Python AI projects
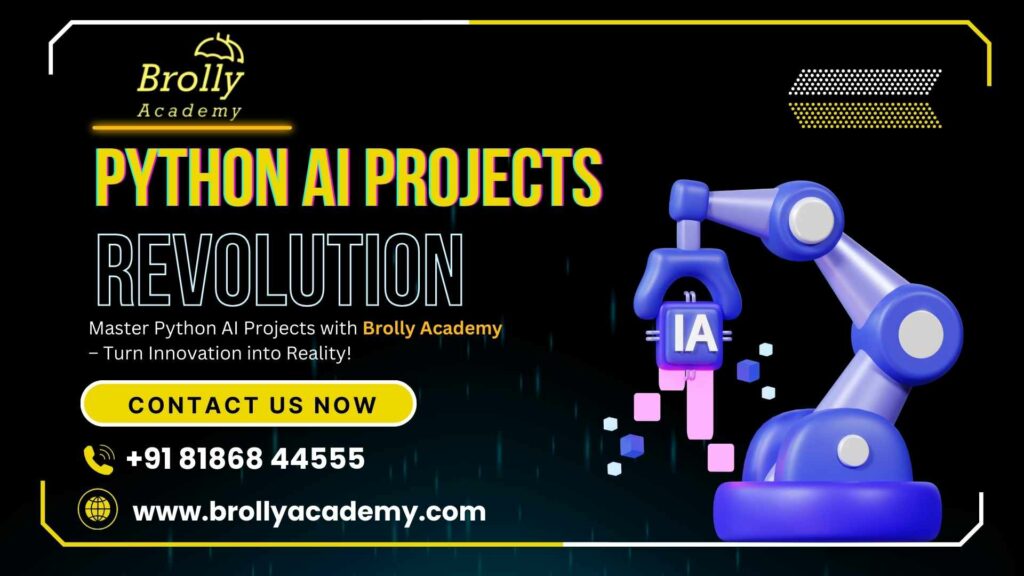
Python AI projects Beginner Level
1. Tic-Tac-Toe Game with AI
Description
Code Link
GitHub Repository: Tic-Tac-Toe with Minimax Algorithm This repository provides detailed code for setting up the game, implementing the Minimax algorithm, and running the game with an AI opponent.
Explanation
Understanding the Minimax Algorithm
- The Minimax algorithm simulates all possible moves in a game, assigning scores to each terminal state (win, loss, or draw).
- It then backtracks through the game tree, selecting the move that maximizes the player’s minimum gain (hence “Minimax”).
Implementing the Algorithm
1. Game Representation
Use a 3×3 grid to represent the Tic-Tac-Toe board.
2. Move Generation
3. Evaluation Function
Develop a function to evaluate the board’s score from the AI’s perspective:
- Positive scores for wins.
- Negative scores for losses.
- Zero for draws.
4. Minimax Function
Implement the recursive Minimax function that:
- Explores all possible moves.
- Applies the evaluation function to terminal states.
- Backtracks to determine the optimal move.
YouTube Video Link
Video: Tic-Tac-Toe with the Minimax Algorithm This video explains the fundamentals behind the Minimax algorithm and demonstrates its application in a Tic-Tac-Toe game.
2. Handwritten Digit Recognition (MNIST) Python AI projects
Description
Code Link
GitHub Repository: MNIST Handwritten Digit Classification This repository provides detailed code for setting up the neural network, training it on the MNIST dataset, and evaluating its performance.
Explanation
Implementing a Neural Network for MNIST Digit Recognition
Dataset Overview
- The MNIST dataset comprises 60,000 training images and 10,000 testing images.
- Each image is a grayscale 28×28 pixel representation of a handwritten digit.
Neural Network Architecture
- Input Layer: 784 neurons (28×28 pixels flattened).
- Hidden Layers: One or more layers with activation functions like ReLU.
- Output Layer: 10 neurons corresponding to digits 0-9, with a softmax activation function to output probabilities.
Training the Model
- Loss Function: Categorical Crossentropy.
- Optimizer: Adam optimizer.
- Metrics: Accuracy.
Evaluation
- Assess the model’s performance on the test set to determine its accuracy in classifying unseen handwritten digits.
YouTube Video Link
Video: Handwritten Digit Recognition using Neural Network This video explains the fundamentals of neural networks and demonstrates how to implement one for digit recognition using the MNIST dataset.
3. Chatbot using Natural Language Processing (NLP)
Description
Code Link
GitHub Repository: Chatbot using NLTK This repository provides code for building a simple chatbot that can respond to user inputs based on predefined patterns.
Explanation
Steps to Create a Rule-Based Chatbot
1. Define the Chatbot's Purpose
- Determine the specific tasks or questions your chatbot will handle, such as customer support or information retrieval.
2. Choose an NLP Library
- Select a Python library like NLTK (Natural Language Toolkit) or spaCy for text processing.
3. Prepare the Dataset
- Collect a set of predefined questions and corresponding answers relevant to your chatbot’s domain.
4. Preprocess the Data
- Tokenize text into words or sentences.
- Remove stop words, punctuation, and perform stemming or lemmatization.
5. Implement Intent Recognition
- Use keyword matching or pattern recognition to identify user intents.
6. Develop Response Generation
- Map recognized intents to predefined responses.
7. Test and Refine
- Evaluate the chatbot’s performance and adjust rules or patterns as needed.
YouTube Video Link
Video: Building a Rule-Based Chatbot with NLTK This video guides you through creating a rule-based chatbot using NLTK, covering data preprocessing, intent recognition, and response generation.
4. Spam Email Classifier
Description
Code Link
GitHub Repository: Naive Bayes Spam Email Classifier This repository provides code for data preprocessing, model training, and evaluation.
Explanation
Steps to Build a Spam Email Classifier
1. Dataset Preparation
- Obtain a labeled dataset containing emails categorized as spam or ham.
- A commonly used dataset is the SMS Spam Collection Dataset from the UCI Machine Learning Repository.
2. Data Preprocessing
- Text Cleaning: Remove unnecessary characters, punctuation, and convert text to lowercase.
- Tokenization: Split text into individual words or tokens.
- Stopword Removal: Eliminate common words (e.g., “the”, “is”) that do not contribute to classification.
- Vectorization: Convert text data into numerical format using techniques like TF-IDF (Term Frequency-Inverse Document Frequency) or Count Vectorization.
3. Model Training
- Use the Multinomial Naive Bayes classifier, which is effective for text classification tasks.
- Train the model on the preprocessed dataset.
4. Model Evaluation
- Assess the model’s performance using metrics such as:
- Accuracy
- Precision
- Recall
- F1-score
- Consider using cross-validation to ensure the model’s robustness.
YouTube Video Link
Video: Creating a Spam Filter using Naive Bayes This video demonstrates building a spam filter using Naive Bayes, including data preprocessing and model evaluation.
5.Movie Recommendation System
Description
Code Link
GitHub Repository: Collaborative Filtering for Movie Recommendations This repository demonstrates collaborative filtering using the MovieLens dataset to recommend movies to users.
Explanation
Steps to Build a Movie Recommendation System
1. Dataset Preparation
- Obtain Data: Use a dataset like MovieLens, which contains user ratings for movies.
- Data Structure: The dataset typically includes user IDs, movie IDs, ratings, and timestamps.
2. Data Preprocessing
- Matrix Construction: Create a user-item rating matrix where rows represent users, columns represent movies, and entries are ratings.
- Handling Missing Data: Decide on a strategy for missing ratings, such as:
- Filling with zeros.
- Using the average rating.
3. Similarity Calculation
- User-User Similarity: Compute similarity between users using metrics like:
- Cosine similarity.
- Pearson correlation.
- Item-Item Similarity: Alternatively, calculate similarity between items (movies) to recommend similar movies.
4. Recommendation Generation
- Predict Ratings: Estimate a user’s rating for unrated movies based on similar users’ ratings.
- Top-N Recommendations: For each user, recommend the top N movies with the highest predicted ratings.
5. Evaluation
- Metrics: Assess the system’s performance using:
- Mean Absolute Error (MAE).
- Root Mean Squared Error (RMSE).
- Validation: Use techniques like cross-validation to evaluate the model’s robustness.
YouTube Video Link
Video: Movie Recommendation System Using Collaborative Filtering This video guides you through building a movie recommendation system using collaborative filtering, covering data preprocessing, similarity calculation, and recommendation generation.
6. Simple Linear Regression (Python AI projects )
Description
Simple linear regression is a fundamental statistical technique used to model the relationship between an independent variable (input) and a dependent variable (output). This guide explains how to implement simple linear regression using Python.
