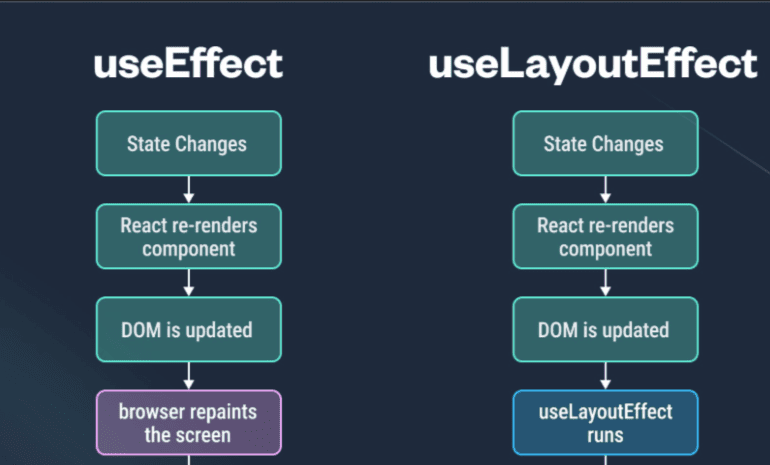
14. What is the useImperativeHandle hook and why would you use it?
Answer:
useImperativeHandle is used with forwardRef to expose specific methods or properties from a child component to the parent. It allows you to customize what is accessible from the parent component when using refs.
Example:
useImperativeHandle(ref, () => ({
focus: () => inputRef.current.focus(),
}));
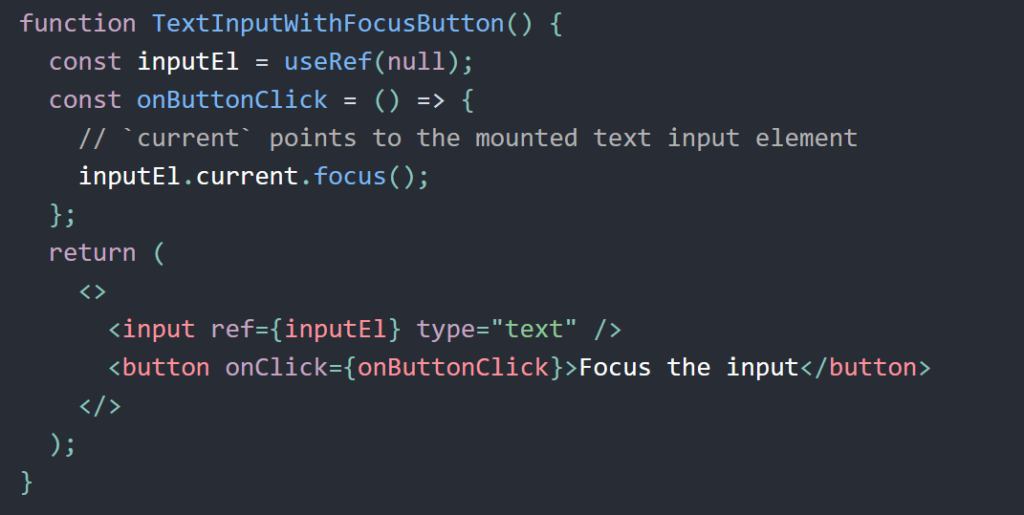
15. What is the difference between componentDidMount and componentWillMount?
Answer:
- componentDidMount is called after the component is mounted and rendered. It is commonly used for tasks like fetching data or initializing external libraries.
- componentWillMount is called before the component is mounted. It is deprecated and should not be used in newer versions of React.
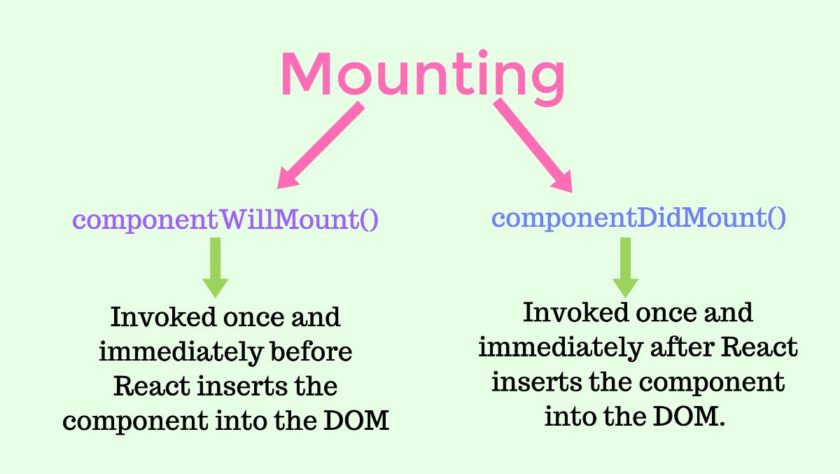
16. What is server-side rendering (SSR) in React?
Answer:
Server-side rendering (SSR) is the process of rendering a React application on the server and sending the fully rendered HTML to the client. This helps improve performance and SEO because the page is pre-rendered before reaching the client, and search engines can crawl the HTML content.
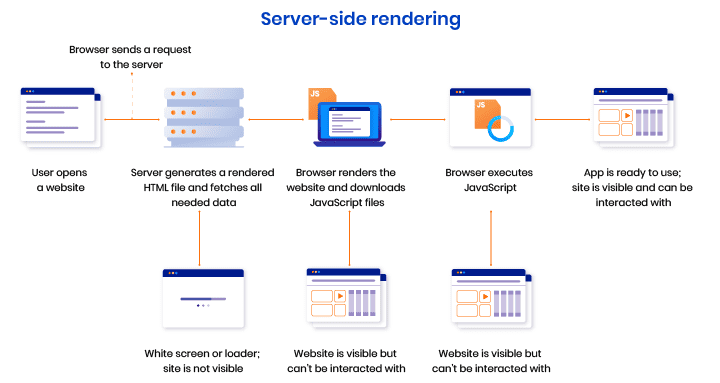
17. What are React Portals?
Answer:
React Portals allow you to render children into a DOM node that exists outside the parent component’s DOM hierarchy. This is useful for elements like modals, tooltips, or dropdowns that need to be rendered outside the main component tree for proper styling and behavior.
Example:
ReactDOM.createPortal(child, container);
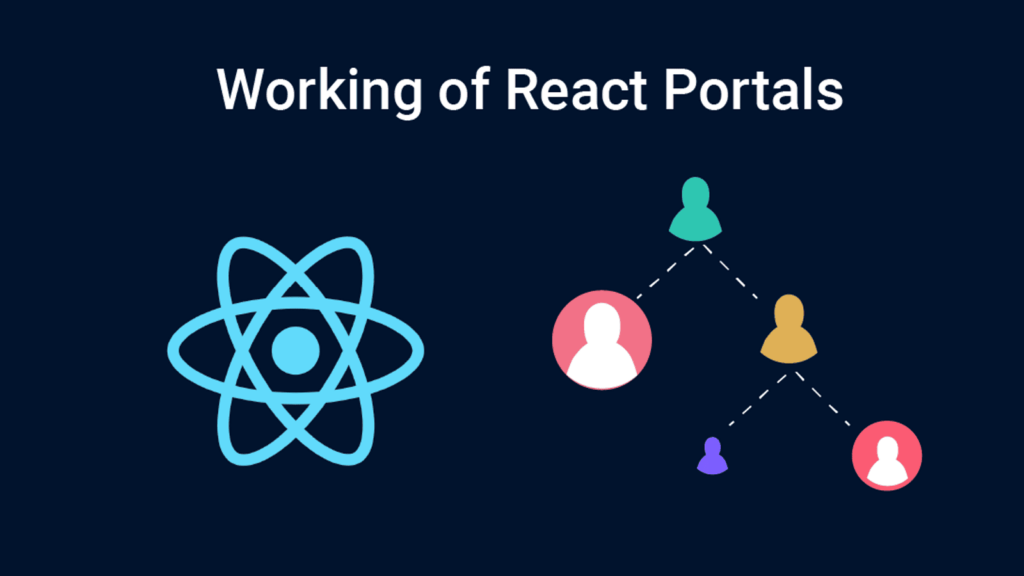
18. How does React handle forms and input validation?
Answer:
React handles forms using controlled components (where form values are managed by state). Input validation can be performed by checking the state value on form submission and updating the UI with error messages if the validation fails.
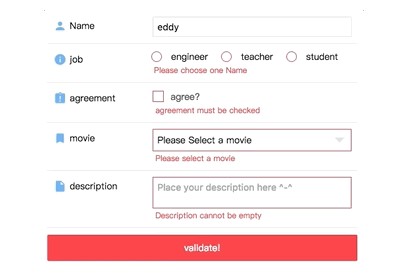
19. What is React.lazy and how do you use it for code splitting?
Answer:
React.lazy is used to lazily load a component only when it is needed. This is a form of code-splitting that improves the initial load time of the application by splitting the code into smaller chunks.
Example:
const MyComponent = React.lazy(() => import(‘./MyComponent’));
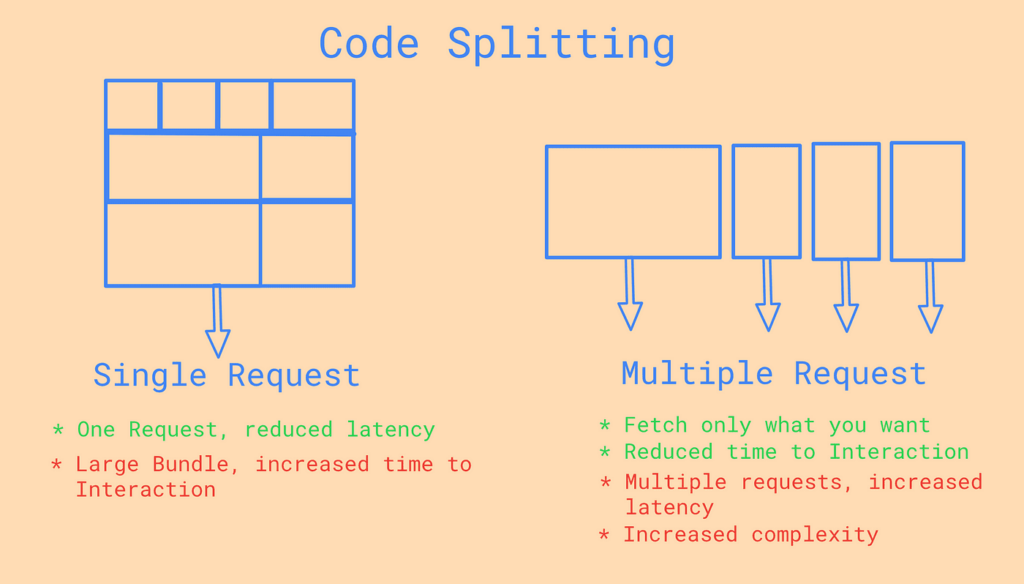
20. What is the difference between useEffect with an empty dependency array [] and without it?
Answer:
- useEffect with an empty dependency array []: The effect runs only once when the component mounts and not on subsequent re-renders. This is similar to componentDidMount in class components.
- useEffect without a dependency array: The effect runs after every render, even if the props or state haven’t changed.
Certainly! Continuing from where we left off:
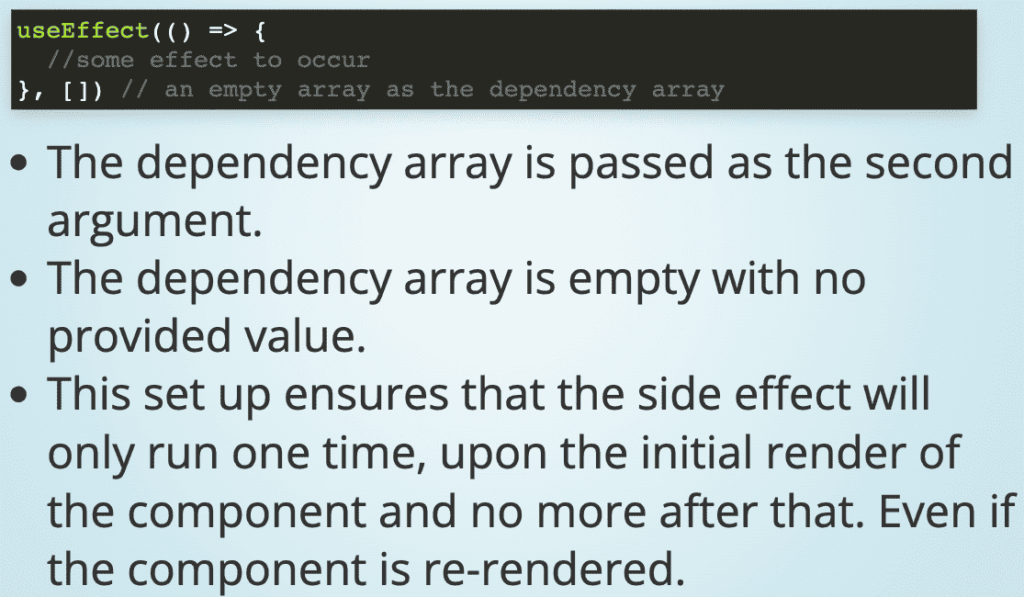
21. What are synthetic events in React?
Answer:
Synthetic events in React are wrapper objects around the native DOM events. React normalizes events so that they have consistent behavior across all browsers. These events are created using the Event system and are passed to event handlers in the React application. They are pooled, which means that the event object is reused across multiple events for performance reasons.
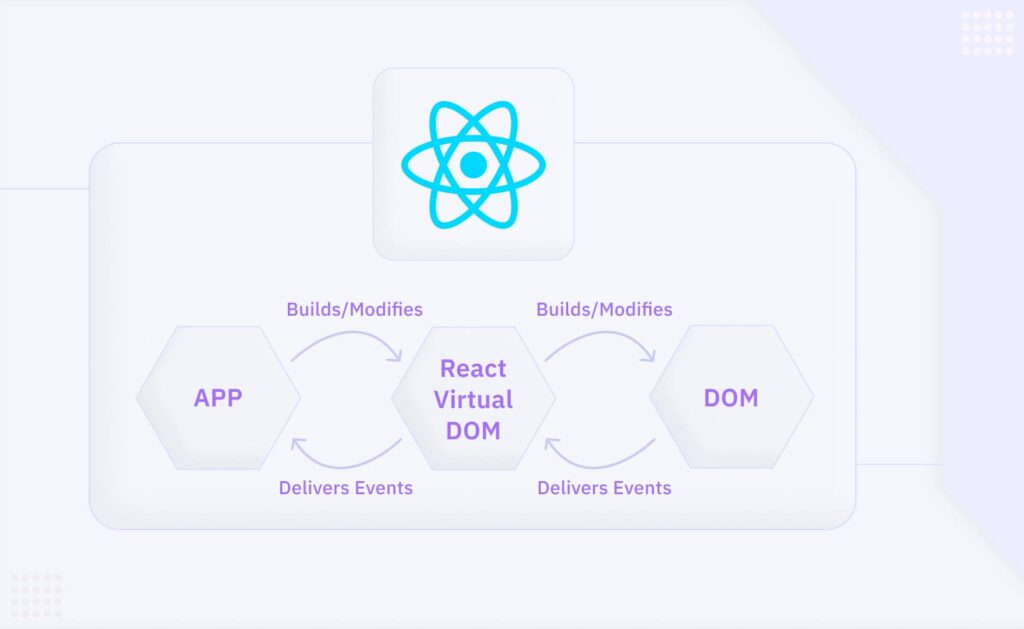
22. What is the purpose of useLayoutEffect hook in React?
Answer:
useLayoutEffect is similar to useEffect but it fires synchronously after all DOM mutations. It is used when you need to make DOM updates (like reading layout values or making measurements) before the browser paints the screen. This is useful for avoiding flicker or layout shifts. Unlike useEffect, which runs after painting, useLayoutEffect runs before the screen update.
Example:
useLayoutEffect(() => {
const rect = myElementRef.current.getBoundingClientRect();
console.log(rect);
}, []);
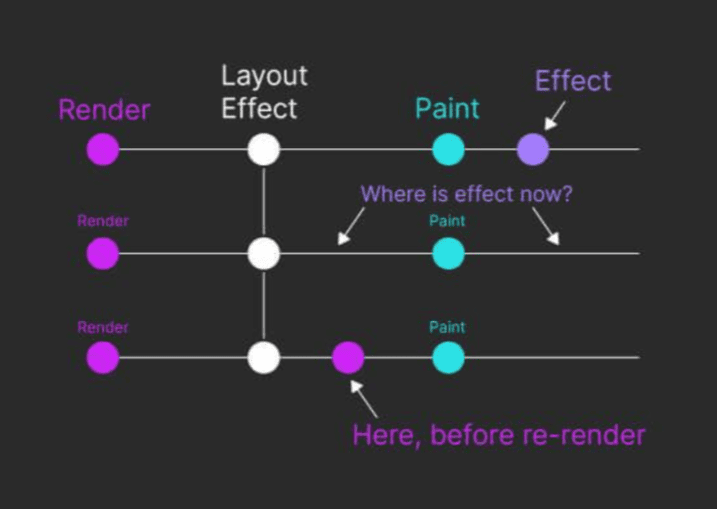
23. How would you create a controlled input in React?
Answer:
A controlled input in React is an input element whose value is controlled by React state. The state of the component is updated whenever the user types in the input, and the input value is bound to this state.