Code Link
GitHub Repository: Simple Linear Regression Example This repository includes Python code for implementing simple linear regression, training the model on a dataset, and predicting output values based on input features.
Explanation
Key Steps in the Repository
1. Loading the Dataset
- The dataset is loaded, and the relationship between the independent and dependent variables is visualized through plots.
2. Data Preprocessing
- Prepare the dataset by cleaning and organizing it for use in the regression model.
3. Model Training
- Use the LinearRegression model from sklearn to fit the regression line to the training data.
4. Prediction
- Predict the dependent variable values based on the independent variable values in the test dataset.
5. Evaluation
- Evaluate the model’s accuracy using metrics such as:
- Mean Squared Error (MSE).
- R-squared.
YouTube Video Link
Video: Implementing Simple Linear Regression in Python This video provides a step-by-step walkthrough of building and evaluating a Simple Linear Regression model using Python and a real-world dataset.
7. Face Detection with OpenCV
Description
Code Link
GitHub Repository: Face Detection with OpenCV This repository demonstrates how to use OpenCV for detecting faces in images and videos, including real-time detection using a webcam.
Explanation
Haar Cascade Classifier
- OpenCV provides pre-trained classifiers for face detection.
- The Haar Cascade Classifier is trained to detect patterns in images that correspond to human faces using machine learning and feature detection techniques.
Steps Involved
1. Load the Classifier
- The pre-trained Haar Cascade classifier is loaded from OpenCV’s built-in data.
2. Read the Image/Video
- Functions like imread() and VideoCapture() are used to load images and video streams, respectively.
3. Grayscale Conversion
- Images are converted to grayscale to simplify the detection process, as face detection works more effectively on grayscale images.
4. Face Detection
- The detectMultiScale() function detects faces by analyzing the image for patterns associated with human faces.
5. Bounding Boxes
- Rectangles are drawn around detected faces to highlight them.
6. Real-Time Detection
- For video streams, this process runs continuously, analyzing each frame for faces and drawing bounding boxes around detected faces in real-time.
Real-Time Face Detection
- The repository includes examples of real-time face detection using a webcam feed.
- The program continuously processes video frames to detect and mark faces.
YouTube Video Link
Video: Face Detection with OpenCV `
8.Image Classification with CNN (Convolutional Neural Network)
Description
Code Link
GitHub Repository: Image Classification on CIFAR-10 using CNN This repository demonstrates how to implement image classification using a CNN to classify images from the CIFAR-10 dataset.
Explanation
Key Steps Involved
1. Loading the Dataset
- The CIFAR-10 dataset is loaded, preprocessed, and normalized to prepare it for training the CNN model.
2. Building the CNN Model
- A CNN model is created using layers such as:
- Convolutional Layers: Extract spatial features from the images.
- Max-Pooling Layers: Reduce spatial dimensions to decrease computational load.
- Dropout Layers: Prevent overfitting by randomly deactivating neurons during training.
- Fully Connected Layers: Aggregate learned features for final classification.
3. Compiling the Model
- The model is compiled with the following:
- Optimizer: Adam for efficient gradient descent.
- Loss Function: Categorical Cross-Entropy for multi-class classification.
- Evaluation Metrics: Accuracy to measure performance.
4. Training the Model
- The model is trained on the CIFAR-10 training set.
- Evaluation is performed on a validation set to monitor training progress.
5. Evaluating the Model
- The trained model is evaluated using:
- Accuracy: Measure of correct classifications.
- Loss Metrics: Quantifies model error.
- Test data is used to assess performance on unseen images.
6. Making Predictions
- The trained model can predict the classes of new, unseen images from the CIFAR-10 dataset.
Applications
- This code provides a basic framework for image classification with CNNs, which can be adapted for more complex datasets and tasks.
YouTube Video Link
Video: CNN for Image Classification using CIFAR-10 This tutorial walks through the implementation of a CNN for image classification, covering dataset preprocessing, model building, training, and evaluation.
9. Stock Price Prediction Using Linear Regression
Description
Code Link
GitHub Repository – Stock Price Prediction Using Linear Regression
Explanation
Implementing a Neural Network for MNIST Digit Recognition
1. Data Collection
- We collect historical stock data using APIs like Alpha Vantage or Yahoo Finance.
2. Data Preprocessing
- We clean the data and handle missing values to ensure accuracy in the prediction model.
3. Feature Selection
- We identify the features that are most relevant to predicting stock prices.
4. Model Training
- We use the Scikit-Learn library to train the linear regression model using the processed data.
5. Model Evaluation
- We evaluate the performance of the model by calculating metrics like Mean Squared Error (MSE) and R-squared.
YouTube Video Link
10. Number Plate Recognition System
Description
Code Link
Explanation
Steps involved in this project:
- Image Acquisition: Capture images or video frames containing vehicles.
- Preprocessing: Convert images to grayscale and apply Gaussian blur to reduce noise and enhance edge detection.
- Edge Detection: Use techniques like the Canny edge detector to highlight the edges of objects, especially the number plate.
- Contour Detection: Identify contours in the image that potentially correspond to the number plate region.
- Plate Localization: Isolate the region of interest (ROI) that contains the number plate.
- Character Segmentation: Separate the characters on the plate into individual segments for OCR.
- Optical Character Recognition (OCR): Use libraries like Tesseract to recognize and extract the text from segmented characters.
YouTube Video Link
Watch the tutorial on Number Plate Recognition with Python and OpenCV
Python AI projects Intermediate Level
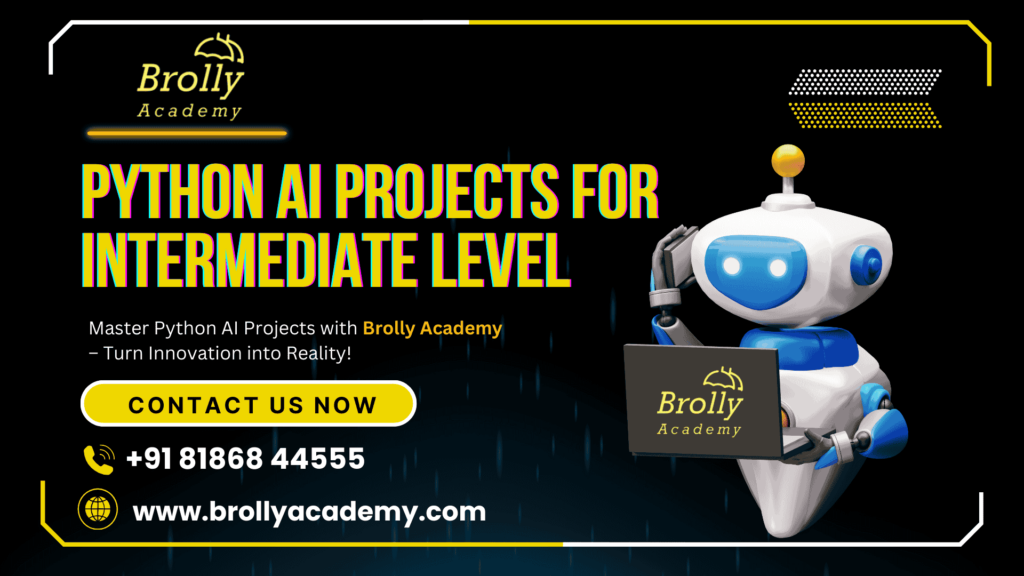
11.Voice Recognition System (Python AI projects)
Description
This project demonstrates how to create a simple voice assistant using Speech Recognition and Natural Language Processing (NLP) in Python. The assistant listens to user commands, converts speech to text, processes the text, and performs tasks based on the recognized commands.
Code Link
GitHub Repository – Voice Assistant using Speech Recognition and NLP
Explanation
1. Speech Recognition
- Use libraries like SpeechRecognition to convert audio input into text. This helps the assistant understand the user’s commands.
2. Natural Language Processing (NLP)
- Process the transcribed text using NLP techniques like tokenization, part-of-speech tagging, and named entity recognition to understand the context and intent behind the command.
3. Command Processing
- Map the user’s intent to predefined actions, such as fetching weather information or setting reminders.
4. Text-to-Speech (TTS)
- Use TTS libraries like pyttsx3 to convert the assistant’s response back into speech, providing vocal feedback to the user.
YouTube Video Link
Watch the tutorial on Building a Voice Assistant with Python
12. AI-powered Music Generator
Description
Code Link
GitHub Repository – MusicalPy: LSTM-based Music Generation
Explanation
1. Data Collection
- Gather a dataset of musical compositions in a suitable format, such as MIDI files. MIDI files encode essential musical elements like notes, rhythms, and instruments.
2. Data Preprocessing
- Convert the MIDI files into a numerical format that can be processed by the LSTM model. This typically involves extracting sequences of notes and their corresponding durations to form input data for training.
3. Model Architecture
- Design an LSTM-based neural network to learn the temporal patterns of music. The architecture typically includes:
- Embedding Layers to represent musical elements.
- LSTM Layers to capture the sequential dependencies in the music.
- Dense Layers to output the predicted next note or sequence.
4. Model Training
- Train the model on the preprocessed dataset, allowing it to learn the underlying patterns and structures of the music. This step may require significant computational resources and time.
5. Music Generation
- After training, use the model to generate new music sequences. You can provide a seed input (such as a sequence of notes), and the model will predict subsequent notes, effectively composing new pieces of music.
YouTube Video Link
Watch the tutorial on How to Generate Music using an LSTM Neural Network in Keras
13. Image Captioning
Description
Code Link
GitHub Repository – Image Captioning with CNN and LSTM
Explanation
1. Data Collection
- Use a dataset containing images and their corresponding captions, such as the Flickr8k, Flickr30k, or MS COCO dataset.
2. Data Preprocessing
- Preprocess images by resizing them and extracting features using a pre-trained CNN model (e.g., VGG16, ResNet).
- Tokenize and preprocess the captions by converting them into sequences of integers and padding them to a uniform length.
3. Model Architecture
- CNN Encoder: Extract features from images using a pre-trained CNN.
- LSTM Decoder: Generate captions using an LSTM network, which takes the image features as input and predicts the next word in the sequence iteratively.
- Combine the CNN and LSTM using a dense layer to align the feature vector with the LSTM’s input dimensions.
4. Model Training
- Train the model using the dataset of images and captions.
- Use loss functions like categorical cross-entropy and metrics like BLEU scores to evaluate performance.
5. Image Captioning
- Provide a new image to the trained model.
- Extract features using the CNN and use the LSTM to generate a sequence of words that forms the caption.
YouTube Video Link
Watch the tutorial on Building an Image Captioning Model
14. K-Means Clustering for Image Segmentation
Description
This project demonstrates how to use LSTM networks to generate music sequences based on a dataset of songs. It showcases the process of training a deep learning model to compose new musical pieces.
Code Link
GitHub Repository: MusicalPy – LSTM-based Music Generation
Explanation
The process of developing an AI-powered music generator using LSTM networks involves several key steps:
1. Data Collection
2. Data Preprocessing
- Gather a dataset of musical compositions in a suitable format, such as MIDI files, which encode musical information like notes, rhythms, and instruments.
- Convert the MIDI files into a numerical representation that can be fed into the LSTM model.
- Extract sequences of notes and their corresponding durations.
3. Model Architecture
- Design an LSTM-based neural network capable of learning the temporal patterns and structures inherent in music. The architecture typically includes:
- Embedding layers to represent musical elements.
- LSTM layers to capture sequential dependencies.
- Dense layers to output the predicted next note or sequence.
4. Model Training
- Train the model on the preprocessed dataset to learn the patterns and structures of the music.
- This step may require substantial computational resources and time.
5. Music Generation
- Use the trained model to generate new music sequences by providing a seed input and allowing the model to predict subsequent notes, effectively composing new pieces.
YouTube Video Link
How to Generate Music using a LSTM Neural Network in Keras
15.Neural Network for Image Classification (using Keras)
Description
Code Link
GitHub Repository: Image Classification with CNN on CIFAR-100
Explanation
1. Data Loading
- Normalize the pixel values of the images to a range of 0 to 1 by dividing by 255.
- One-hot encode the labels to prepare them for categorical classification.
2. Data Preprocessing
- Normalize the pixel values of the images to a range of 0 to 1 by dividing by 255.
- One-hot encode the labels to prepare them for categorical classification.
3. Model Architecture
- Construct a CNN model with the following layers:
- Convolutional Layers: Apply multiple convolutional layers with increasing filter sizes to extract hierarchical features from the images.
- Activation Functions: Use ReLU (Rectified Linear Unit) activations to introduce non-linearity.
- Pooling Layers: Incorporate max-pooling layers to reduce spatial dimensions and computational complexity.
- Flatten Layer: Flatten the 3D feature maps into 1D vectors.
- Fully Connected Layers: Add dense layers to perform classification based on the extracted features.
- Output Layer: Use a softmax activation function in the output layer to produce probability distributions over the 100 classes.
4. Model Compilation
- Compile the model using the Adam optimizer and categorical cross-entropy loss function, suitable for multi-class classification tasks.
5. Model Training
- Train the model on the training dataset, specifying the number of epochs and batch size.
- Monitor the validation accuracy to assess the model’s performance and prevent overfitting.
6. Model Evaluation
- Evaluate the trained model on the test dataset to determine its generalization capability.
YouTube Video Link
16. Stock Market Sentiment Analysis
Description
Code Link
Explanation
1. Data Collection
- Gather a dataset of news articles or social media posts related to the stock market.
2. Data Preprocessing
- Clean the text data by removing stop words, special characters, and performing tokenization.
- Apply stemming or lemmatization to reduce words to their base forms.
3. Sentiment Analysis
- Use NLP models to classify the sentiment of each article or post as positive, negative, or neutral.
- Calculate sentiment scores to quantify the overall sentiment.
4. Correlation Analysis
- Analyze the relationship between sentiment scores and stock market movements.
- Identify patterns or correlations that suggest how sentiment influences stock prices.
5. Model Development
- Develop predictive models that incorporate sentiment analysis to forecast stock market trends.
- Evaluate the models using appropriate performance metrics.
17. Movie Recommendation System (with Content-Based Filtering)
Description
Code Link
Explanation
1. Data Collection
- Obtain a dataset containing movie information, including attributes like genre, director, description, and cast.
2. Data Preprocessing
- Clean the data by handling missing values and standardizing text fields.
- Convert textual data into numerical representations using techniques like TF-IDF (Term Frequency-Inverse Document Frequency) or word embeddings.
3. Similarity Calculation
- Compute similarity scores between movies based on their attributes.
- Use cosine similarity or other distance metrics to measure how similar two movies are.
4. Recommendation Generation
- For a given movie that a user likes, identify other movies with high similarity scores.
- Recommend these similar movies to the user.
18. Customer Segmentation Using K-Means Clustering
Description
Code Link
Explanation
1. Data Collection
- Obtain a dataset containing customer information, including features such as age, annual income, and spending score.
2. Data Preprocessing
- Handle missing values and standardize the data to ensure all features contribute equally to the clustering process.
3. Applying K-Means Clustering
- Use the K-Means algorithm to partition customers into distinct clusters based on their features.
- Determine the optimal number of clusters (K) using methods like the Elbow Method or Silhouette Score.
4. Recommendation Generation
- Examine the characteristics of each cluster to understand the different customer segments.
- Visualize the clusters using dimensionality reduction techniques like PCA (Principal Component Analysis) for better interpretation.
5. Utilizing the Segments
- Develop targeted marketing strategies tailored to each customer segment.
- Enhance customer satisfaction by addressing the specific needs and preferences of each group.
19.Facial Emotion Recognition
Description
Code Link
GitHub Repository: Facial Emotion Recognition Using CNN
Explanation
1. Data Collection
- Obtain a dataset containing facial images labeled with corresponding emotions.
2. Data Preprocessing
- Resize images to a consistent size.
- Normalize pixel values to a range of 0 to 1.
- Augment the dataset to improve model generalization.
3. Model Architecture
- Construct a CNN with multiple convolutional and pooling layers to extract hierarchical features.
- Include fully connected layers to perform classification.
- Use a softmax activation function in the output layer to predict probabilities for each emotion class.
4. Model Compilation
- Compile the model using an appropriate optimizer and loss function.
5. Model Training
- Train the model on the training dataset, specifying the number of epochs and batch size.
- Monitor validation accuracy to assess performance and prevent overfitting.
5. Model Evaluation
- Evaluate the trained model on a separate test dataset to determine its generalization capability.
YouTube Video Link
20. AI for Hand Gesture Recognition
Description
Code Link
GitHub Repository: Hand Gesture Recognition Using MediaPipe
Explanation
Key Components:
- Hand Pose Estimation with MediaPipe:
- MediaPipe provides a pre-trained model for real-time hand tracking, detecting key points on the hand to understand its position and orientation.