Example:
const [value, setValue] = useState(“”);
const handleChange = (e) => {
setValue(e.target.value);
};
return <input type=”text” value={value} onChange={handleChange} />;
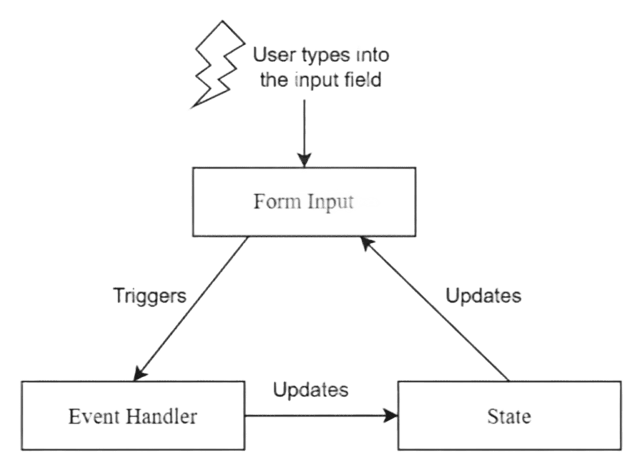
24. What is the useEffect hook's dependency array and how does it work?
Answer:
The dependency array of useEffect specifies when the effect should run. If the array is empty ([]), the effect runs only once after the initial render (similar to componentDidMount). If it contains values, the effect runs whenever any of the values in the array change.
Example:
useEffect(() => {
fetchData();
}, [data]); // Effect will run whenever “data” changes
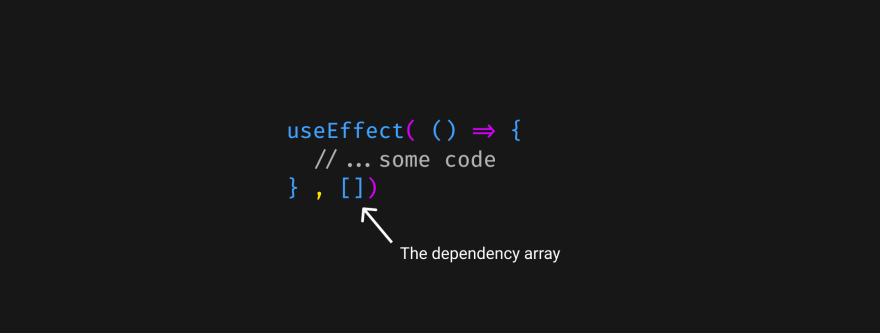
25. What are fragments in React and why would you use them?
Answer:
Fragments are a way to group multiple elements without adding extra nodes to the DOM. React.Fragment allows you to return multiple elements from a component without wrapping them in a single parent element, which helps avoid unnecessary DOM nodes.
Example:
return (
<React.Fragment>
<h1>Hello</h1>
<p>Welcome to React</p>
</React.Fragment>
);
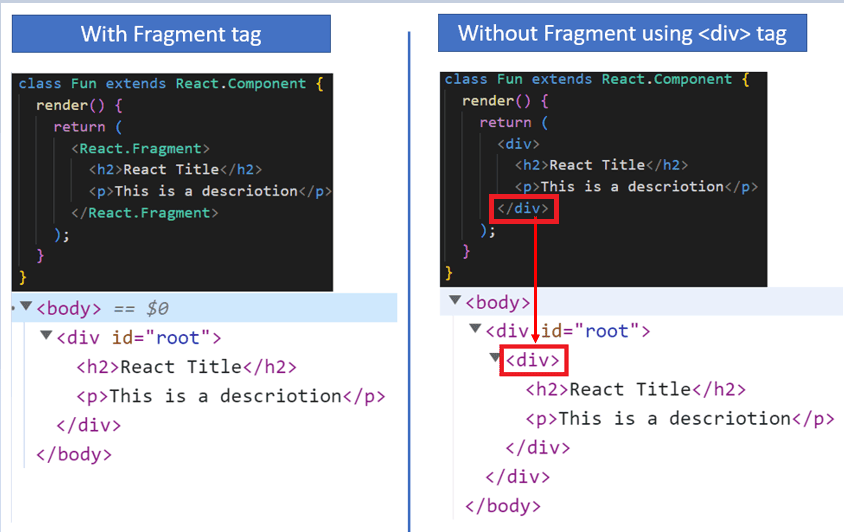
26. What is lazy loading in React?
Answer:
Lazy loading in React allows you to load components only when they are required, which improves the initial loading time of the application. React’s React.lazy can be used to dynamically import components, while Suspense can be used to display a loading state while the component is being fetched.
Example:
const LazyComponent = React.lazy(() => import(‘./LazyComponent’));
return (
<Suspense fallback={<div>Loading…</div>}>
<LazyComponent />
</Suspense>
);
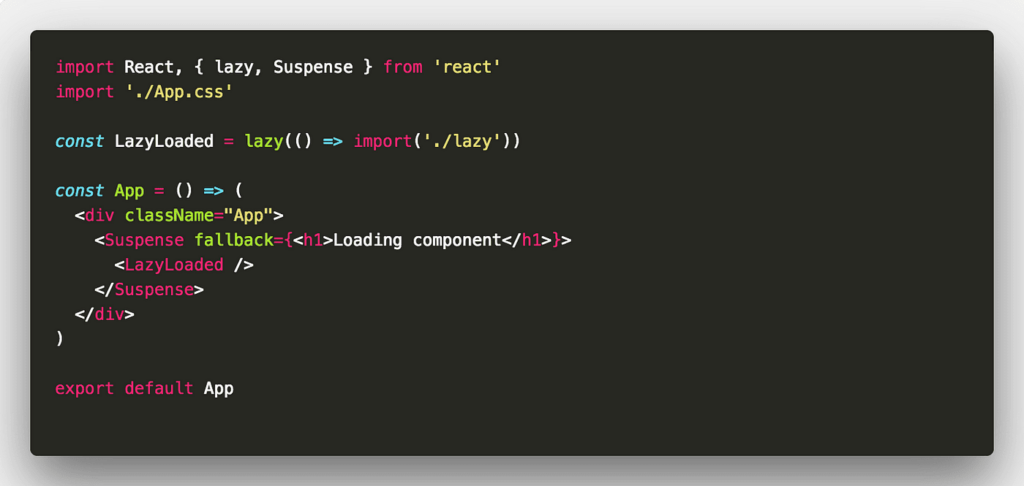
27. How does React handle forms and validation?
Answer:
React handles forms using controlled components, where the form’s input values are managed by the component’s state. Validation can be done by checking the form state on submit or by using libraries like Formik or React Hook Form. You can display error messages by conditionally rendering based on validation results.
Example:
const [name, setName] = useState(“”);
const [error, setError] = useState(“”);
const handleSubmit = () => {
if (name === “”) {
setError(“Name is required”);
} else {
setError(“”);
// Submit the form
}
};
return (
<form onSubmit={handleSubmit}>
<input value={name} onChange={(e) => setName(e.target.value)} />
{error && <span>{error}</span>}
<button type=”submit”>Submit</button>
</form>
);
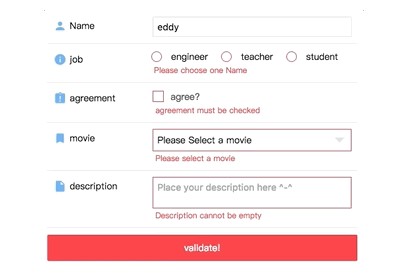
28. What are Hooks rules in React?
Answer:
The Rules of Hooks ensure that hooks are used correctly and consistently:
- Only call hooks at the top level of the component or inside other hooks (don’t call hooks inside loops, conditions, or nested functions).
- Only call hooks from React functional components or custom hooks. These rules are enforced to maintain consistency and avoid bugs in the component lifecycle.
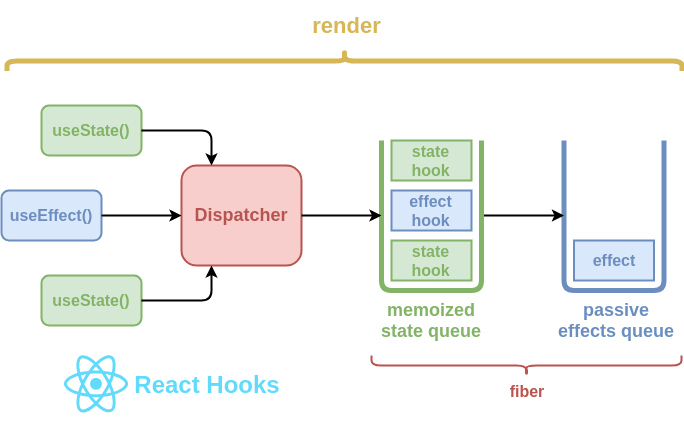
29. What is the purpose of use Imperative Handle?
Answer:
useImperativeHandle is a hook used with forwardRef to customize the instance value that is exposed to parent components when using ref. It allows you to expose specific methods or properties to the parent component instead of the entire component instance.
Example:
useImperativeHandle(ref, () => ({
focus: () => inputRef.current.focus(),
}));
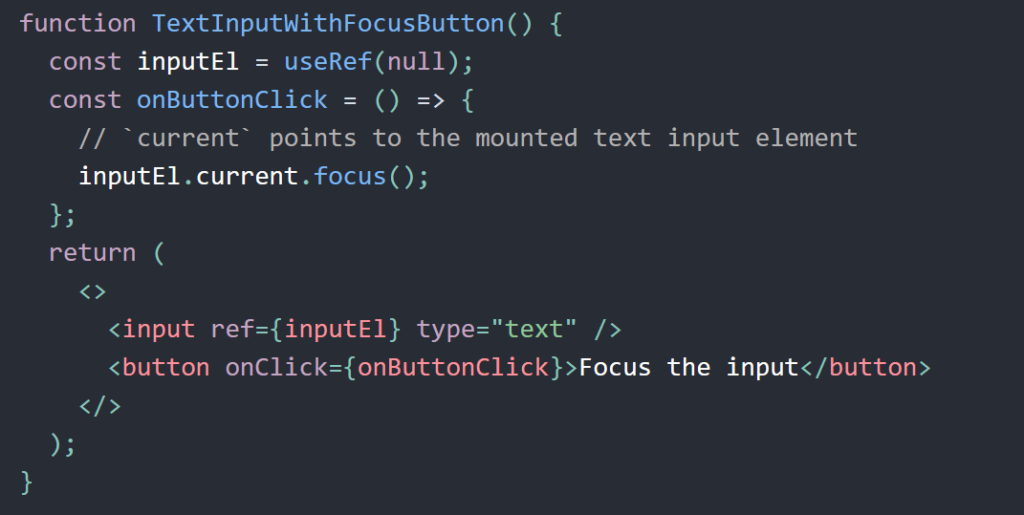
30. How do you pass data between sibling components in React?
Answer:
In React, sibling components cannot directly communicate with each other. However, you can pass data between siblings through their common parent. The parent can store the shared state and pass the data down to the children via props.
Example:
function Parent() {
const [sharedData, setSharedData] = useState(“Hello”);
return (
<>
<Child1 sharedData={sharedData} />
<Child2 setSharedData={setSharedData} />
</>
);
}
function Child1({ sharedData }) {
return <div>{sharedData}</div>;
}
function Child2({ setSharedData }) {
return <button onClick={() => setSharedData(“Updated Data”)}>Update</button>;
}
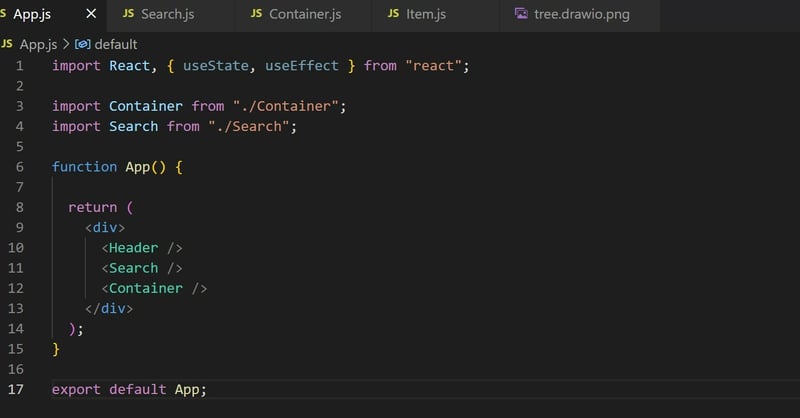
31. What are prop types in React?
Answer:
Prop types are used to validate the types of props that a component receives. This helps catch errors and ensure that components receive the expected types of data. You can define prop types using the prop-types library.
Example:
import PropTypes from ‘prop-types’;
const MyComponent = ({ name, age }) => {
return <div>{name} is {age} years old</div>;
};
MyComponent.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
};
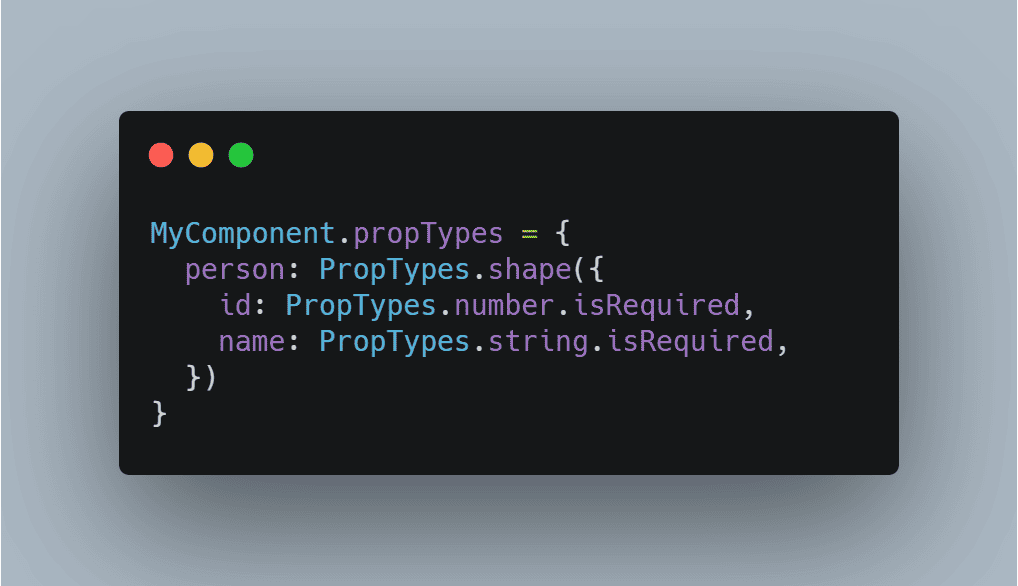
33. What is a higher-order component (HOC) in React?
Answer:
A higher-order component (HOC) is a function that takes a component and returns a new component with additional props or logic. HOCs are used for code reuse and are often used to add functionality such as authentication checks, logging, or data fetching to components.
Example:
function withAuth(Component) {
return function AuthComponent(props) {
if (!isAuthenticated) {
return <Redirect to=”/login” />;
}
return <Component {…props} />;
};
}
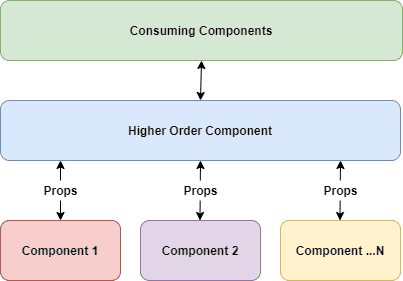
34. What is the Context API in React and how does it work?
Answer:
The Context API allows you to share values (such as global state or settings) between components without having to explicitly pass props at each level. It consists of a Provider component to provide values and a Consumer or useContext hook to access values.
Example:
const ThemeContext = React.createContext(‘light’);
function MyComponent() {
const theme = useContext(ThemeContext);
return <div>The theme is {theme}</div>;
}
function App() {
return (
<ThemeContext.Provider value=”dark”>
<MyComponent />
</ThemeContext.Provider>
);
}
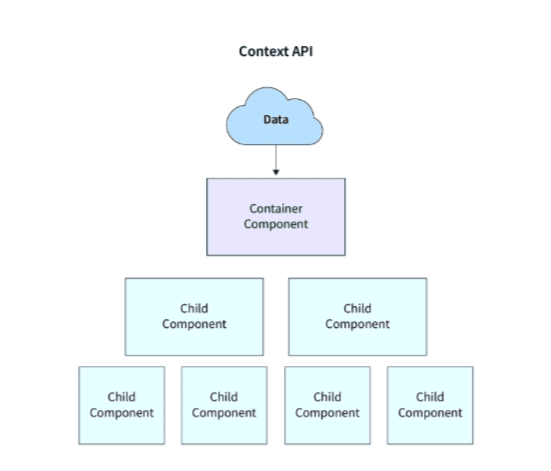
35. What is the use of the React.StrictMode wrapper in React?
Answer:
React.StrictMode is a wrapper component used in development mode to identify potential problems in an application. It helps detect unsafe lifecycle methods, deprecated APIs, and other issues. It does not affect production builds and is intended only for development.
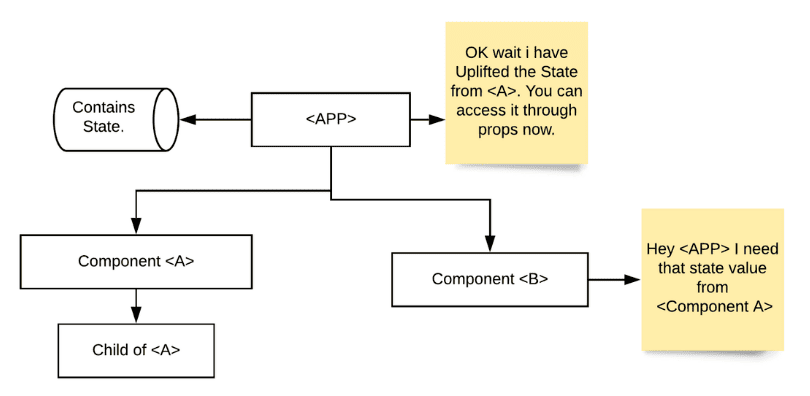
36. What is the role of keys in a list of elements in React?
Answer:
Keys help React identify which items in a list have changed, been added, or removed. They are essential for optimizing the re-rendering of lists, as React uses the keys to minimize the number of DOM updates and ensure that components are updated correctly.
Example:
const items = [‘apple’, ‘banana’, ‘orange’];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
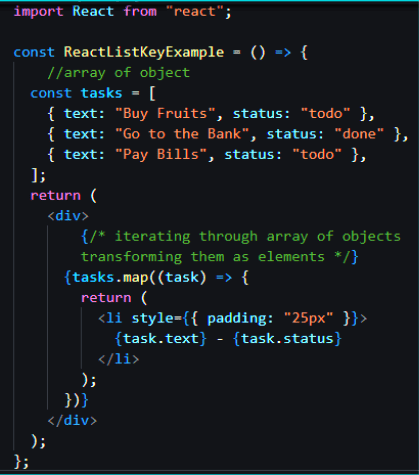
37. What is a controlled component in React?
Answer:
A controlled component is an element (such as an input or textarea) whose value is controlled by React’s state. The value of the input is bound to the component’s state, and any changes are handled through the onChange event to update the state.
Example:
const [value, setValue] = useState(”);
const handleChange = (e) => {
setValue(e.target.value);
};
return <input type=”text” value={value} onChange={handleChange} />;
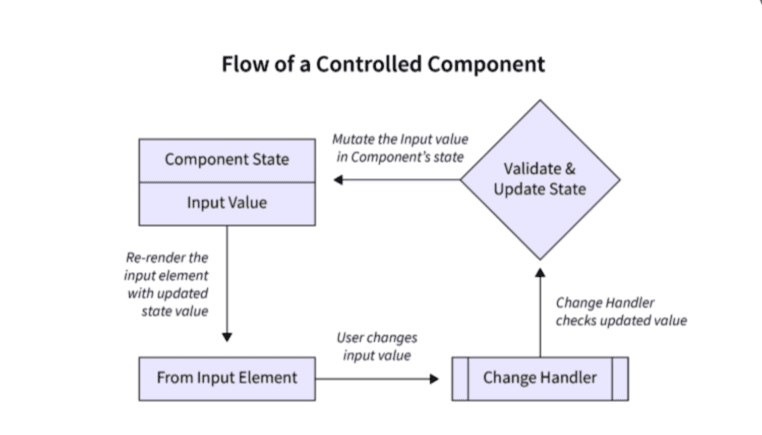
38. What is the use of the useContext hook in React?
Answer:
The useContext hook is used to access the current value of a Context in a functional component. It allows you to avoid passing props manually through multiple layers of components, simplifying data sharing in the component tree.
Example:
const UserContext = React.createContext();
function Component() {
const user = useContext(UserContext);
return <div>{user.name}</div>;
}
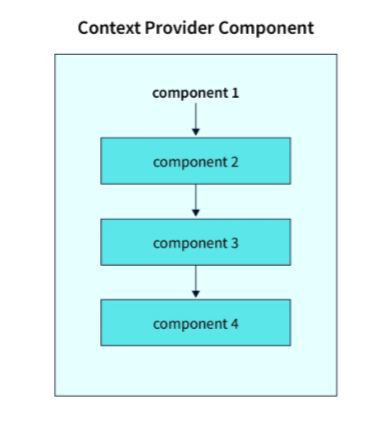
39. What are the benefits of using TypeScript with React?
Answer:
TypeScript adds static type checking to React applications, helping catch bugs early in the development process. It provides better editor support, autocompletion, and refactoring tools. It also helps with better documentation and understanding of the code, making the development process more reliable and efficient.
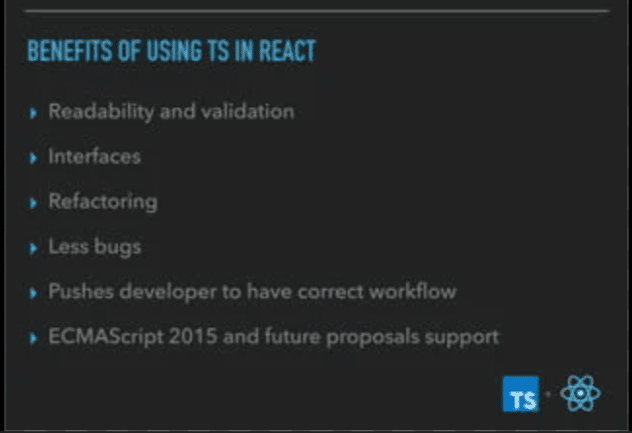
40. What is the purpose of React DevTools?
Answer:
React DevTools is a browser extension that allows you to inspect and debug React applications. It provides a UI for inspecting the component tree, props, and state of components, and also includes features like performance profiling and debugging hooks.
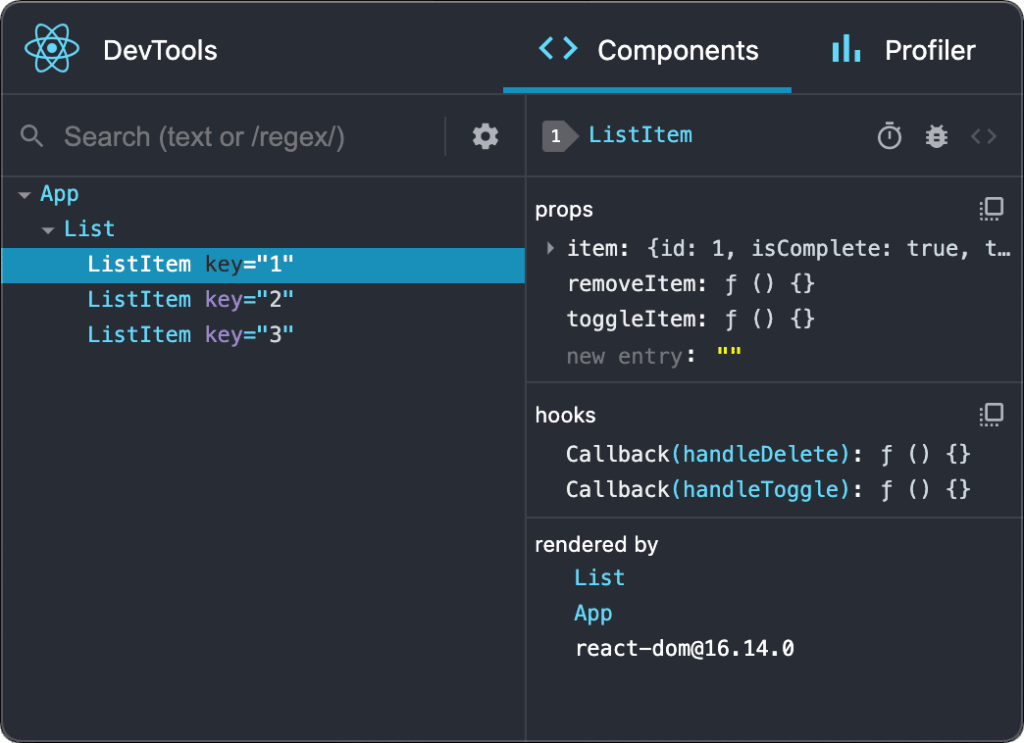
41. What are React's lifecycle methods and how do they differ between class components and functional components?
Answer:
- Class components: React lifecycle methods include componentDidMount, componentDidUpdate, componentWillUnmount, etc. These methods are used for side-effects like data fetching, DOM manipulation, etc.
- Functional components: With the introduction of hooks, functional components use useEffect to handle side effects that would typically be handled using lifecycle methods in class components. Based on the dependency array, the useEffect hook can mimic componentDidMount, componentDidUpdate, and componentWillUnmount.
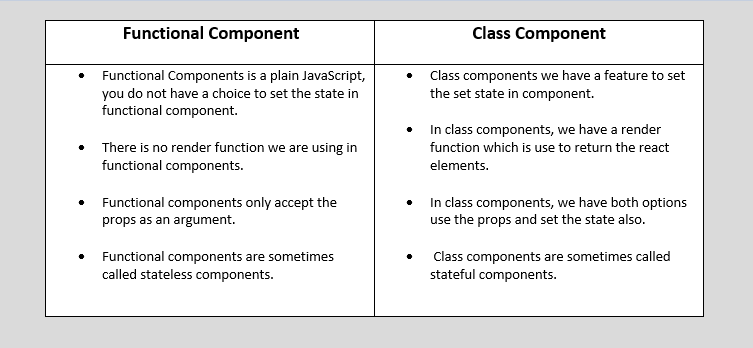
42. What is React.memo() and how does it work?
Answer:
React.memo() is a higher-order component that memoizes a component, preventing unnecessary re-renders. It only re-renders the component if its props change. This is useful for functional components that render the same output for the same input, optimizing performance.
Example:
const MyComponent = React.memo((props) => {
return <div>{props.value}</div>;
});
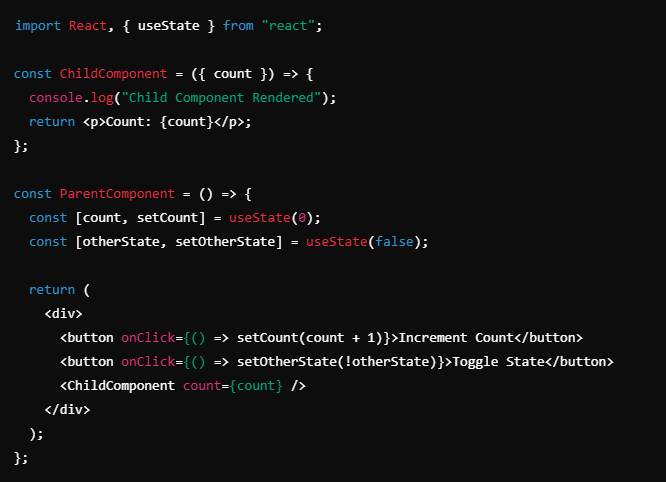
43. What is the difference between useState and useReducer in React?
Answer:
- useState: Simple state management for handling primitive values or simple data structures. It is suitable for smaller state logic.
- useReducer: More suitable for complex state logic that involves multiple sub-values or when the next state depends on the previous one. It provides a more centralized and predictable state management mechanism.
Example of useReducer:
const initialState = { count: 0 };
const reducer = (state, action) => {
switch (action.type) {
case ‘increment’:
return { count: state.count + 1 };
case ‘decrement’:
return { count: state.count – 1 };
default:
return state;
}
};
const [state, dispatch] = useReducer(reducer, initialState);
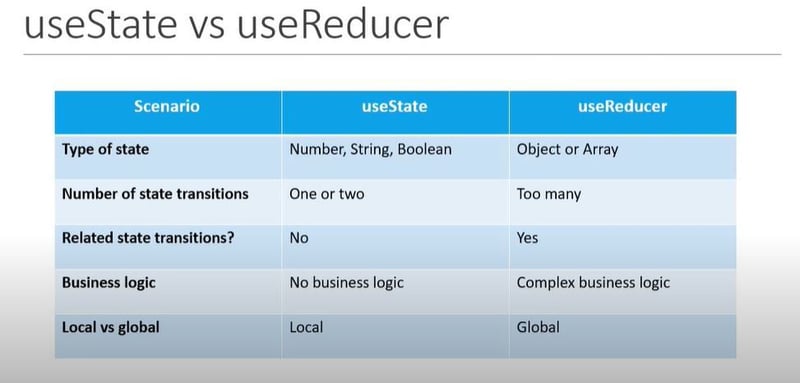
44. What is the purpose of forwardRef in React?
Answer:
forwardRef is a higher-order component that allows you to pass a ref from a parent component to a child component. It is useful when you want to allow the parent to directly access a DOM node or a child component instance.
Example:
const MyComponent = React.forwardRef((props, ref) => {
return <input ref={ref} />;
});
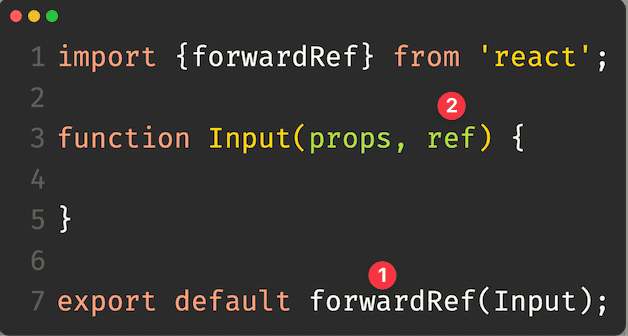
45. How do you handle errors in React components?
Answer:
Errors in React components can be handled using Error Boundaries. These are components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI.
Example:
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
console.log(error, errorInfo);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
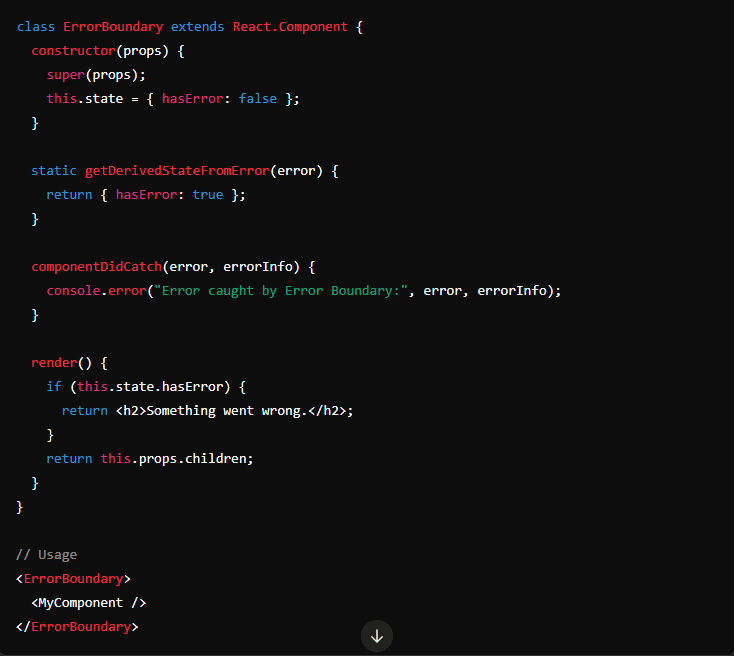
46. What is code splitting in React and how do you implement it?
Answer:
Code splitting is the practice of splitting the bundle into smaller files to improve the loading time of the application. In React, code splitting can be implemented using React.lazy and Suspense for dynamic imports of components.
Example:
const LazyComponent = React.lazy(() => import(‘./LazyComponent’));
return (
<Suspense fallback={<div>Loading…</div>}>
<LazyComponent />
</Suspense>
);
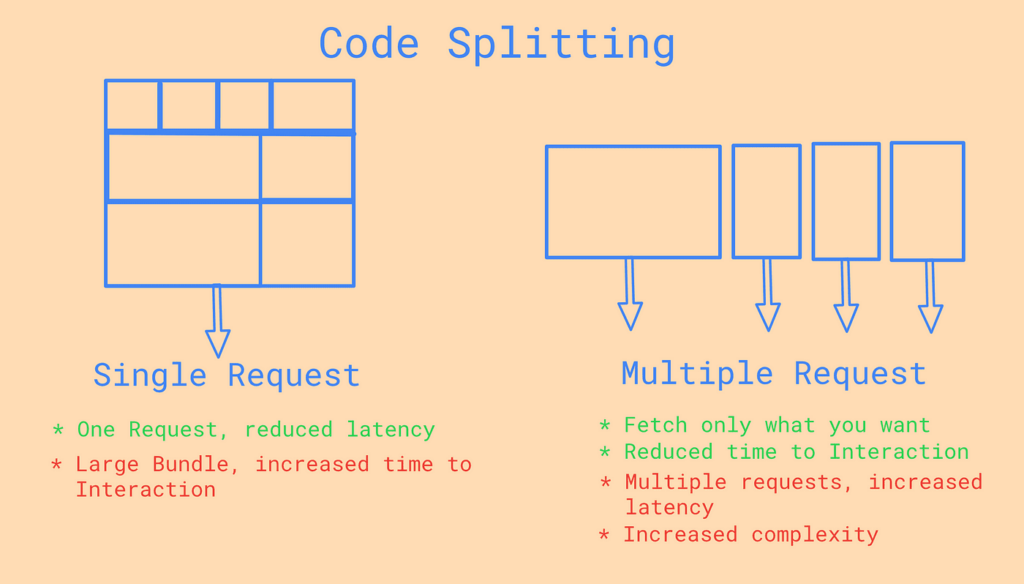
47. What is the useRef hook used for in React?
Answer:
The useRef hook is used to persist a mutable reference to a DOM element or a value across renders. It doesn’t cause re-rendering when its value changes. It is commonly used for accessing DOM elements or storing values that don’t need to trigger re-renders.