- Gesture Recognition with MLP:
- The detected key points serve as input features for a Multi-Layer Perceptron (MLP), a type of neural network, to classify various hand gestures.
Steps to Implement:
- Set Up the Environment:
- Install the necessary libraries, including MediaPipe and TensorFlow.
- Capture Video Input:
- Use OpenCV to capture real-time video from a webcam.
- Hand Pose Detection:
- Apply MediaPipe’s hand tracking model to detect key points on the hand in each frame.
- Feature Extraction:
- Extract relevant features from the detected key points, such as distances and angles between points.
- Gesture Classification:
- Feed the extracted features into the MLP model to classify the hand gesture.
- Display Results:
- Overlay the recognized gesture label on the video feed for real-time feedback.
YouTube Video Link
Python AI projects advanced level
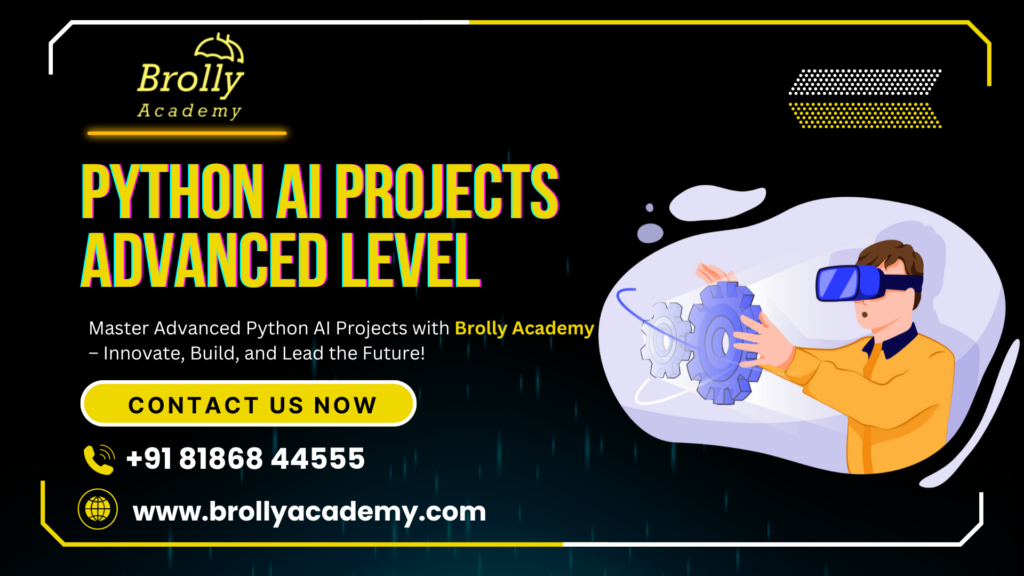
21. Deep Q-Network (DQN) for Atari Games
Description
Code Link
Explanation
Key Components:
1. Deep Q-Network (DQN):
- DQN combines Q-learning with deep neural networks to approximate the Q-value function, enabling the agent to learn optimal policies directly from raw pixel inputs.
2. Experience Replay:
- This technique stores the agent’s experiences in a replay buffer and samples mini-batches during training to break the correlation between consecutive experiences, stabilizing the learning process.
3. Target Network
- A separate target network is periodically updated with the weights of the main Q-network to provide consistent target Q-values, further enhancing training stability.
Steps to Implement:
1. Set Up the Environment
- Install the necessary libraries, including TensorFlow and OpenAI Gym, to facilitate the development and training of the DQN agent.
2. Initialize the DQN Agent
- Define the architecture of the neural network that will approximate the Q-value function.
- Set up the experience replay buffer and target network.
3. Training Loop
- For each episode, the agent interacts with the environment, stores experiences, and updates the Q-network by sampling from the replay buffer.
- Periodically update the target network to maintain training stability.
4. Evaluation
- After training, evaluate the agent’s performance on the Atari game to assess its learning effectiveness.
YouTube Video Link
22. Generative Adversarial Network (GAN) for Image Generation
Description
Code Link
Explanation
The project involves building a DCGAN, which consists of the following key components and steps:
Key Components:
1. Generator Network
- The generator network takes random noise as input and transforms it through a series of transposed convolutional layers to produce synthetic images.
2. Discriminator Network
- The discriminator network evaluates images to determine whether they are real (from the training dataset) or fake (generated by the generator).
3. Adversarial Training
- The generator and discriminator are trained simultaneously in a competitive process: the generator aims to produce realistic images to fool the discriminator, while the discriminator strives to accurately distinguish between real and fake images.
Steps to Implement:
1. Set Up the Environment
- Install the necessary libraries, including TensorFlow and Keras.
2. Prepare the Dataset
- Load and preprocess the training data, such as images from the CIFAR-10 dataset.
3. Define the Generator and Discriminator Models
- Construct the generator and discriminator networks using convolutional layers.
4. Compile the Models
- Compile the models with appropriate loss functions and optimizers.
5. Train the GAN
- Train the GAN by alternating between updating the discriminator and the generator.
6. Generate Images
- After training, use the generator to produce new images based on random noise inputs.
YouTube Video Link
23.Deep Learning for Time Series Forecasting
Description
Code Link
Explanation
Steps to Implement:
1. Data Preparation
- Preprocess the time series data by normalizing it (e.g., using MinMaxScaler) and reshaping it into sequences suitable for LSTM input.
2. Model Architecture
- Build an LSTM model with LSTM units and Dense layers for making the final prediction output.
3. Model Compilation
- Use an optimizer like Adam and a loss function like mean squared error for regression tasks.
4. Model Training
- Train the model using the training data, specifying the number of epochs and batch size.
5. Model Evaluation
- Evaluate the model’s performance on the test set and visualize the predictions alongside the actual values.
YouTube Video Link
24. Object Detection with YOLO (You Only Look Once)
Description
Code Link
GitHub Repository: YOLO Object Detection with OpenCV
Explanation
The project follows these steps for implementing real-time object detection:
Key Components
- Loading YOLO Model:
- The repository guides you through loading the YOLO model configuration and weights files.
- Processing Input:
- It demonstrates how to read images or capture video frames using OpenCV.
- Object Detection:
- The code passes input data through the YOLO network and processes the outputs to identify detected objects.
- Displaying Results:
- The detected objects are annotated on the input images or video frames and displayed in real-time.
Steps to Implement
- Set Up the Environment:
- Install the necessary libraries, such as OpenCV and NumPy.
- Download YOLO Files:
- Obtain the YOLO model configuration and weights files from the official YOLO website or repository.
- Load the Model:
- Use OpenCV’s cv2.dnn.readNet function to load the YOLO model with the configuration and weights files.
- Process Input:
- Capture images or video frames using OpenCV’s cv2.VideoCapture for video streams or cv2.imread for images.
- Perform Detection:
- Pass the input through the YOLO network and process the outputs to extract detected objects.
- Display Results:
Annotate the detected objects on the input and display the results using OpenCV’s cv2.imshow.
YouTube Video Link
25. Neural Style Transfer
Description
Code Link
GitHub Repository: Neural Style Transfer using TensorFlow
Explanation
- Preprocess Images:
- Resize and normalize the content and style images to prepare them for processing.
- Model Selection:
- Use a pre-trained CNN (e.g., VGG19) to extract feature maps at various layers.
- Extract Features:
- Use deeper layers for content representation and shallower layers for style representation.
- Compute style representations using Gram matrices.
- Define Loss Functions:
- Content Loss: Measures the difference between content features of the content and generated images.
- Style Loss: Measures the difference between style features (Gram matrices) of the style and generated images.
- Optimization:
- Use gradient descent to minimize the combined loss function (content + style loss).
- Generate Output:
- Start with the content image or random noise and optimize iteratively to create the stylized image.
YouTube Video Link
26. Speech Recognition System
Description
Code Link
Explanation
1. Data Collection
- Use datasets like Speech Commands or LibriSpeech for training and testing your model.
2. Preprocessing
- Extract features from audio files, such as MFCCs (Mel Frequency Cepstral Coefficients).
- Normalize audio lengths or pad them to ensure consistency across inputs.
3. Model Architecture
- Use Recurrent Neural Networks (RNNs) like LSTM or GRU for sequence modeling, as speech data has temporal dependencies.
- Apply Connectionist Temporal Classification (CTC) loss for alignment between the audio signal and transcribed text.
4. Training
- Train the model on GPUs to speed up computation.
- Monitor key metrics like Word Error Rate (WER) and Loss to evaluate performance during training.
5. Evaluation and Testing
- Validate the model on unseen data.
- Use benchmarks to compare your model’s accuracy and performance.