Example:
const inputRef = useRef(null);
const focusInput = () => {
inputRef.current.focus();
};
return <input ref={inputRef} />;
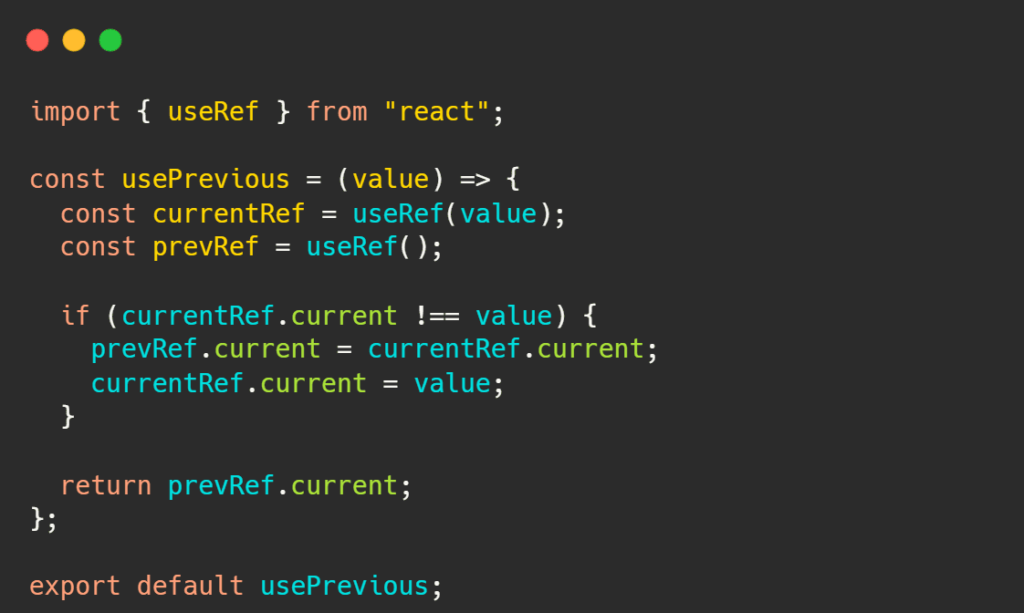
48. What is useCallback and how does it differ from useMemo?
Answer:
- useCallback: Memoizes a function so that it is not recreated on every render unless its dependencies change. It’s useful when passing functions as props to avoid unnecessary re-renders.
- useMemo: Memoizes a value or calculation, preventing it from being recalculated on every render unless its dependencies change. It’s useful when performing expensive calculations.
Example:
const memoizedCallback = useCallback(() => {
// function logic
}, [dependencies]);
const memoizedValue = useMemo(() => expensiveComputation(), [dependencies]);
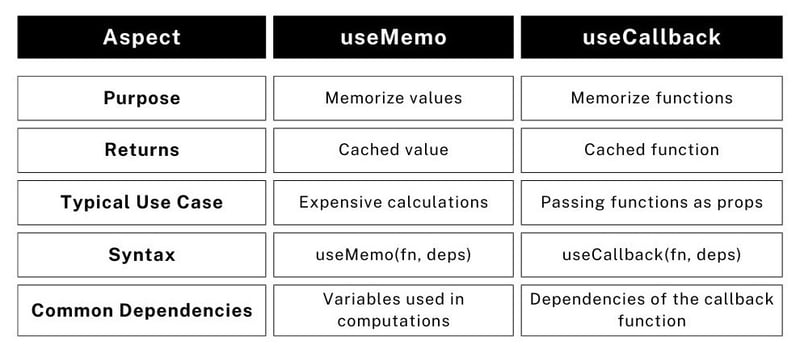
49. What are controlled vs uncontrolled components in React?
Answer:
- Controlled components: Their values are controlled by React state. Any change to the input value updates the state and causes a re-render.
- Uncontrolled components: Their values are controlled by the DOM itself, and React only interacts with them via ref rather than the state.
Example of a controlled component:
const [value, setValue] = useState(”);
const handleChange = (e) => setValue(e.target.value);
return <input value={value} onChange={handleChange} />;
Example of an uncontrolled component:
const inputRef = useRef(null);
const handleSubmit = () => {
console.log(inputRef.current.value);
};
return <input ref={inputRef} />;
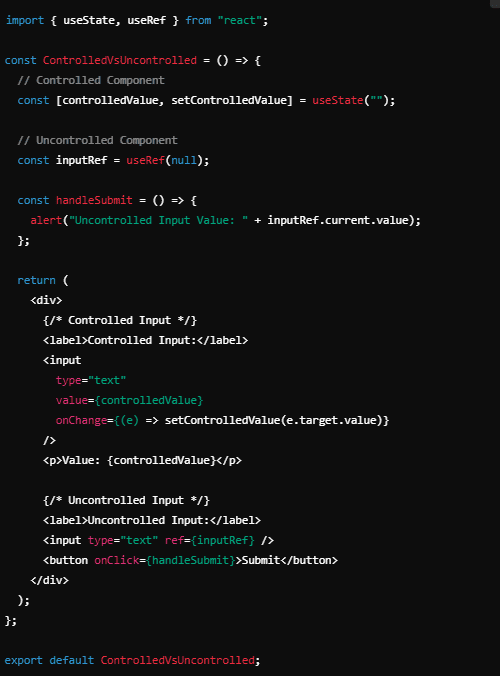
50. What is the difference between React.Fragment and (short syntax)?
Answer:
React.Fragment and <></> (empty tags) are used for grouping multiple elements without adding extra nodes to the DOM. They function identically, but <></> is the short syntax introduced in React 16.2 to avoid the need to explicitly write React.Fragment.
Example:
// Using React.Fragment
return (
<React.Fragment>
<h1>Title</h1>
<p>Content</p>
</React.Fragment>
);
// Using short syntax
return (
<>
<h1>Title</h1>
<p>Content</p>
</>
);
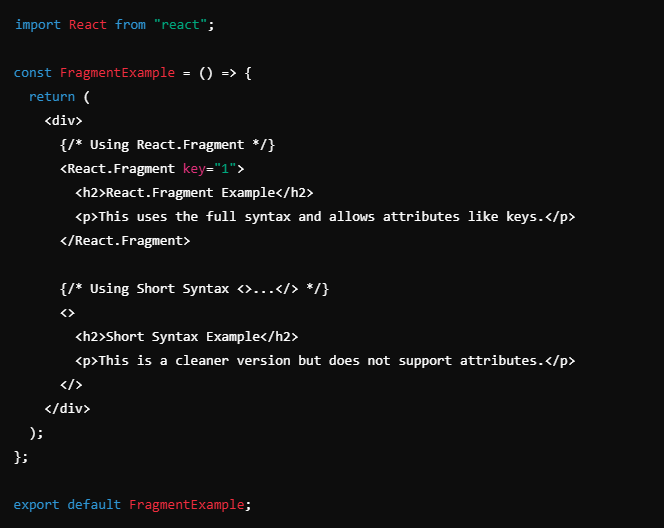
Master React JS Interview Questions and Answers – Get Expert Guidance and Crack Your Interview with Brolly Academy!
Learn From Our React js Expert Trainer
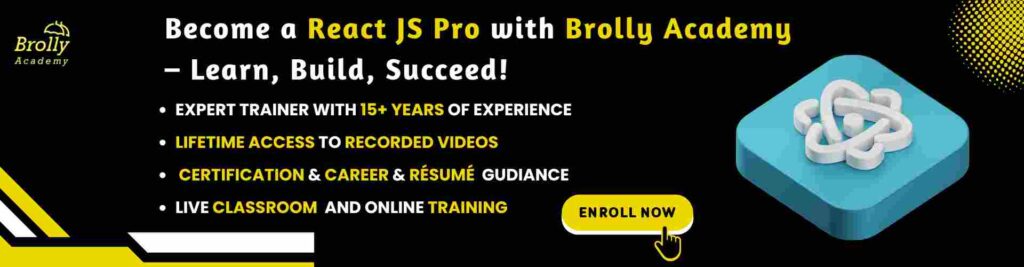
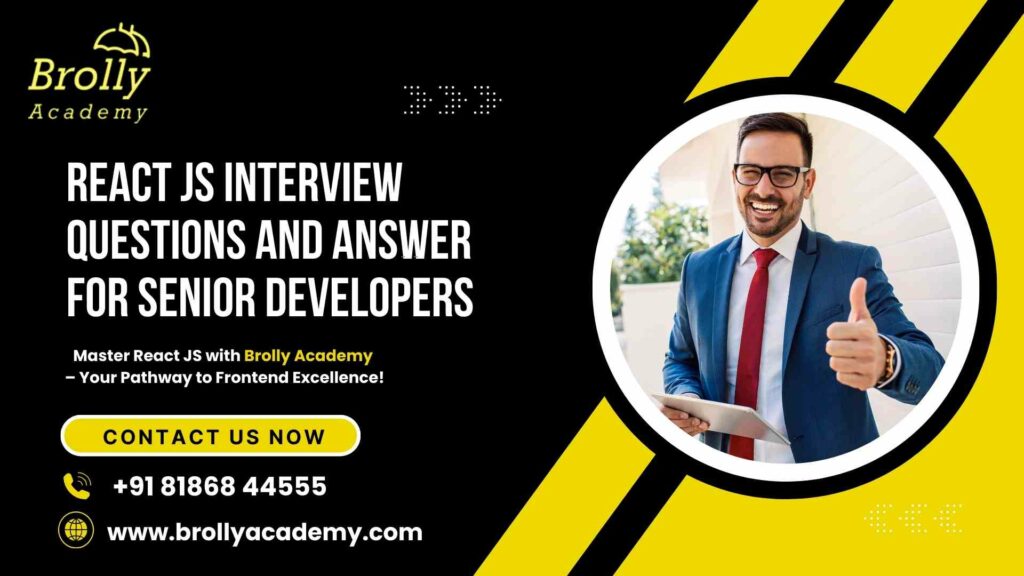
Advanced React js Interview Questions And Answer For Senior developer
1. What is React's reconciliation algorithm, and how does it improve performance?
Answer:
React uses a reconciliation algorithm (also called “diffing”) to efficiently update the DOM. It compares the virtual DOM with the real DOM and applies only the necessary changes, minimizing the number of updates and optimizing performance.
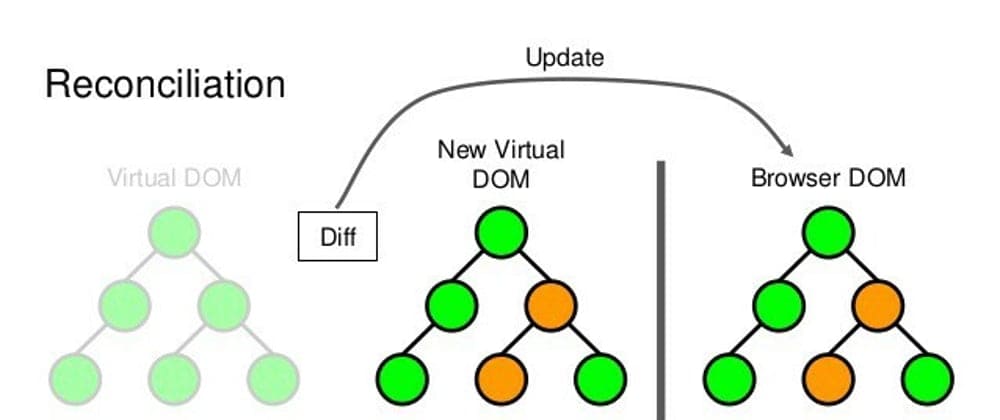
2. How does React handle updates in a concurrent mode?
Answer:
Concurrent Mode in React allows rendering to be interruptible, improving app performance and responsiveness. It allows React to pause work and come back to it later, prioritizing more important updates. React can switch between tasks and ensures smooth user experiences even with heavy UI changes.
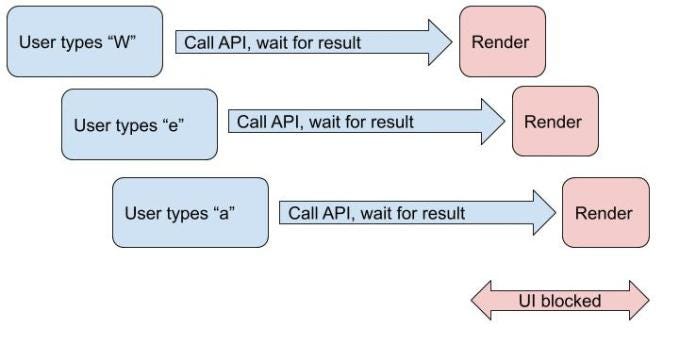
3. What is the difference between React.createElement() and JSX?
Answer:
JSX is syntactic sugar for React.createElement(). JSX allows us to write HTML-like code in React components, which is then compiled to React.createElement() calls that create JavaScript objects representing the UI elements.
Example:
// JSX
<h1>Hello</h1>;
// Equivalent with React.createElement
React.createElement(‘h1’, null, ‘Hello’);
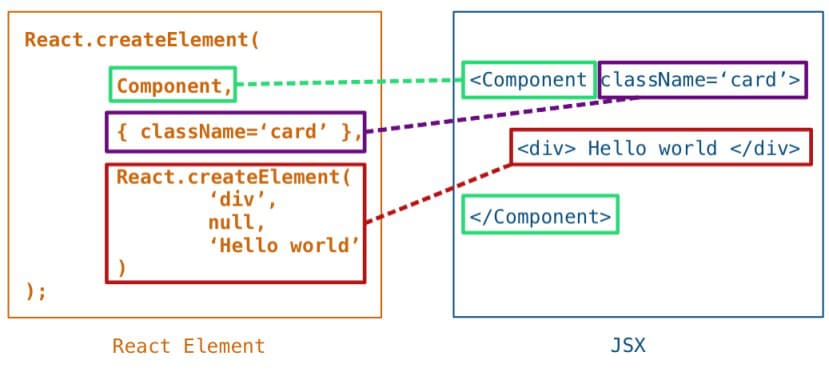
4. What are the potential issues with React's Context API, and how can you mitigate them?
Answer:
The main issues with the Context API are unnecessary re-renders of consumers when the context value changes and scalability concerns. To mitigate this:
- Keep the context values minimal and stable.
- Split the context into smaller contexts if necessary.
- Use memoization techniques such as React.memo and useMemo to optimize rendering.
5. How does React handle event delegation, and why is it important?
Answer:
React uses event delegation to handle events efficiently. Instead of attaching event listeners to each individual element, React attaches a single event listener to the root of the component tree (the root node). When an event occurs, it bubbles up the DOM, and React checks if the event target matches the desired element.
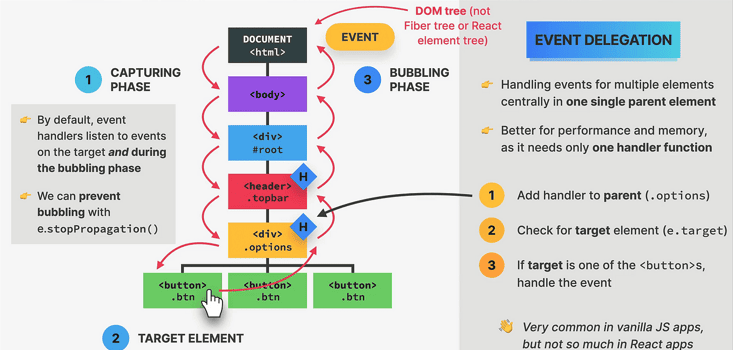
6. What is the significance of the key prop in React, and what are the consequences of using non-unique keys?
Answer:
The key prop is used by React to identify elements in a list, helping React efficiently update and reconcile the DOM. Non-unique or incorrect keys can lead to unexpected rendering issues, such as improper component reordering, and negatively impact performance.
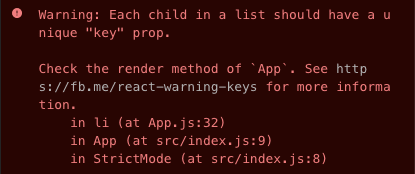
7. What are the pros and cons of using class components vs. functional components in React?
Answer:
- Class components: Provide full access to React’s lifecycle methods, but they are more verbose and can be harder to manage with increasing complexity.
- Functional components: Easier to write, test, and manage, especially with hooks. However, they did not have lifecycle methods until the introduction of hooks.
Since the introduction of hooks, functional components are more commonly used.
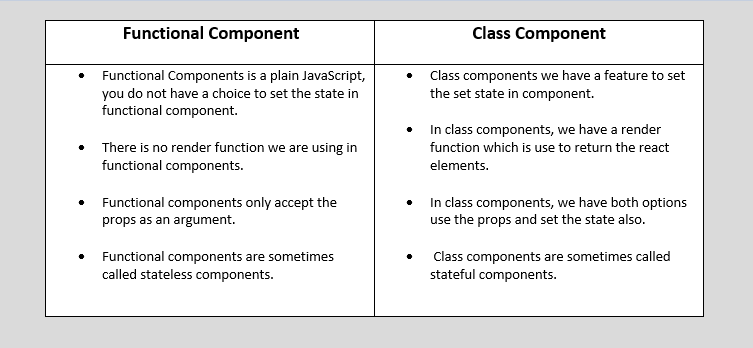
8. What is the purpose of React.StrictMode, and how does it work?
Answer:
React.StrictMode is a development tool to highlight potential problems in the application, such as unsafe lifecycle methods, deprecated APIs, and side effects in render functions. It helps developers identify issues before they become bugs in production.
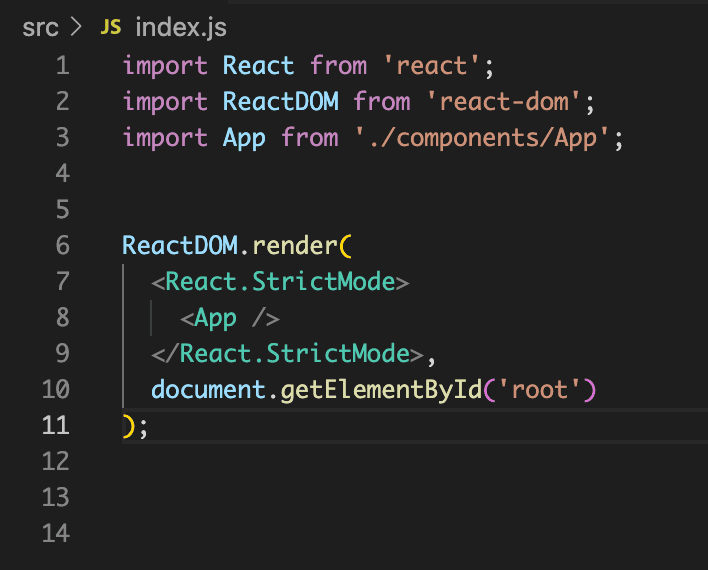
9. Explain how server-side rendering (SSR) works in React, and what are the challenges associated with it?
Answer:
SSR is the process of rendering React components on the server and sending the HTML to the client, improving the initial page load time and SEO. Challenges include handling client-specific functionality like event listeners and state management, and optimizing performance on both the server and client sides.
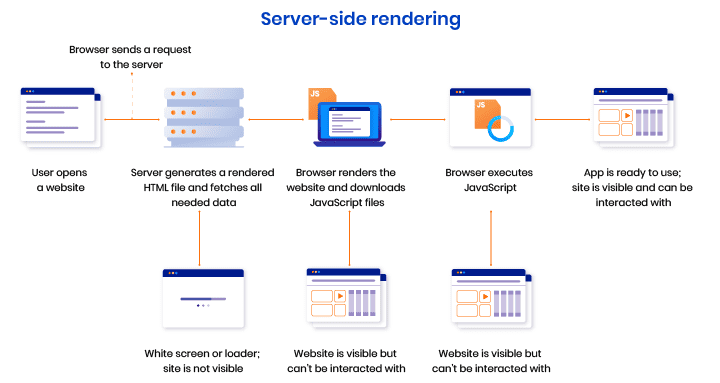
10. What is the significance of the React fiber architecture introduced in React 16?
Answer:
The Fiber architecture is React’s new reconciliation algorithm that improves rendering performance and enables features like concurrent rendering, time-slicing, and better handling of animations and transitions. It makes React more flexible in terms of prioritizing and interrupting work.
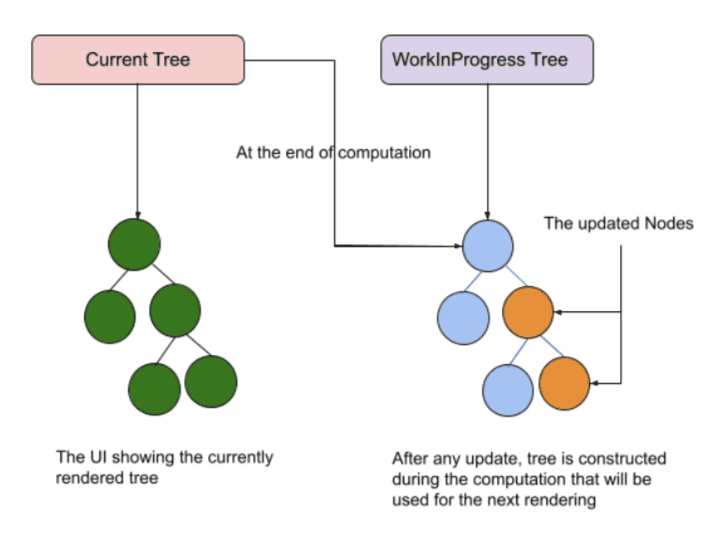
Learn From Our React js Expert Trainer
11. How would you implement a custom hook in React, and when would you use one?
Answer:
A custom hook is a JavaScript function that uses React hooks to encapsulate reusable logic across multiple components. Custom hooks are used when you need to extract component logic into reusable functions.
Example:
function useWindowWidth() {
const [width, setWidth] = useState(window.innerWidth);
useEffect(() => {
const handleResize = () => setWidth(window.innerWidth);
window.addEventListener(‘resize’, handleResize);
return () => window.removeEventListener(‘resize’, handleResize);
}, []);
return width;
}
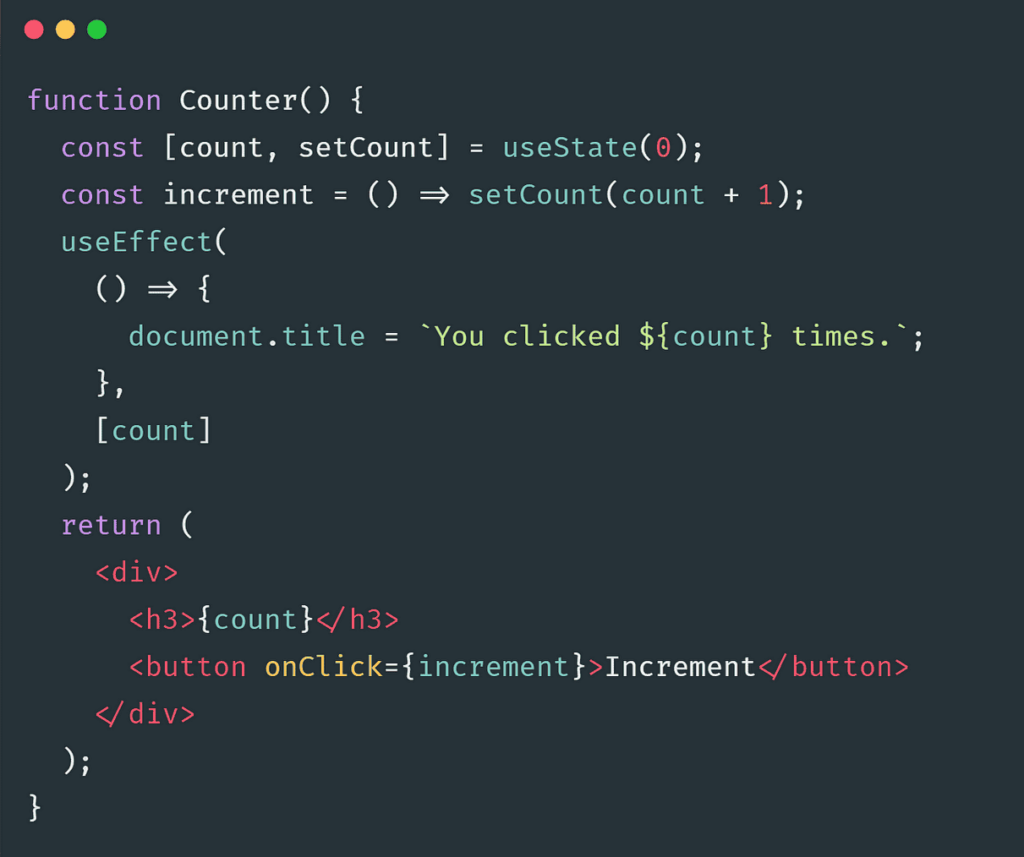
12. How do you optimize React performance in a large-scale application?
Answer:
- Code splitting using React.lazy and Suspense.
- Memoization with useMemo and useCallback to avoid unnecessary re-renders.
- React.memo to memoize functional components.
- Lazy loading of components and routes.
- Avoiding inline functions and objects in the render method.
- Virtualization for large lists or tables.
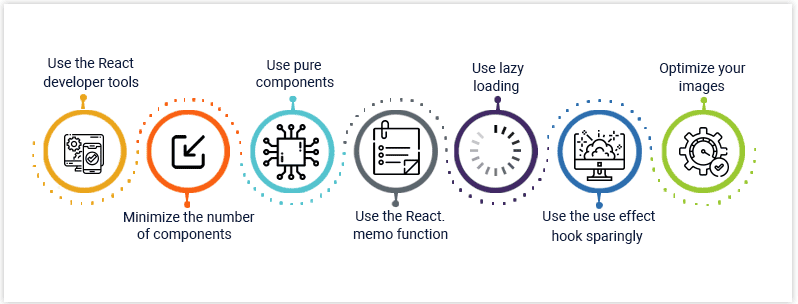
13. What are higher-order components (HOCs) in React, and how are they different from render props?
Answer:
- HOCs: Functions that take a component and return a new component with additional functionality or props. Common use cases include adding authentication, logging, etc.
- Render props: A pattern where a component receives a function as a prop and calls it to render content.
- The main difference is that HOCs wrap components, while render props pass functions for rendering.
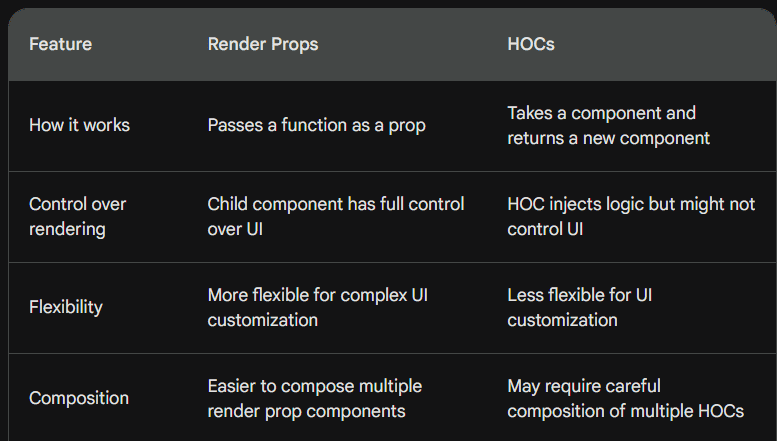
14. What is the useCallback hook, and how does it differ from useMemo?
Answer:
- useCallback: Memoizes a function and ensures it is not recreated on every render unless its dependencies change. It’s useful when passing callbacks to child components to prevent unnecessary re-renders.
- useMemo: Memoizes the result of an expensive calculation and ensures it is recomputed only when its dependencies change
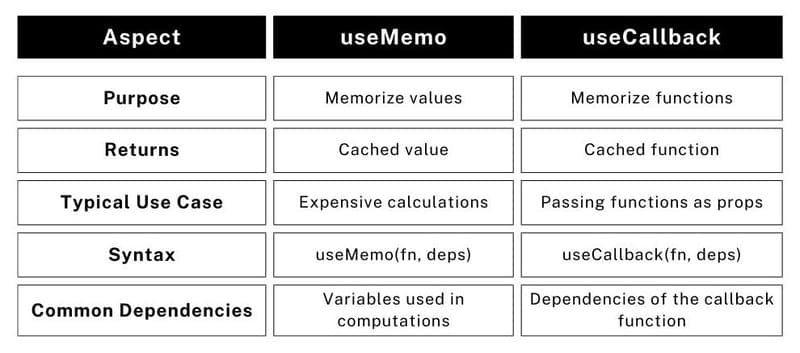
15. How would you manage global state in a React application?
Answer:
Global state in React can be managed using:
- React Context API for simpler state management.
- State management libraries like Redux, MobX, or Zustand for more complex global state needs.
- useReducer for more advanced state management with complex logic.
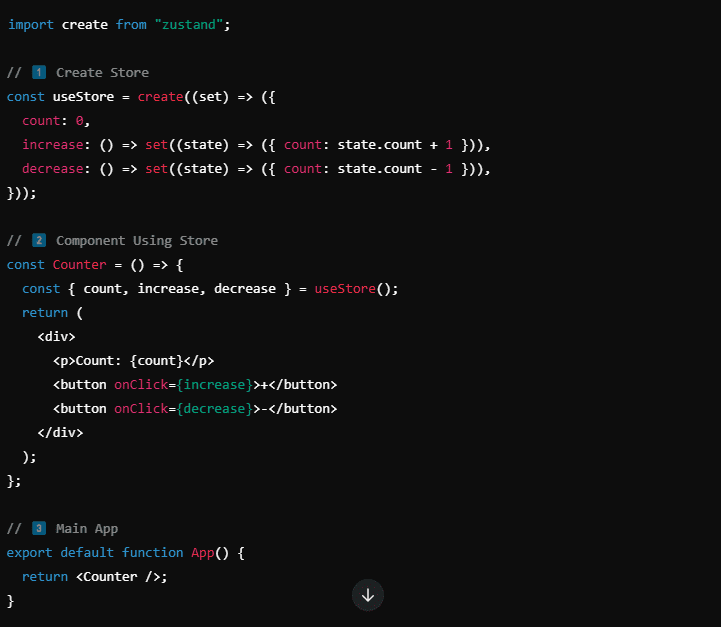
16. What is the purpose of useImperativeHandle, and when should it be used?
Answer:
useImperativeHandle is used with forwardRef to expose specific instance methods or properties from a child component to a parent component. It allows controlling what part of a component’s API is exposed to the parent.
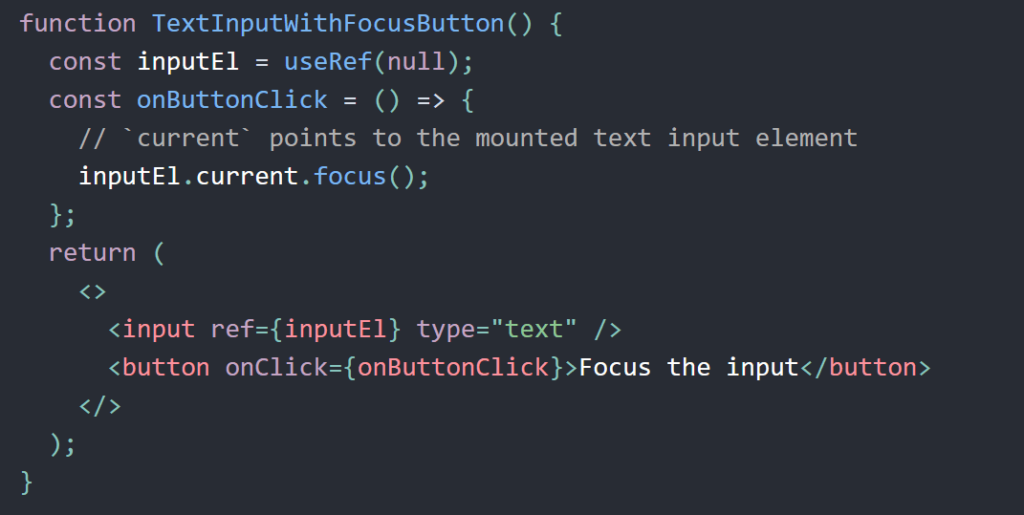
17. What are render props, and how do they work in React?
Answer:
Render props is a pattern where a component passes a function as a prop, and the function is used to dynamically render the component’s content. This allows for more flexible component compositions.
Example:
function MouseTracker({ render }) {
const [x, y] = useMousePosition();
return <div>{render({ x, y })}</div>;
}
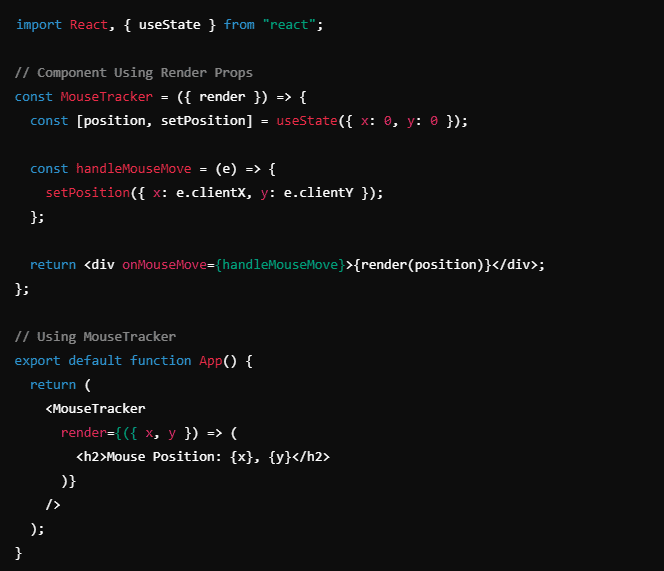
18. What is the significance of React's useLayoutEffect hook?
Answer:
useLayoutEffect runs synchronously after all DOM mutations but before the browser paints the screen. It is useful for measuring or manipulating the DOM before the user sees the changes, such as handling layout-related logic (e.g., measuring elements, triggering animations).