6. Deployment
- Integrate the trained model into applications for real-time or batch processing speech-to-text tasks.
YouTube Video Link
- The fundamentals of speech recognition.
- How to set up the environment and training pipeline.
- Step-by-step guidance on training and deploying the model.
27. NLP Text Summarizer
Description
Code Link
GitHub Repository: Abstractive Text Summarization with Hugging Face Transformers
Steps to Build a Text Summarizer
1. Text Summarization Types
- Extractive Summarization: Selects the most important sentences directly from the text.
- Abstractive Summarization: Generates new, concise sentences that paraphrase the input text.
2. Pre-trained Models
- Use models like T5 (Text-to-Text Transfer Transformer) or BART (Bidirectional and Auto-Regressive Transformers) for generating summaries.
3. Implementation Workflow
- Install Libraries: Use Hugging Face’s transformers and datasets libraries to work with pre-trained models.
- Load Pre-trained Model: Load a model like T5 or BART from Hugging Face’s model hub.
- Prepare Data: Preprocess the input text, which might include tokenization and formatting into sequences that the model can process.
- Generate Summaries: Use the generate method from Hugging Face’s API to generate summaries.
- Evaluate Performance: Use evaluation metrics such as ROUGE or BLEU to assess the quality of the generated summaries.
YouTube Video Link
- An explanation of the differences between extractive and abstractive summarization.
- A step-by-step code walkthrough for implementing summarization using pre-trained models like T5 and BART.
- Guidance on evaluating and deploying the summarizer in real-world applications.
28. AI Chatbot with Transformer Models (like GPT)
Description
Code Link
Chatbot using OpenAI GPT API – GitHub Repository
This repository contains complete code for implementing a chatbot that communicates with OpenAI’s GPT API to generate responses based on user inputs.
Explanation
1. Set Up the Environment
- Install necessary Python libraries like openai, requests, and flask (for web-based interfaces).
2. API Key Setup
- Obtain an OpenAI API key by registering with OpenAI and securely store it for API interaction.
3. Chatbot Logic
- Implement a function that sends user input to the GPT API and retrieves generated responses.
- Manage conversation context to allow the chatbot to maintain coherence across multiple exchanges.
4. User Interaction
- Set up a user interface either through the command line or via web technologies such as Flask or Django.
5. Enhancements
- Add additional features like personalized responses, intent recognition, or API integration for dynamic data.
YouTube Video Link
This video tutorial provides a detailed explanation of how to set up and interact with the chatbot. It covers the entire process from understanding the GPT API to running the chatbot in real-time.
29.Deep Neural Network for Sentiment Analysis
Description
Code Link
For a complete implementation of the sentiment analysis model, refer to this GitHub repository:
Deep Neural Network for Sentiment Analysis – GitHub Repository
This repository provides the full codebase for implementing sentiment analysis using an LSTM model in Keras.
Explanation
1. Data Preprocessing
- Load a labeled dataset (e.g., IMDb movie reviews) containing reviews marked as positive or negative.
- Use tokenization and padding to convert text into numerical sequences suitable for neural networks.
2. Model Architecture
- Embedding Layer: Converts words into dense vectors that capture semantic relationships.
- LSTM Layer: Captures long-range dependencies and context in text sequences.
- Dense Layer: Outputs the probability of the sentiment being positive or negative using a sigmoid activation function.
3. Training the Model
- Train the model using a binary cross-entropy loss function and accuracy as the evaluation metric to classify reviews based on sentiment.
4. Prediction
- After training, the model can predict the sentiment of new, unseen text.
YouTube Video Link
This video provides a detailed walkthrough of the entire process, covering setup, model architecture, training, and evaluation.
30. Self-Driving Car Simulation
Description
Code Link
For a complete implementation of reinforcement learning for autonomous driving using the CARLA simulator, refer to this GitHub repository:
Autonomous Driving with Deep Reinforcement Learning – GitHub Repository (Carla Simulator)
Explanation
1. Choose the Simulation Environment
- Select a platform like CARLA, Unity ML-Agents, or AirSim to create a realistic driving environment.
2. Setup and Installation
- Install the simulation environment (e.g., CARLA, Unity, or AirSim), and follow the detailed setup guides provided by the platform.
3. Reinforcement Learning Algorithm
- State Space: Define the input information such as vehicle sensors and the environment.
- Action Space: Specify possible actions like steering, accelerating, and braking.
- Reward Function: Design a reward structure to provide feedback for desired behaviors, such as staying on the road or avoiding collisions.
- Implement RL algorithms such as Deep Q-Networks (DQN) or Proximal Policy Optimization (PPO).
4. Training the Agent
- The agent interacts with the simulation, learning from its actions and adjusting its behavior over time.
5. Evaluation
- Evaluate the agent’s performance across different scenarios, such as varying weather conditions and different road types.
YouTube Video Link
31. AI-powered Facial Recognition System:
Description
Code Link
For a complete implementation of a facial recognition system, refer to this GitHub repository:
AI-powered Facial Recognition – GitHub Repository
Explanation
1. Prepare the Dataset
- Use datasets such as LFW (Labeled Faces in the Wild) or WIDER FACE, which contain labeled images of faces for training the model.
2. Face Detection
- Use algorithms like Haar Cascades or Dlib to detect faces in images or videos. OpenCV offers efficient face detection using pre-trained classifiers.
3. Face Recognition
- After detecting the face, extract facial features using deep learning models such as VGG-Face, FaceNet, or OpenFace.
- Facial embeddings (numerical representation of a face) are then compared to a database of stored embeddings for recognition.
4. Model Training (Optional for Custom Datasets)
- If you are using a custom dataset, train a CNN model or fine-tune a pre-trained model for the facial recognition task.
5. Face Verification
- Compare the generated embeddings with pre-stored embeddings of known faces to verify a person’s identity.
YouTube Video Link
32.AI Model for Predicting Protein Structure
Description
Code Link
You can refer to AlphaFold by DeepMind, which has set a benchmark for protein structure prediction:
AlphaFold – GitHub Repository
Explanation
1. Understanding the Problem
- A protein’s function is determined by its 3D structure, which is formed by the folding of the protein chain of amino acids.
- The goal is to predict this 3D shape from the primary structure (amino acid sequence).
2. Dataset
- Collect datasets such as Protein Data Bank (PDB) or UniProt, which contain protein sequences and their associated 3D structures.
3. Model Architecture
- CNNs (Convolutional Neural Networks): Used to extract spatial features and relationships in the sequence.
- GNNs (Graph Neural Networks): Used to model relationships between atoms in the protein.
- RNNs/LSTMs: Handle the sequential nature of the protein data.
- Transformers: Efficiently capture long-range dependencies in the sequences, a technique explored by AlphaFold.
4. Training the Model
- The model learns to map amino acid sequences to 3D structures by training on large datasets.
- Evaluation is based on the accuracy of the predicted structure compared to the actual structure, often using RMSD (Root Mean Square Deviation).
5. Key Techniques Used
- CNNs, GNNs, Transformers, and Reinforcement Learning (to improve folding predictions) are used to simulate protein folding and predict more accurate structures.
YouTube Video Link
For a deeper understanding of protein structure prediction using deep learning, watch this video on AlphaFold by DeepMind:
33. Generative Music with GANs
Description
Code Link
You can explore the following GitHub repository for the practical implementation of music generation using GANs:
Music Generation with GANs – GitHub Repository
Explanation
1. Prepare the Dataset
- Use datasets such as MAESTRO, JSB Chorales, or GTZAN which contain MIDI files that are ideal for music generation.
2. Preprocess the Data
- Convert MIDI files into note representations or piano roll matrices, which are compatible with neural networks.
3. Model Architecture
- The GAN model consists of two parts:
- Generator: Outputs sequences of notes (or piano rolls) mimicking real compositions.
- Discriminator: Evaluates whether the music generated by the generator is real or fake.
- Recurrent Neural Networks (RNNs) or Long Short-Term Memory (LSTM) networks are often used to capture temporal dependencies in the music.
4. Training the Model
- The generator and discriminator compete against each other to improve the quality of the generated music.
5. Generating Music
- Once trained, the generator can produce new music. You can use random inputs or specific conditions like genre or tempo to guide the generation.
6. Postprocessing
- After generating music, convert the output back into MIDI or audio formats (e.g., WAV, MP3) using tools like FluidSynth or Timidity.
YouTube Video Link
For a visual walkthrough on how to generate music using GANs, check out this YouTube tutorial:
This video provides practical demonstrations with code and music samples.
34. AI in Healthcare: Disease Diagnosis from Medical Images
Description
Code Link
You can explore the following GitHub repository for the complete implementation of medical image classification:
Medical Image Classification – GitHub Repository
Explanation
1. Dataset Collection
- Publicly available datasets like NIH Chest X-ray (for lung disease detection) or LIDC-IDRI (for lung cancer detection) are commonly used.
2. Preprocessing
- Resize the images to a uniform size (e.g., 224×224 pixels).
- Normalize pixel values and apply data augmentation techniques to improve the model’s generalization ability.
3. Model Architecture
- CNNs (Convolutional Neural Networks) are the go-to architecture for image-based tasks.
- Pre-trained models like VGG16, ResNet50, or DenseNet can be fine-tuned for medical image classification through transfer learning.
4. Training
- Use loss functions like binary cross-entropy (for binary classification) or categorical cross-entropy (for multi-class classification).
- Optimizers like Adam are typically used for efficient model training.
5. Generating Music
- Evaluate the trained model using metrics like accuracy, precision, recall, and AUC (Area Under the Curve).
6. Deployment
- Once trained, deploy the model as a real-time diagnostic tool, allowing doctors to upload new medical images for instant diagnosis.
YouTube Video Link
For a visual guide to deep learning in medical diagnosis, you can watch the following video:
35. Autonomous Drone Navigation
Description
Code Link
For a complete implementation of autonomous drone navigation using RL, check out this GitHub repository:
Autonomous Drone Navigation – GitHub Repository
Explanation
1. Define Environment
- The environment includes factors like obstacles, goals, and the drone’s states (position, velocity, etc.).
The reward function gives feedback based on the drone’s actions, such as reaching the goal or avoiding collisions.
2. RL Model Setup
- Use model-free algorithms like Q-learning or Deep Q-Networks (DQN) for discrete action spaces. For continuous action spaces, you can use algorithms like PPO (Proximal Policy Optimization) or DDPG (Deep Deterministic Policy Gradient).
3. Train the Model
- Train the drone in a simulation environment (using tools like AirSim or Gazebo) where it interacts with the environment and learns to maximize rewards through trial and error.
4. Testing & Deployment
- After the model is trained in a simulation, transfer it to a real drone for further fine-tuning.
- Implement safety measures and fail-safes to ensure the drone’s reliable operation in the real world.
5. Generating Music
- Evaluate the trained model using metrics like accuracy, precision, recall, and AUC (Area Under the Curve).
YouTube Video Link
For a step-by-step tutorial on implementing RL for autonomous drone navigation, check out this YouTube video:
36. AI for Real-time Speech Translation
Description
Code Link
For a practical implementation of real-time speech translation, check out this GitHub repository:
Real-Time Speech Translation – GitHub Repository
Explanation
1. Speech Recognition (ASR - Automatic Speech Recognition)
- Convert spoken words into text using an ASR model. Pre-trained models like DeepSpeech, Wav2Vec 2.0, or Google Speech-to-Text can be used for this task.
2. RL Model Setup
- Once the speech is converted to text, use an NMT model to translate the text from the source language to the target language. Popular models include Google Translate API, OpenNMT, or MarianMT for real-time translation.
3. Speech Synthesis (TTS - Text-to-Speech)
- After translation, convert the translated text back into speech using TTS models like Google Text-to-Speech or Tacotron to produce the translated speech in the target language.
4. Integration of All Components
- Build an application that integrates the ASR, NMT, and TTS systems to provide continuous, real-time translation. This system should handle live input through a microphone, translate the speech, and generate output speech in real time.
5. Optimization and Fine-tuning
- Fine-tune the translation and synthesis models for better accuracy and speed.
- Address challenges like accents, background noise, and latency to ensure smooth user experience.
YouTube Video Link
For a tutorial on building a real-time speech translation system, check out this YouTube video:
37. Deep Reinforcement Learning for Game Playing (like Dota 2)
Description
Code Link
For a complete implementation of DRL for Dota 2, refer to the following GitHub repository:
Dota 2 Reinforcement Learning – GitHub Repository
Explanation
1. Environment Setup
- Use APIs like OpenAI Five for Dota 2 or PySC2 for StarCraft II to interface with the game.
- OpenAI Gym or Unity ML-Agents can also be used to create and simulate game environments for training agents.
2. Agent's Objective
- The agent’s objective is to maximize its long-term reward by taking actions like defeating enemies or gathering resources. The reward function helps guide these decisions.
3. Model Architecture
- Common algorithms for game playing include DQN, PPO, and Actor-Critic models.
- These models use deep learning techniques, such as CNNs (for spatial recognition) or LSTMs (for sequential decision-making).
4. Integration of All Components
- The agent learns by playing multiple episodes, exploring the environment and improving from the feedback it receives (rewards).
- Training requires substantial computational power and can take significant time to reach optimal performance.
5. Optimization and Fine-tuning
- After training, test the agent’s performance in real game scenarios and fine-tune the model as needed.
- The agent can be deployed for use in live games or competitive play.
YouTube Video Link
38. AI for Video Summarization
Description
Code Link
Here’s a GitHub repository that demonstrates video summarization techniques:
Video Summarization – GitHub
Steps to Build Video Summarization Model
1. Data Collection and Preprocessing
- Collect a diverse dataset of videos (e.g., movie clips, YouTube videos).
- Preprocess the data by extracting important features such as:
- Frames: Extract key frames from the video.
- Audio: Use audio processing techniques (e.g., spectrograms or MFCCs).
- Text: If available, extract text from subtitles or speech-to-text.
2. Feature Extraction
- Visual Features: Use Convolutional Neural Networks (CNNs) to extract visual features from frames.
- Temporal Relationships: Use Recurrent Neural Networks (RNNs) or LSTMs to capture the relationships between frames.
- Audio Features: Extract audio features using methods like spectrograms or MFCCs.
3. Scene Detection
- Break down the video into shots or scenes.
- Use shot boundary detection to segment the video into meaningful parts.
4. Content Selection
- Supervised Learning: Train a classifier (e.g., SVM, CNN) to classify which scenes are important based on labels like “important” or “irrelevant”.
- Unsupervised Learning: Use clustering techniques (e.g., K-Means, Autoencoders) to group similar scenes and select representative ones.
5. Video Summary Generation
- Select the most relevant scenes or frames to form a coherent video summary.
- Audio: Use Text-to-Speech (TTS) to narrate the summary if necessary.
6. Video Summary Generation
- Evaluate the quality of the summary by comparing it to human-generated summaries.
- Use metrics like precision, recall, and F1-score to measure the quality of the summary.
Tools and Libraries
- OpenCV: For video frame processing.
- TensorFlow / PyTorch: For building and training the model.
- Keras: For deep learning models.
- Speech-to-Text (STT) and Text-to-Speech (TTS): For audio processing.
- FFmpeg: For video and audio preprocessing.
YouTube Video Link
For a detailed tutorial on video summarization using AI, check out this video:
39. AI in Finance: Fraud Detection System
Description
Code Link
Here’s a GitHub repository with an example of a fraud detection system:
Fraud Detection System – GitHub
Steps to Build a Fraud Detection System
1. Data Collection and Preprocessing
- Obtain transactional datasets from financial institutions or publicly available sources like Kaggle (e.g., credit card fraud detection dataset).
- Preprocess the data by:
- Handling missing values.
- Encoding categorical features.
- Normalizing numerical values to ensure consistency.
2. Feature Engineering
- Extract relevant features such as:
- Transaction amount, type, time, location, and customer’s transaction history.
- Use techniques like time-series analysis for temporal data and anomaly detection to find outliers that could indicate fraudulent activity.
3. Model Selection
- Supervised Learning:
- Use classification models like Logistic Regression, Random Forest, Gradient Boosting Machines (GBM), or Support Vector Machines (SVM).
- Unsupervised Learning:
- Use anomaly detection techniques like Isolation Forest, K-Means Clustering, or Autoencoders to detect fraud when labeled data isn’t available.
- Deep Learning:
- For detecting temporal patterns in transactions, use Long Short-Term Memory (LSTM) networks.
4. Model Training and Testing
- Split the dataset into training and testing sets.
- Train the model on historical transactional data with labels indicating fraud.
- Evaluate model performance using metrics such as accuracy, precision, recall, F1-score, and AUC-ROC curve. The F1-score is particularly important due to the imbalanced nature of fraud datasets.
5. Real-Time Prediction
- After training, deploy the model to predict fraudulent transactions in real-time.
- Integrate the model with the financial system using streaming data or batch processing to flag transactions for review or action.