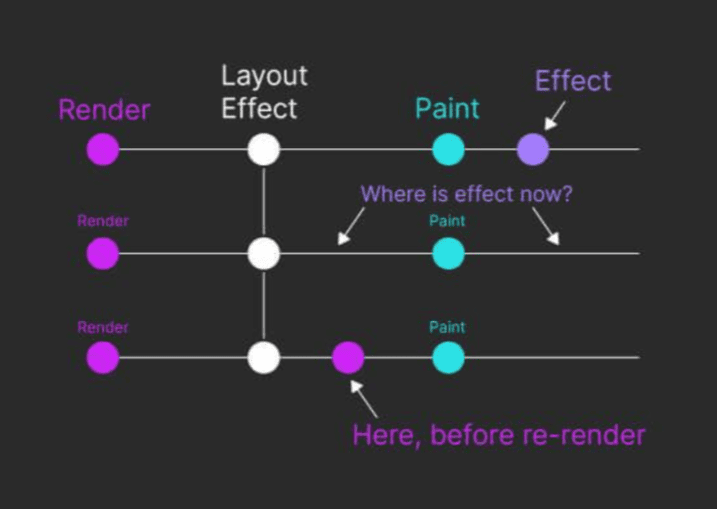
19. How does React's shouldComponentUpdate method work, and how is it different from React.memo?
Answer:
shouldComponentUpdate is a lifecycle method in class components that allows you to control whether a component should re-render when its state or props change. React.memo is a higher-order component used for functional components to optimize re-renders by memoizing the component and only re-rendering when props change.
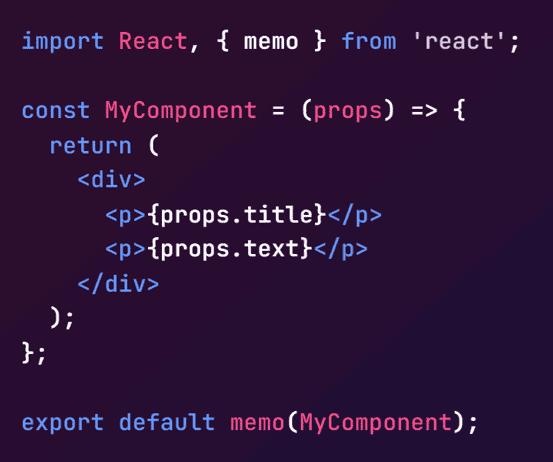
20. How would you handle forms in React with complex validation and conditional rendering?
Answer:
Complex forms can be handled by:
- State management using useState or useReducer to manage form values.
- Formik or React Hook Form for handling validation, form submission, and state management.
- Conditional rendering for showing validation messages or form sections based on user input or state.
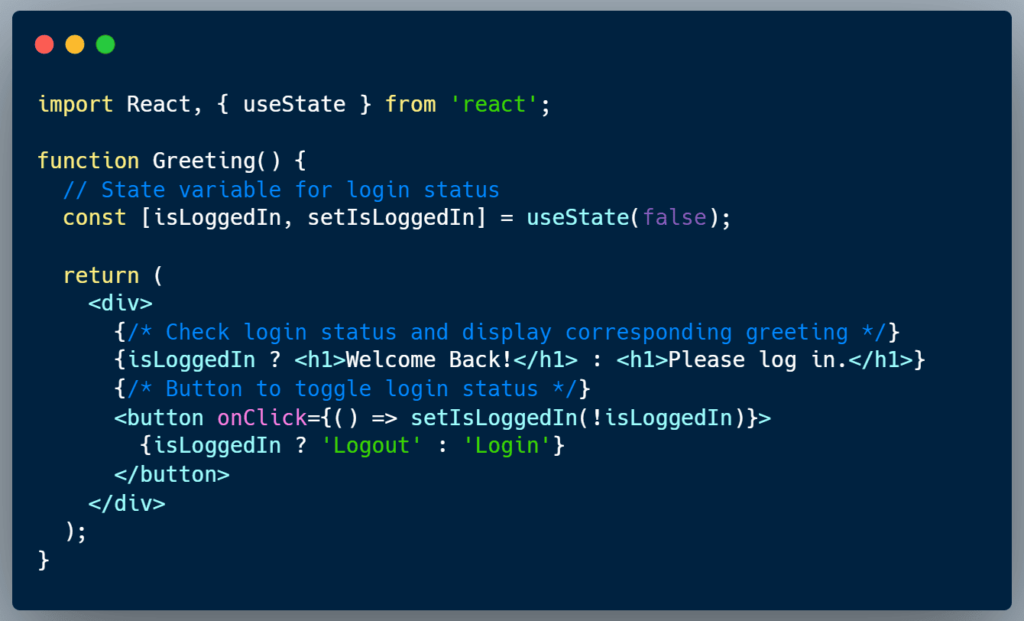
Learn From Our React js Expert Trainer
21. What is the concept of the "virtual DOM," and how does React use it to optimize performance?
Answer:
The virtual DOM is an in-memory representation of the actual DOM. React uses the virtual DOM to efficiently update the real DOM by performing a “diffing” algorithm that calculates the minimal set of changes needed to update the UI.
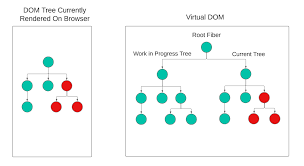
22. What are the potential performance bottlenecks when using React in large-scale applications?
Answer:
Potential bottlenecks include:
- Excessive re-renders due to improper use of keys, state updates, or functions passed as props.
- Large component trees without proper memoization or optimization techniques.
- Inefficient state management leading to unnecessary renders.
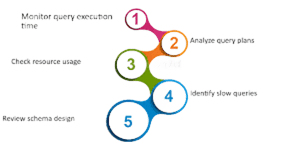
23. What is the React.lazy() function, and how does it work?
Answer:
React.lazy() allows you to dynamically import components only when they are needed, improving initial load performance. It works in combination with Suspense to display a loading state while the component is being loaded.
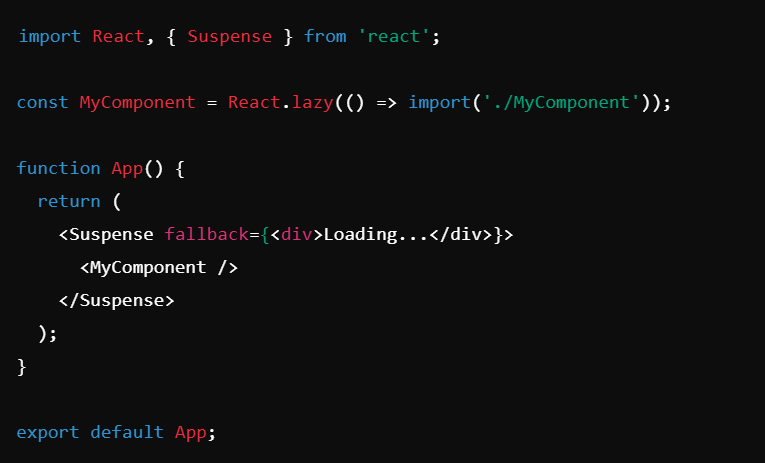
24. How does React’s useEffect hook handle cleanup, and what are the scenarios in which cleanup is necessary?
Answer:
Cleanup in useEffect is done by returning a function from the effect, which React will call when the component unmounts or before the effect re-runs. Cleanup is necessary when there are side-effects like subscriptions, timers, or event listeners.
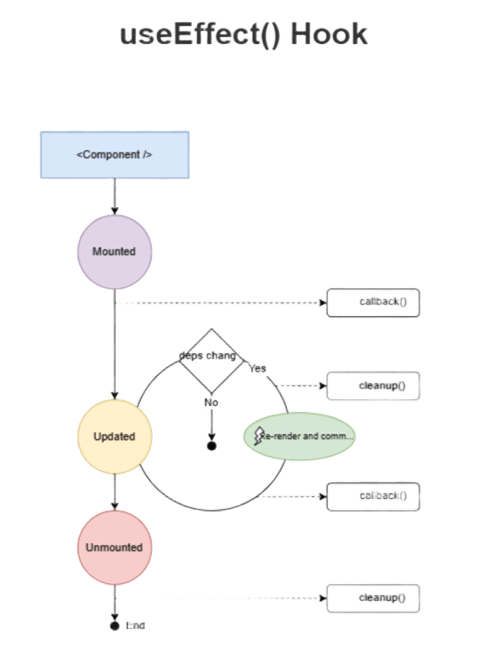
25. How do you handle side-effects in React components, and what hooks do you use?
Answer:
Side-effects in React components are handled using the useEffect hook. useEffect is used for tasks like data fetching, subscriptions, and manually changing the DOM.
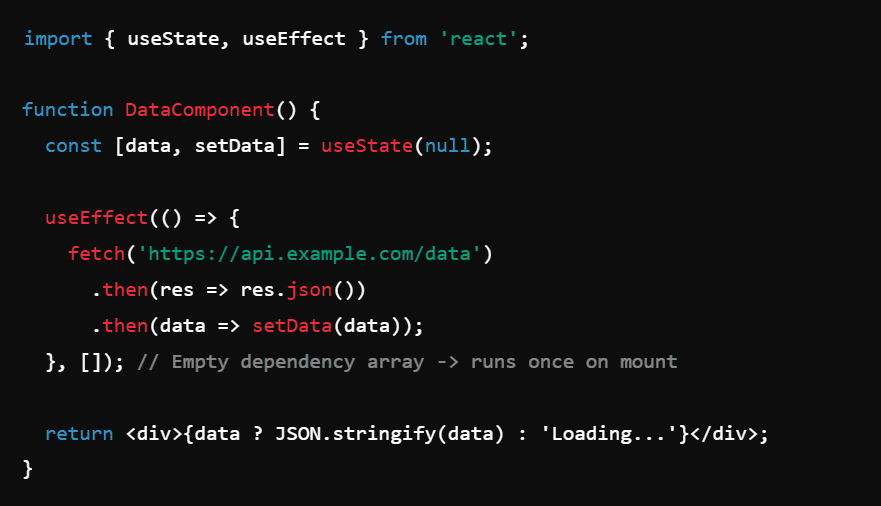
26. What is the difference between useEffect and useLayoutEffect?
Answer:
- useEffect: Runs asynchronously after the paint (render) process.
- useLayoutEffect: Runs synchronously before the paint, allowing you to make DOM changes or measurements before the user sees the changes.
- useLayoutEffect: Runs synchronously before the paint, allowing you to make DOM changes or measurements before the user sees the changes.
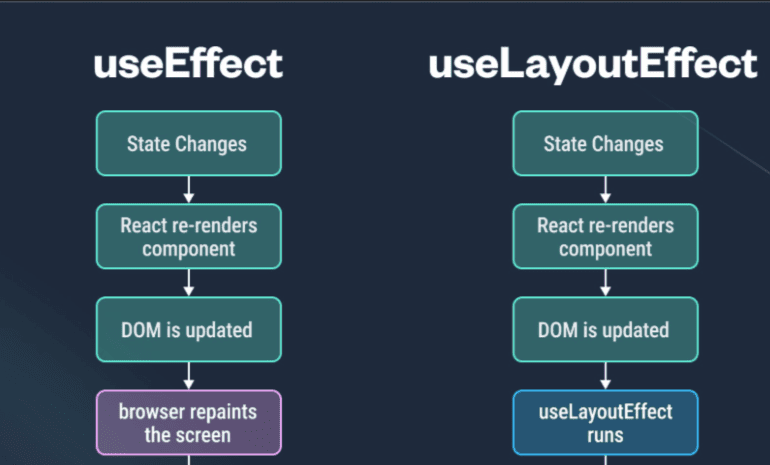
27. How do you optimize React code splitting in large-scale applications?
Answer:
Code splitting can be optimized by using dynamic imports, React.lazy(), and Suspense for components that are not needed immediately. Also, splitting routes and using Webpack for optimizing chunk sizes and lazy-loading specific modules can improve performance.
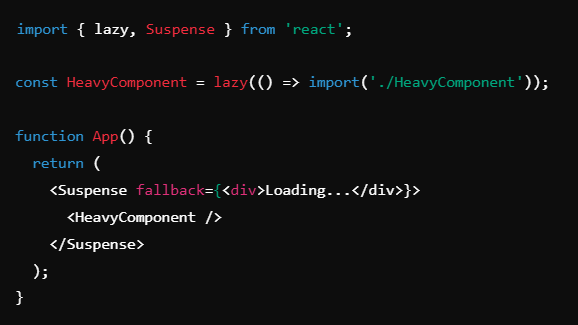
28. What are the best practices for managing side-effects in React?
Answer:
Best practices include:
- Use useEffect for side-effects.
- Ensure side-effects are not run on every render by providing a dependency array.
- Clean up side-effects using the cleanup function of useEffect.
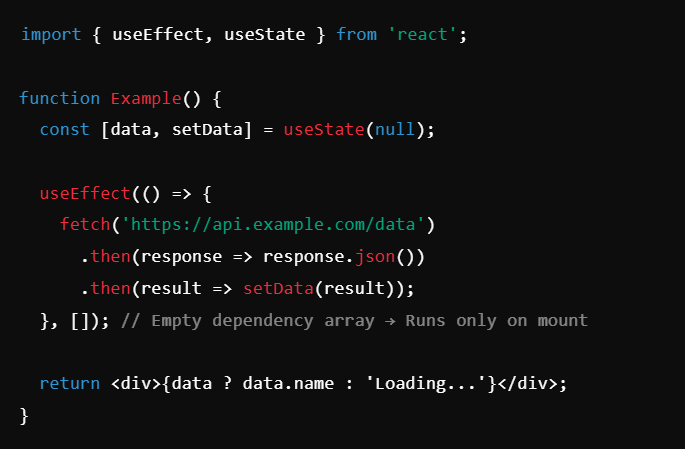
29. What is the significance of useRef in optimizing performance?
Answer:
useRef allows you to persist values across renders without triggering re-renders. It’s useful for accessing DOM nodes, saving values that need to be shared across
renders without affecting performance, and avoiding unnecessary re-renders.
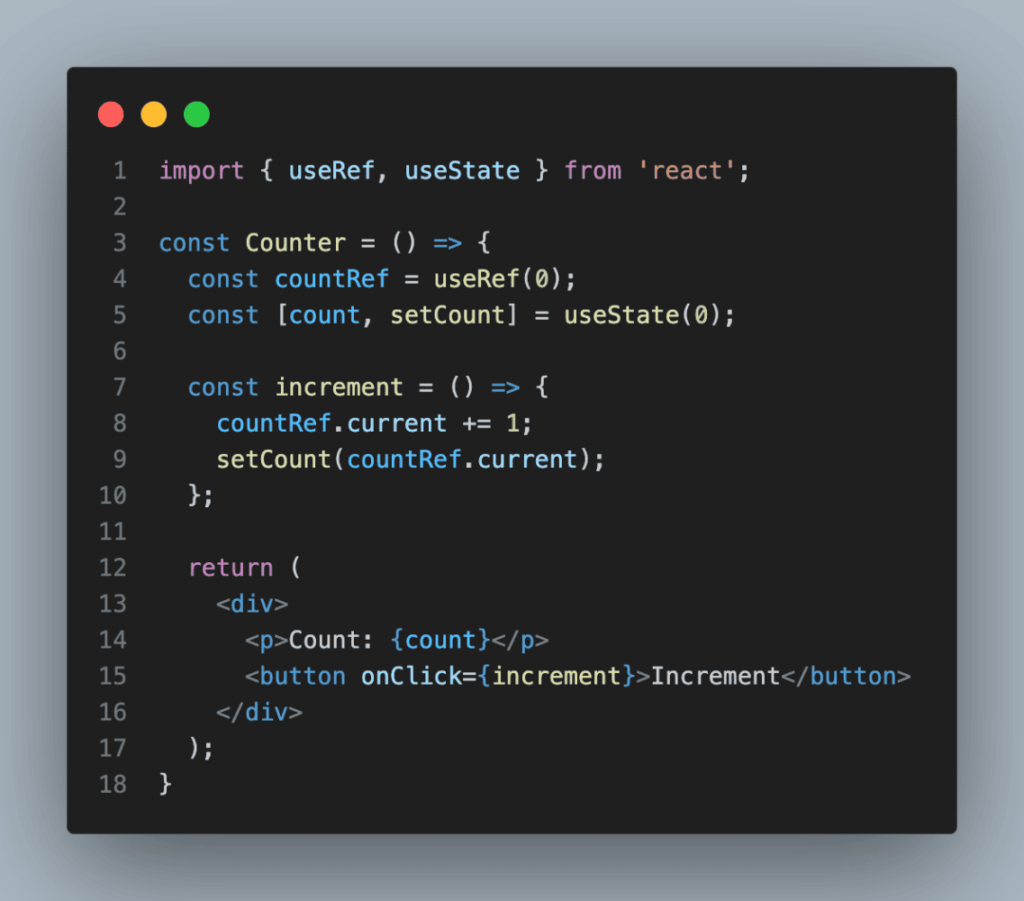
30. What is the role of the key prop in React's reconciliation algorithm?
Answer:
The key prop is used to identify elements in a list and ensure that React can efficiently update the list by comparing previous and new virtual DOM states. It helps React decide which elements to re-render and which to preserve, optimizing performance.
These questions and answers should give you a comprehensive understanding of expert-level React concepts!
Here are 20 more expert-level React interview questions:
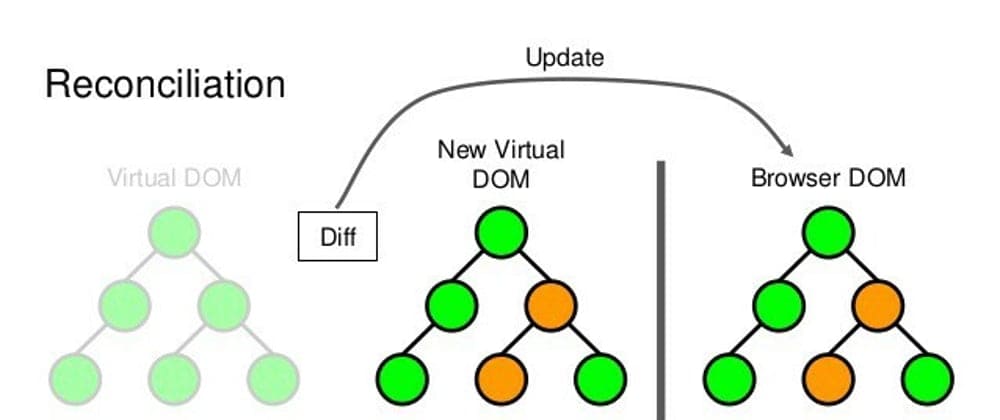
Learn From Our React js Expert Trainer
31. What is React's Context API, and how would you implement it for managing global state in large applications?
Answer:
The React Context API allows for managing and passing state throughout the component tree without prop drilling. For large applications, the Context API can be used in conjunction with useReducer or state management libraries like Redux to efficiently handle complex state logic.
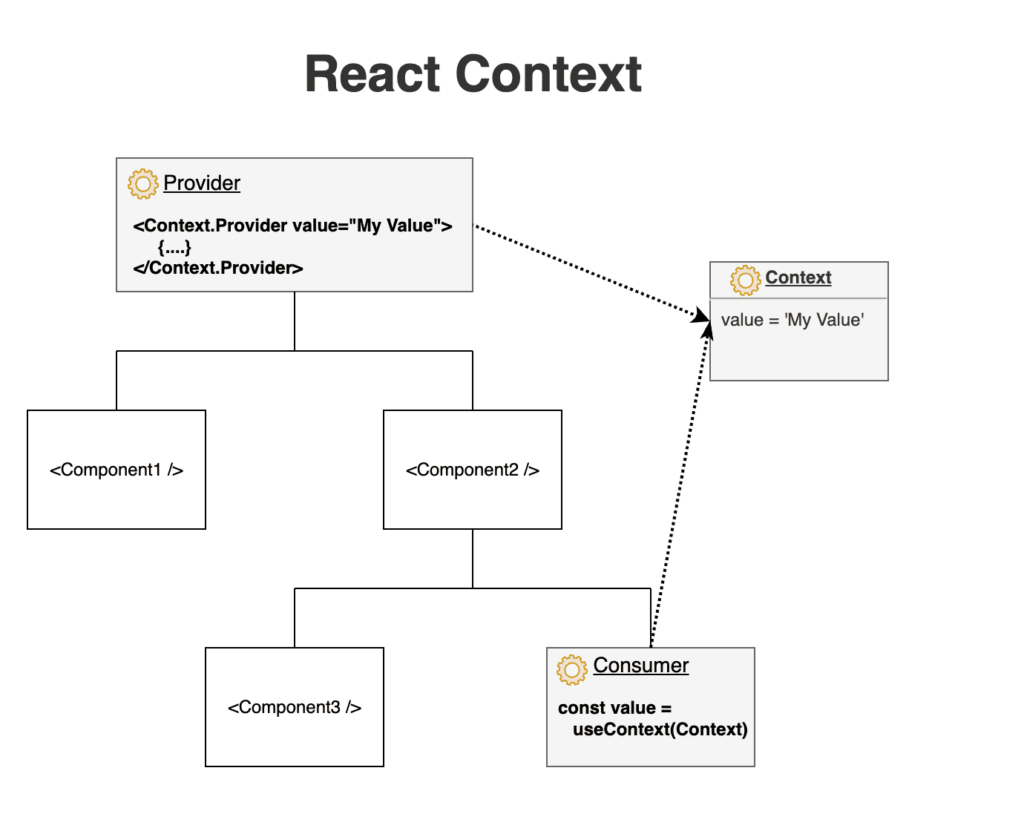
32. What are controlled vs uncontrolled components, and what are their advantages/disadvantages?
Answer:
- Controlled components: Their value is controlled by React state, ensuring predictable behavior and making them easier to manage.
- Uncontrolled components: Their value is controlled by the DOM itself, allowing for less boilerplate but less predictable behavior. They are useful for form elements with no complex logic.
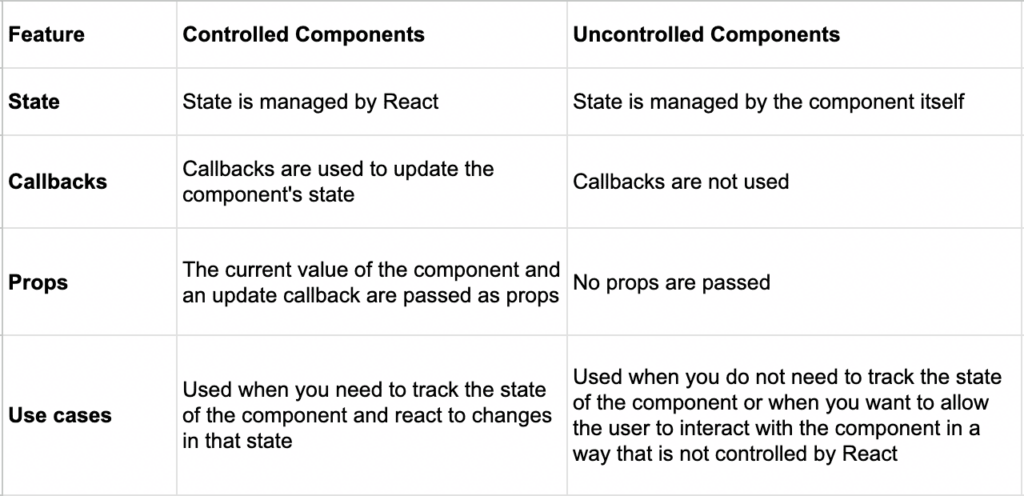
33. What is "prop drilling," and how can you avoid it in React?
Answer:
Prop drilling refers to passing props through multiple layers of components. It can be avoided by using the Context API, Redux, or other state management libraries to pass data directly to deeply nested components without passing through intermediate layers.
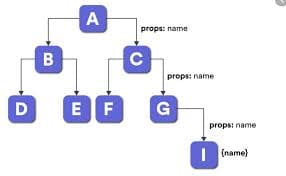
34. What is the purpose of React.memo and PureComponent? How do they help optimize performance?
Answer:
- React.memo: A higher-order component that prevents unnecessary re-renders of a functional component by memoizing the result based on props.
- PureComponent: A base class for class components that implements shouldComponentUpdate with a shallow prop and state comparison, preventing unnecessary re-renders.
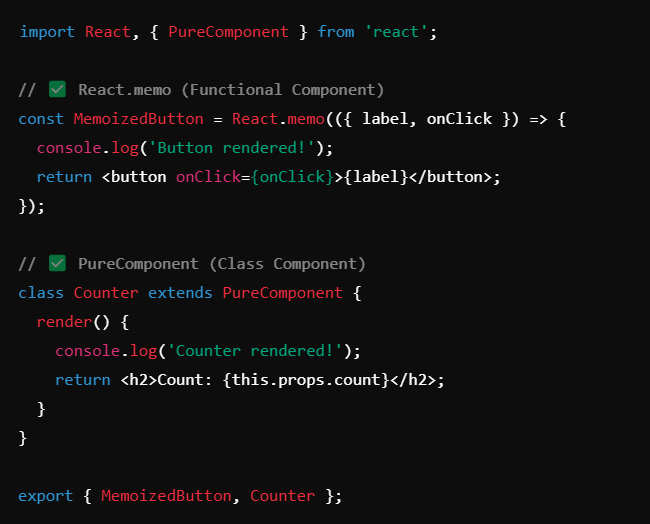
35. Explain React’s Virtual DOM and how it differs from the actual DOM.
Answer:
The virtual DOM is an in-memory representation of the real DOM. React compares the virtual DOM with the real DOM using a diffing algorithm and updates only the parts of the DOM that have changed, making updates faster and more efficient than direct manipulation of the actual DOM.
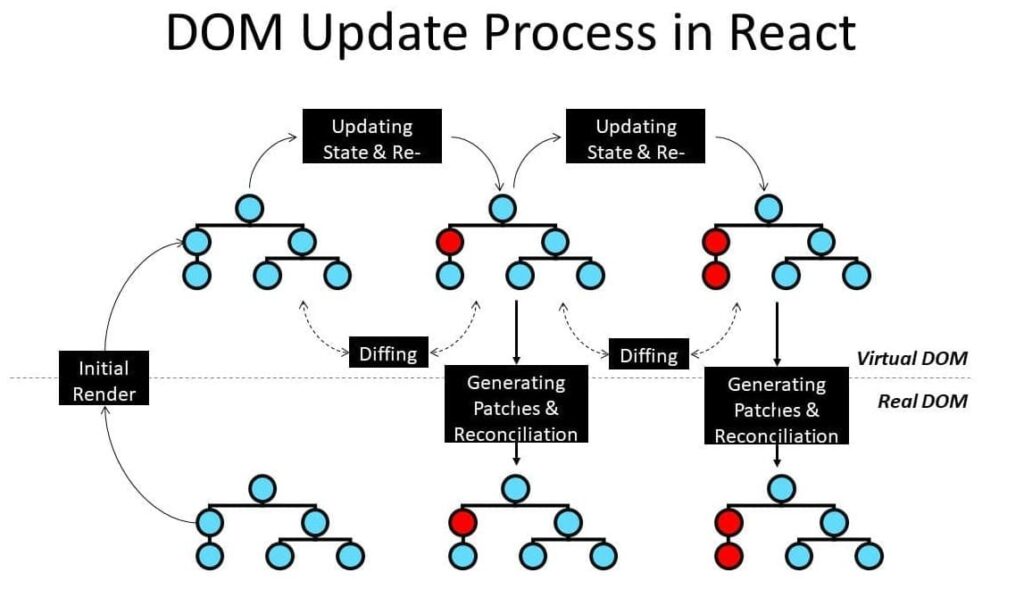
36. How does React handle component updates when using setState in class components?
Answer:
setState is asynchronous and triggers a re-render of the component. React batches multiple state updates for performance, and the new state is merged with the previous state. React ensures efficient updates through the reconciliation algorithm and the virtual DOM.
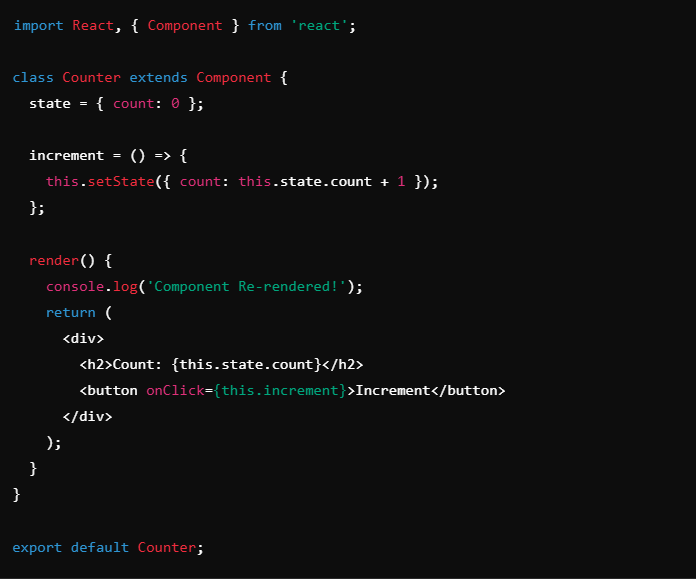
Learn From Our React js Expert Trainer
37. How do you implement code splitting in a React application?
Answer:
Code splitting can be implemented using React.lazy() and Suspense. You can dynamically import components only when needed, reducing the initial loading time of your application.
Example:
const LazyComponent = React.lazy(() => import(‘./LazyComponent’));
return (
<Suspense fallback={<div>Loading…</div>}>
<LazyComponent />
</Suspense>
);
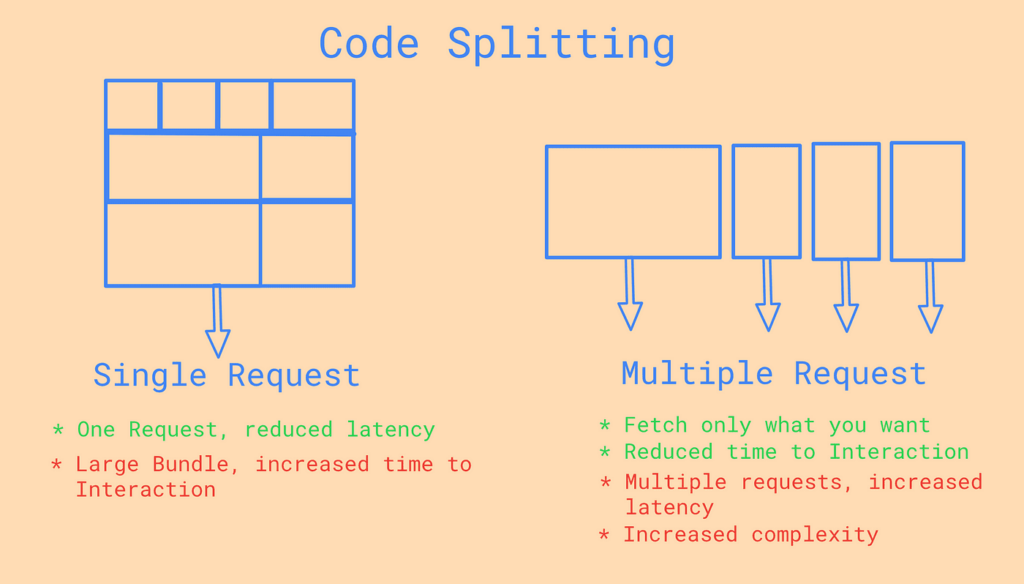
38. What is the difference between the useEffect hook and useLayoutEffect hook in React?
Answer:
- useEffect: Executes after the paint (render) process, making it ideal for side effects like fetching data, subscribing to events, and setting up timers.
- useLayoutEffect: Runs synchronously after all DOM mutations but before the browser repaints, useful for measuring DOM elements or making layout adjustments before the user sees the updates.
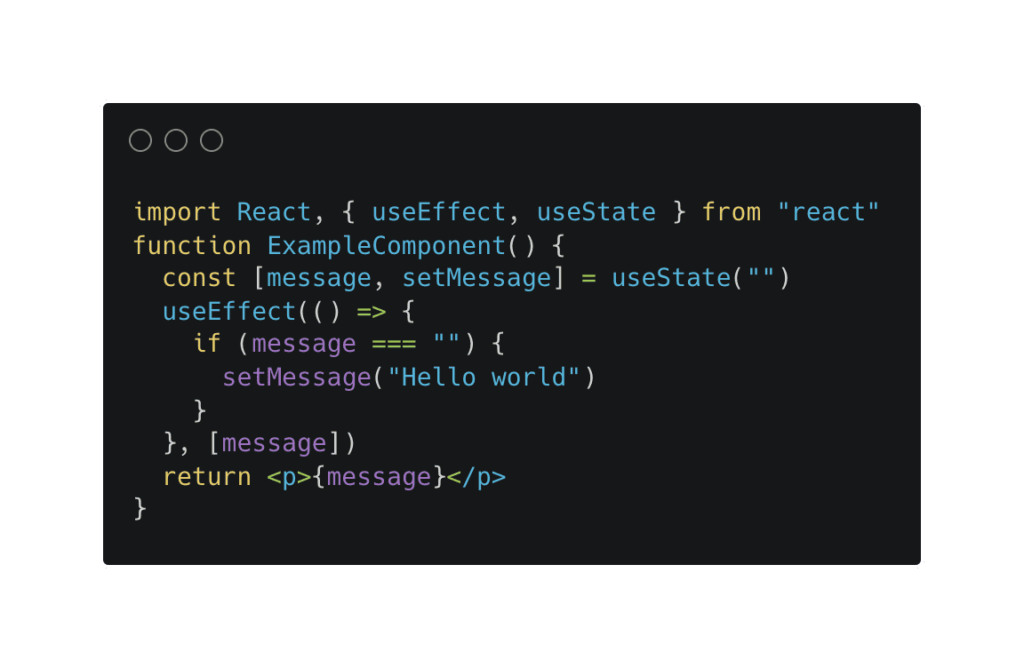
39. How does React handle asynchronous rendering with Concurrent Mode?
Answer:
Concurrent Mode enables React to interrupt rendering to work on higher-priority updates, improving user interactions by keeping the UI responsive. React can pause and resume work, allowing less critical tasks to be deferred while more important tasks take precedence.