6. Model Monitoring and Retraining
- Continuously monitor the model’s performance as fraudulent techniques evolve.
- Retrain the model periodically with new transactional data to maintain its accuracy.
Tools and Libraries
- Scikit-learn: For machine learning models and preprocessing.
- XGBoost / LightGBM: For gradient boosting models.
- TensorFlow / Keras: For deep learning models like LSTM.
- Pandas / NumPy: For data manipulation and preprocessing.
- Matplotlib / Seaborn: For data visualization and model evaluation.
YouTube Video Link
For a tutorial on building a fraud detection system using machine learning, check this video:
40. AI-powered Virtual Personal Assistant
Description
Code Link
Here’s a GitHub repository to help you get started on building a virtual assistant:
Virtual Personal Assistant – GitHub
Steps to Build an AI-powered Virtual Personal Assistant
1. Speech Recognition
- Convert spoken language into text using speech recognition libraries like Google Speech API or SpeechRecognition in Python.
- Process the speech input to remove background noise, normalize the audio, and segment words for further analysis.
2. Natural Language Understanding (NLU)
- Use NLP to understand the user’s intent from the speech-to-text input.
- This involves:
- Tokenization (breaking input into words).
- Part-of-speech tagging (assigning grammatical labels to words).
- Named Entity Recognition (NER) (identifying specific entities like dates, names, etc.).
- Syntactic parsing (understanding sentence structure).
- Popular NLP libraries for this include spaCy, NLTK, and Hugging Face’s Transformers.
3. Intent Recognition
- Map user input to specific actions through intent classification.
- Example: User says “Set an alarm for 8 AM,” and the intent is to “Set Alarm”.
- Use pre-trained models like BERT or Rasa NLU for intent classification and entity extraction.
4. Task Execution
- Perform tasks based on the recognized intent. These could include:
- Web search: Integrate with APIs like Google Custom Search or DuckDuckGo.
- Setting reminders/alarms: Use schedule library or system APIs for reminders.
- Controlling smart devices: Use platforms like IFTTT or Home Assistant to interface with IoT devices.
- Playing music: Connect to Spotify API, Apple Music API, or other media control APIs.
5. Response Generation
- After task execution, generate a spoken response using Text-to-Speech (TTS).
- Libraries like gTTS or pyttsx3 can convert text to speech.
- The assistant then responds audibly to the user, closing the interaction.
6. Voice Feedback and Continuous Learning
- Enhance the assistant by incorporating continuous learning through user feedback.
- Implement reinforcement learning or feedback loops to fine-tune the system and improve its accuracy over time.
- This allows the assistant to understand a broader range of commands and more complex contexts.
Tools and Libraries
- Speech Recognition: Google Speech API, SpeechRecognition.
- NLP: spaCy, NLTK, Hugging Face Transformers, Rasa NLU.
- Text-to-Speech (TTS): gTTS, pyttsx3.
- Task Execution: IFTTT, Home Assistant, Google Calendar API.
- Python Libraries: Flask (for backend), Requests (for API calls).
YouTube Video Link
Python AI projects advanced level
41. Deep Q-Learning for Game Playing
Description
GitHub Repository
Explanation
1. Deep Q-Network (DQN)
- DQN combines Q-learning with deep neural networks to approximate the Q-value function. This allows the agent to learn optimal strategies directly from the raw pixel data of Atari game screens.
2. Experience Replay
- The agent’s experiences are stored in a replay buffer. During training, mini-batches are sampled from this buffer to break correlations between consecutive experiences. This technique helps stabilize the learning process by reducing the variance in updates.
3. Target Network
- A target network is maintained separately from the main Q-network. The target network is updated periodically with the weights of the main Q-network. This improves training stability by providing consistent Q-value targets for learning.
Steps to Implement:
1. Set Up the Environment
- Install necessary libraries like TensorFlow and OpenAI Gym to create the environment and facilitate training the DQN agent.
2. Initialize the DQN Agent
- Define the architecture of the neural network to approximate the Q-value function.
- Set up the experience replay buffer and the target network.
3. Training Loop
- For each episode, the agent interacts with the environment, stores experiences, and updates the Q-network by sampling from the replay buffer.
- Periodically update the target network to maintain stable training.
4. Evaluation
- After the agent is trained, evaluate its performance on Atari games to assess its ability to learn and make optimal decisions.
YouTube Video Link
For a comprehensive walkthrough and visual demonstration of building a deep Q-learning agent for Atari games, watch this tutorial:
42. Generative Adversarial Network (GAN) for Image Generation
Description
GitHub Repository
Explanation
1. Generator Network
- The generator network receives random noise as input and processes it through transposed convolutional layers to create synthetic images that resemble the training data.
2. Discriminator Network
- The discriminator is a binary classifier that evaluates images to determine whether they are real (from the training set) or fake (generated by the generator).
3. Adversarial Training
- The generator and discriminator are trained together in a competitive process. The generator attempts to produce images that fool the discriminator, while the discriminator learns to differentiate real from fake images. This adversarial setup drives the generator to produce increasingly realistic images over time.
Steps to Implement:
1. Set Up the Environment
- Install the required libraries, such as TensorFlow and Keras, to facilitate model development.
2. Prepare the Dataset
- Load and preprocess the training data. You can use popular datasets like CIFAR-10 for image generation.
3. Define the Generator and Discriminator Models
- Build the generator and discriminator networks using convolutional layers, suitable for generating high-quality images.
4. Compile the Models
- Compile both the generator and discriminator with appropriate loss functions (e.g., binary cross-entropy) and optimizers (e.g., Adam).
5. Train the GAN
- Alternate between training the discriminator and the generator, optimizing the models until the generator produces realistic images.
6. Generate Images
- Once trained, use the generator to produce new images from random noise inputs.
YouTube Video Link
43. Deep Learning for Time Series Forecasting
Description
GitHub Repository
Explanation
1. Data Preparation
- The time series data is preprocessed, typically by normalizing the data (using tools like MinMaxScaler) and reshaping it into sequences that can be fed into an LSTM model.
2. Model Architecture
- The LSTM model is constructed with layers that include LSTM units to capture the temporal dependencies and Dense layers for making the final prediction.
3. Model Compilation
- The model is compiled using an appropriate optimizer (such as Adam) and a loss function (commonly mean squared error) suitable for regression tasks.
4. Model Training
- The model is trained on the historical data for a specified number of epochs and batch size, allowing the model to learn from the patterns in the data.
5. Model Evaluation
- After training, the model is evaluated on a separate test set. Predictions are visualized to assess how well the model forecasts future values in comparison to actual data.
YouTube Video Link
For a step-by-step guide on building an LSTM model for time series forecasting, you can watch this tutorial:
44. Object Detection with YOLO (You Only Look Once)
Description
Code link
Explanation
1. Loading YOLO Model
- The repository walks you through loading the YOLO model configuration and weights files, essential for running YOLO object detection.
2. Processing Input
- You’ll learn how to read images or capture video frames using OpenCV, a crucial step for real-time object detection.
3. Object Detection
- The core of the implementation involves passing the input through the YOLO model and processing the outputs to identify detected objects in the image or video.
4. Displaying Results
- The code shows how to annotate detected objects on the input image or video and display the results in real-time using OpenCV.
Steps to Implement
1. Set Up the Environment
- Install libraries such as OpenCV and NumPy.
2. Download YOLO Files
- Obtain the YOLO model configuration and weights files from the official YOLO website or the repository.
3. Load the Model
- Use OpenCV’s cv2.dnn.readNet function to load the YOLO model with the configuration and weights.
4. Process Input
- Capture images or video frames using OpenCV’s cv2.VideoCapture for video streams or cv2.imread for individual images.
5. Perform Detection
- Pass the input through the YOLO model and process the output to extract detected objects.
6. Display Results
- Annotate the detected objects on the image/video and display them using cv2.imshow from OpenCV.
YouTube Video Link
For a comprehensive walkthrough and visual demonstration of implementing YOLO for real-time object detection, watch this tutorial:
45. Neural Style Transfer
Description
Code link
Explanation
Key Steps in Implementing NST
1. Preprocess Images
- Resize and normalize the content and style images to fit the network’s requirements.
2. Model Selection
- Use a pre-trained CNN model, such as VGG19, to extract feature maps at different layers of the network.
3. Extract Features
- For content, use deeper layers of the CNN to extract content features.
- For style, use shallower layers and compute style representations using Gram matrices.
4. Define Loss Functions
- Content Loss: This is the difference between the content features of the content image and the generated image.