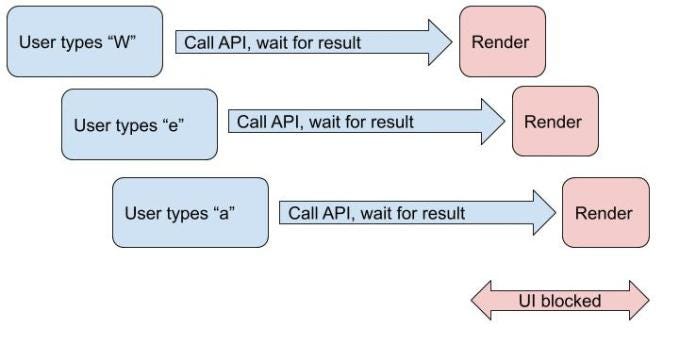
40. What are the major differences between Redux and the Context API for managing state in React?
Answer:
- Redux: A predictable state container for JavaScript apps that provides more robust features such as middleware, actions, reducers, and a central store.
- Context API: A simpler alternative to Redux that allows for sharing state across components without prop drilling. It is better suited for small-to-medium-scale state management.
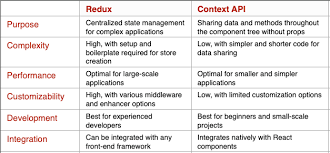
Learn From Our React js Expert Trainer
41. How do you handle side-effects in functional components using the useEffect hook?
Answer:
Side-effects like data fetching, event listeners, and subscriptions are handled by useEffect. It runs after the render and can be configured to run only when specific dependencies change, or only once on mount and unmount using an empty dependency array [].
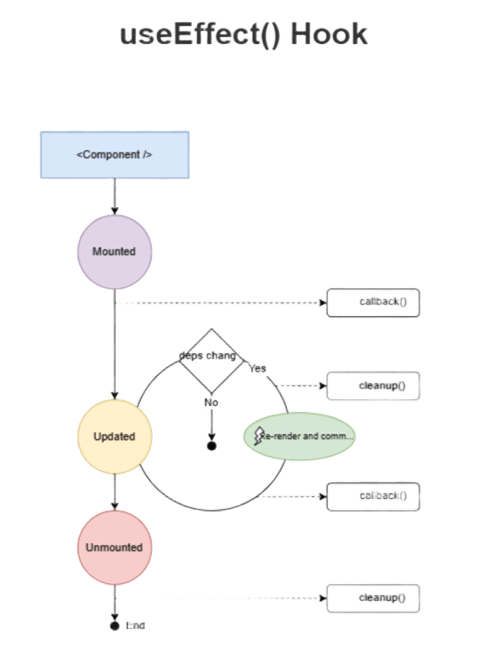
42. Explain the concept of a "Higher Order Component" (HOC) and provide an example.
Answer:
A Higher-Order Component (HOC) is a function that takes a component and returns a new component with additional functionality. HOCs allow for code reuse and separation of concerns.
Example:
function withLoadingIndicator(Component) {
return function WithLoadingIndicator({ isLoading, …props }) {
return isLoading ? <div>Loading…</div> : <Component {…props} />;
};
}
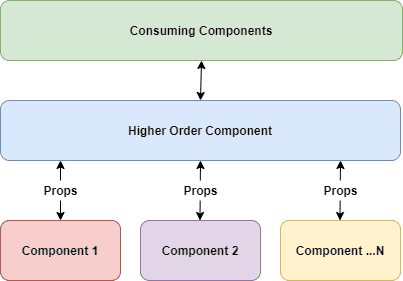
43. What is the difference between useRef and useState in React?
Answer:
- useState triggers a re-render when the state changes, making it ideal for data that affects the UI.
- useRef does not trigger a re-render when the value changes, making it useful for storing values or DOM references without affecting the UI.
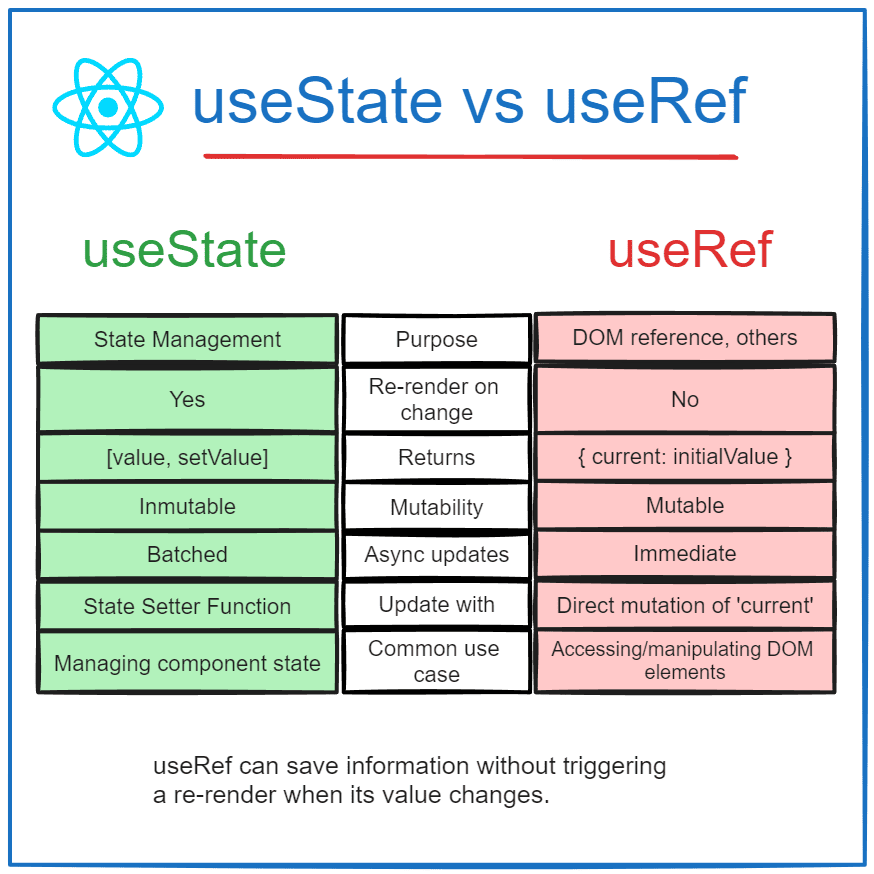
44. What are "render props" in React, and how are they different from HOCs?
Answer:
Render props is a pattern where a component takes a function as a prop, and the function returns JSX to render dynamically. Unlike HOCs, which wrap a component, render props allow a component to share logic by passing a function that renders the UI.
Example:
function MouseTracker({ render }) {
const [x, y] = useMousePosition();
return <div>{render({ x, y })}</div>;
}
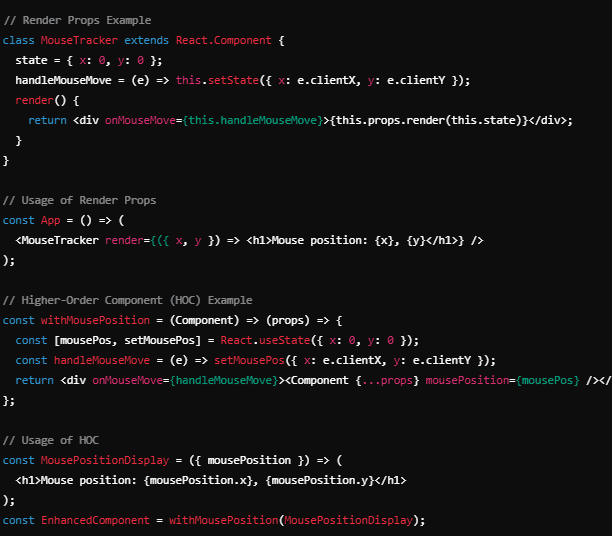
45. Explain the concept of "keyed fragments" and their use in React.
Answer:
Keyed fragments are used when rendering multiple elements without creating extra DOM nodes. React uses the key prop on fragments to track and optimize the rendering of multiple elements.
Example:
const list = [‘apple’, ‘banana’, ‘cherry’];
return (
<React.Fragment>
{list.map((item, index) => (
<React.Fragment key={index}>
<li>{item}</li>
</React.Fragment>
))}
</React.Fragment>
);
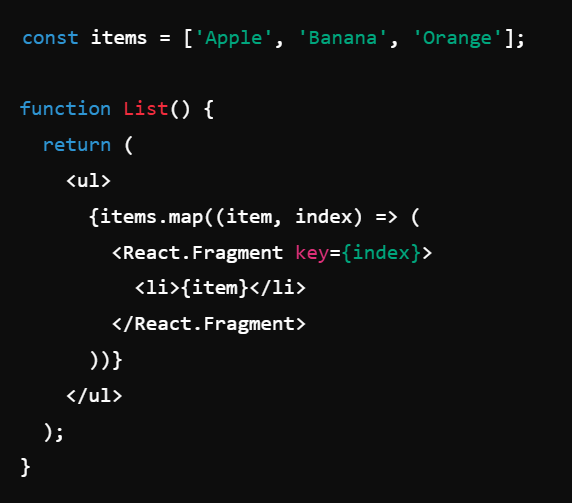
Learn From Our React js Expert Trainer
46. How do you optimize React performance in large applications with multiple updates?
Answer:
- Memoization: Use React.memo, useMemo, and useCallback to avoid unnecessary re-renders.
- Lazy loading and code splitting: Use React.lazy() and Suspense to load components only when required.
- Virtualization: Use libraries like react-window or react-virtualized to efficiently render long lists.
- Avoid Inline Functions and Objects: Inline functions and objects are recreated on each render, causing unnecessary re-renders.
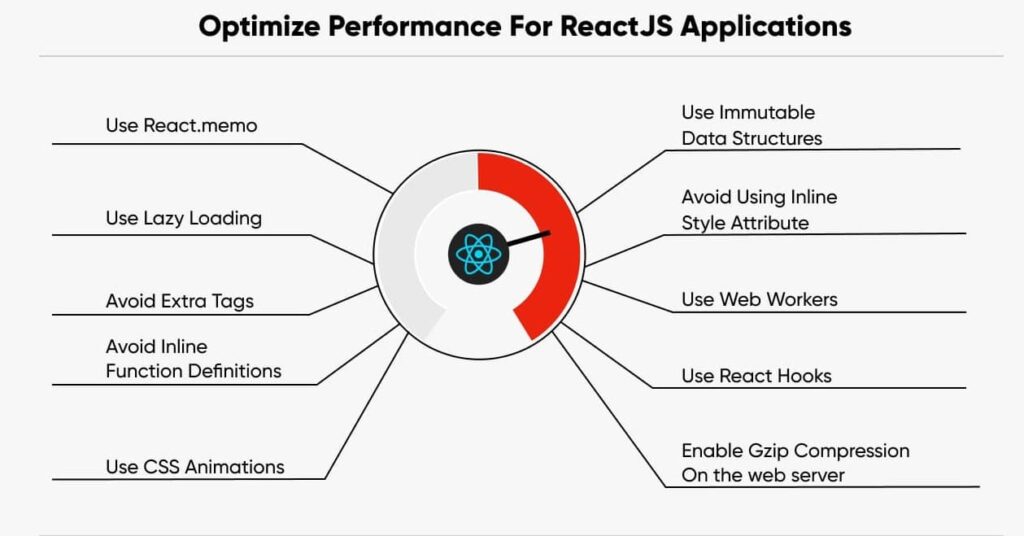
47. What are Suspense and lazy loading in React, and how do they improve performance?
Answer:
Suspense is a React feature that allows components to “wait” for something (such as data or code) before rendering. React.lazy() enables dynamic import of components, which allows the loading of components only when they are needed, reducing the initial bundle size and improving performance.
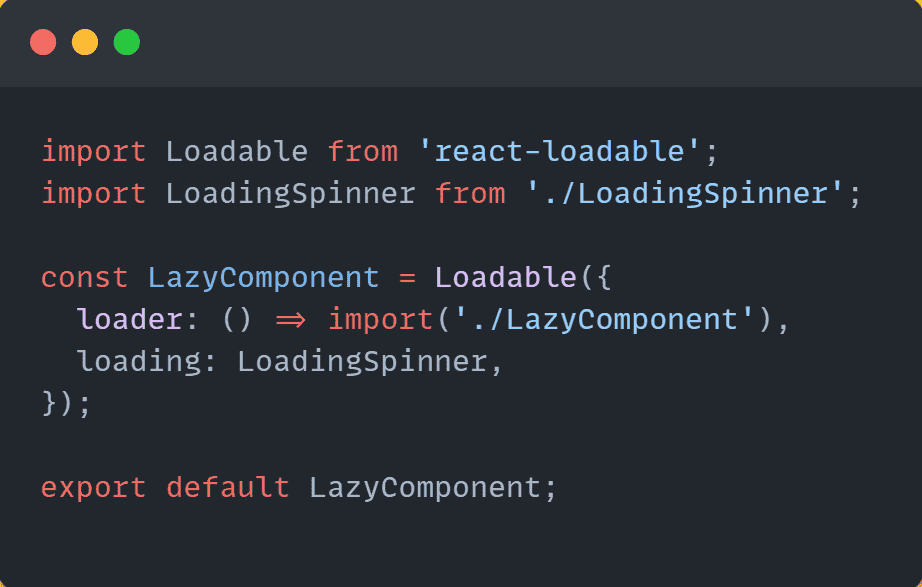
48. What are the benefits of using TypeScript with React?
Answer:
TypeScript provides strong static typing and type safety, making it easier to catch errors during development and improving the maintainability of large-scale React applications. It also provides better developer experience with autocompletion and type inference.
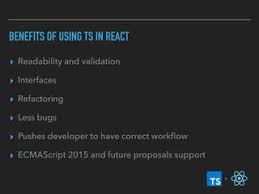
49. What are the differences between componentDidMount, componentDidUpdate, and componentWillUnmount lifecycle methods in React?
Answer:
- componentDidMount: Called once after the component is mounted. Ideal for data fetching or initialization.
- componentDidUpdate: Called after a component’s state or props change. Useful for responding to state changes or making additional updates.
- componentWillUnmount: Called before the component is unmounted. Ideal for cleanup tasks like clearing timers or removing event listeners.
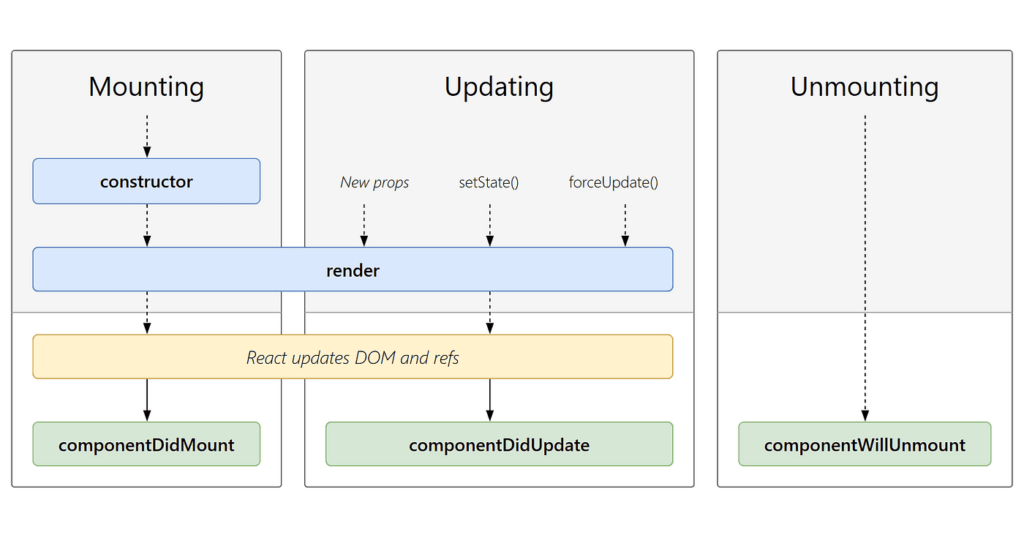
50. How do you manage large forms in React with dynamic fields or validations?
Answer:
- Use Formik or React Hook Form for managing complex form state and validations.
- For dynamic fields, manage form data with arrays or nested objects in the state.
- Leverage Yup or Joi for schema-based validation to handle complex rules.
- Use conditional rendering to show/hide fields based on other form inputs.
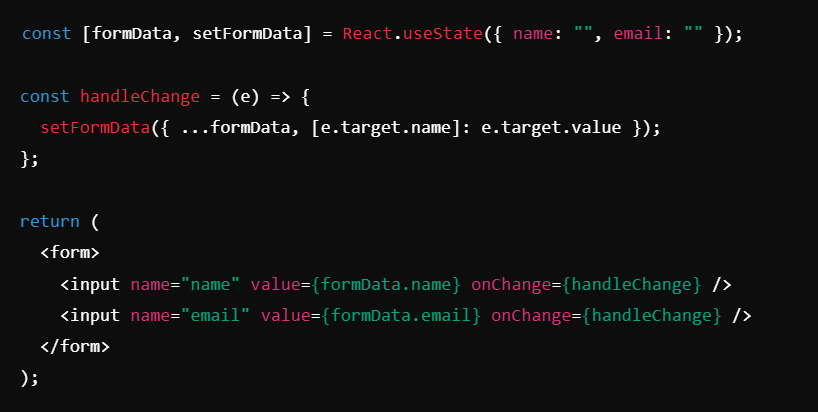