- Style Loss: This measures the difference between the style features (Gram matrices) of the style image and the generated image.
5. Optimization
- Use gradient descent to minimize the combined loss function, which is the sum of content and style loss.
6. Generate Output
- Start with the content image or random noise and iteratively optimize it to generate the final stylized image.
YouTube Video Link
46. Speech Recognition System
Description
Code link
Mozilla DeepSpeech – GitHub Repository
Explanation
Key Steps in Implementing the Speech Recognition System
1. Data Collection
- Use publicly available datasets like Speech Commands or LibriSpeech that contain large collections of speech data for training.
2. Preprocessing
- Extract relevant audio features, such as MFCCs (Mel Frequency Cepstral Coefficients), from the raw audio files.
- Normalize the audio lengths or pad them to ensure consistency across the dataset.
3. Model Architecture
- Utilize RNN-based architectures like LSTM (Long Short-Term Memory) or GRU (Gated Recurrent Units) for modeling sequential data.
- Apply Connectionist Temporal Classification (CTC) loss to align the audio sequences with the corresponding text output.
4. Training
- Train the model using GPUs for faster computation, as speech recognition models can be computationally intensive.
- Monitor metrics such as Word Error Rate (WER) and Loss during training to evaluate the model’s performance.
5. Evaluation and Testing
- Validate the model on unseen data to test how well it generalizes.
- Use benchmark datasets to compare your model’s performance and accuracy.
6. Deployment
- Once trained, integrate the model into applications for either real-time or batch processing of speech input.
YouTube Video Link
47. NLP Text Summarizer
Description
Code link
Abstractive Text Summarization with Hugging Face Transformers – GitHub Repository
Explanation
Key Steps to Build the Text Summarizer
1. Text Summarization Types
- Extractive Summarization: Selects important sentences directly from the input text.
- Abstractive Summarization: Generates new sentences that paraphrase the input, producing more fluent and human-like summaries.
2. Pre-trained Models
- Use T5 (Text-to-Text Transfer Transformer) or BART (Bidirectional and Auto-Regressive Transformers), both of which have shown exceptional performance in text generation tasks such as summarization.
3. Implementation Workflow
- Install Libraries: Install Hugging Face’s transformers and datasets libraries.
- Load Pre-trained Model: Use the T5 or BART model available on Hugging Face’s model hub.
- Prepare Data: Preprocess the input text and tokenize it into sequences for model input.
- Generate Summaries: Use the generate method of the model to generate summaries.
- Evaluate Performance: Measure summary quality using evaluation metrics like ROUGE or BLEU.
YouTube Video Link
48. AI Chatbot with Transformer Models (like GPT)
Description
Code link
Chatbot using OpenAI GPT API – GitHub Repository
Explanation
1. Set Up the Chatbot
- Connect to OpenAI’s API using your API key.
- Set up the necessary environment for processing inputs and generating responses.
2. Context-based Responses
- The chatbot is able to generate responses based on the context of the ongoing conversation, ensuring a coherent and engaging dialogue.
3. Real-time Interaction
- The chatbot can interact with users in real-time, processing and responding to queries.
YouTube Video Link
For a detailed tutorial on building your own AI chatbot with the OpenAI GPT API, check out this video:
49. Deep Neural Network for Sentiment Analysis
Description
Code link
For a complete implementation of Sentiment Analysis using an LSTM model in Keras, refer to this GitHub repository:
Deep Neural Network for Sentiment Analysis – GitHub Repository
Steps to Build the Model
1. Data Preprocessing
- Load datasets such as IMDb movie reviews, where each review is labeled as positive or negative.
- Use tokenization to convert text data into numerical representations (sequences of integers) and padding to ensure uniform input size.
2. Model Architecture
- Embedding Layer: Converts words into dense vectors, allowing the model to understand semantic relationships.
- LSTM Layer: Handles long-term dependencies in the data, which is crucial for understanding context in text.
- Dense Layer: The final layer outputs a sentiment probability (positive or negative) using sigmoid activation.
3. Training the Model
- Use binary cross-entropy loss function for classification tasks and accuracy as a performance metric.
4. Prediction
- After training, use the model to predict the sentiment of unseen text data.
YouTube Video Link
For a step-by-step guide on building the model, watch this tutorial:
Sentiment Analysis with Deep Learning (Keras) – YouTube
50. Self-Driving Car Simulation
Description
Code link
For a complete implementation of autonomous driving using Deep Reinforcement Learning with the CARLA simulator, refer to this GitHub repository:
Autonomous Driving with Deep Reinforcement Learning – GitHub Repository
Steps Involved
1. Choose the Simulation Environment
- Use a simulation platform like CARLA, Unity ML-Agents, or AirSim to create a realistic environment for the self-driving car.
- These platforms provide rich environments with realistic vehicle dynamics and traffic scenarios for training autonomous agents.
2. Setup and Installation
- Install the chosen simulation environment (e.g., CARLA, Unity, or AirSim).
- Each platform has specific setup guides for installation and configuration.
3. Reinforcement Learning Algorithm
- State Space: Define the input data like sensor readings (e.g., camera, LiDAR) or environmental factors.
- Action Space: Define the possible actions the car can take, such as steering, accelerating, or braking.
- Reward Function: Create a function that rewards the car for desirable actions, such as staying in lane or reaching a destination, and penalizes for undesirable actions (e.g., collisions).
- RL Algorithm: Implement RL algorithms like Deep Q-Networks (DQN) or Proximal Policy Optimization (PPO) to allow the agent to learn optimal driving policies.
4. Training the Agent
- The agent interacts with the environment, performs actions, and adjusts its behavior based on the rewards it receives after each step. Over time, it learns the best strategies for driving.
5. Evaluation
- Evaluate the trained agent’s performance across various driving scenarios such as different weather conditions, road types, and traffic situations.
YouTube Video Link
FAQ’s
Python AI projects
Can Python be used for AI?
Yes, Python is widely used for AI development due to its simplicity, rich libraries, and strong community support. Libraries like TensorFlow, PyTorch, and Scikit-learn make it ideal for machine learning and AI tasks.
How to make AI projects with Python?
To create AI projects with Python, start by learning the basics of Python, then dive into machine learning libraries such as TensorFlow, Keras, and Scikit-learn. Implement simple AI algorithms, then gradually work on more complex projects.
Which project is best for AI?
The best AI projects for beginners include image recognition, chatbots, recommendation systems, and sentiment analysis. As you progress, you can work on advanced projects like self-driving cars or neural networks for more challenges.
AI కోసం పైథాన్ ఉపయోగించవచ్చా?
అవును, పైథాన్ అనేది ఆర్టిఫిషియల్ ఇంటెలిజెన్స్ (AI) కోసం అత్యంత ప్రాచుర్యం పొందిన ప్రోగ్రామింగ్ భాష. దీని సులభత, లైబ్రరీలు, మరియు కమ్యూనిటీ మద్దతు వలన AI ప్రాజెక్టుల కోసం చాలా అనుకూలంగా ఉంటుంది.
Who is the father of AI?
The father of AI is John McCarthy, who coined the term “Artificial Intelligence” in 1956 and played a key role in the development of AI as a field of study.
How do I start my first AI project?
Begin by learning Python programming and AI fundamentals. Choose a beginner-friendly project like building a chatbot or a simple classifier. Use libraries like Scikit-learn for machine learning, or Keras for neural networks.
Can I create my own AI?
Yes, with the right knowledge and tools, anyone can create their own AI projects. Start small with basic AI models, and as you gain experience, you can build more complex systems.
Which language is used for AI?
Python is the most popular language for AI development, but other languages like R, Java, and C++ are also used depending on the project requirements.
Is intro to AI hard?
The introduction to AI can be challenging, but with consistent practice and proper resources, it becomes easier. Start with simple algorithms and gradually build up to more complex topics.
Does ChatGPT use Python?
Yes, ChatGPT uses Python, specifically libraries like TensorFlow and PyTorch, for training the underlying models. Python is commonly used in AI models for its versatility and rich ecosystems
Does ChatGPT use Python?
Yes, ChatGPT uses Python, specifically libraries like TensorFlow and PyTorch, for training the underlying models. Python is commonly used in AI models for its versatility and rich ecosystems
Why is Python named after a snake?
Python is not named after the reptile, but rather after the British comedy series Monty Python’s Flying Circus, which the creator, Guido van Rossum, enjoyed. The name reflects the language’s playful and easy-going philosophy.
Which Python is best for AI?
Python 3.x is recommended for AI projects, as it supports modern libraries and features. Ensure you are using the latest version of Python 3 for optimal performance.